Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial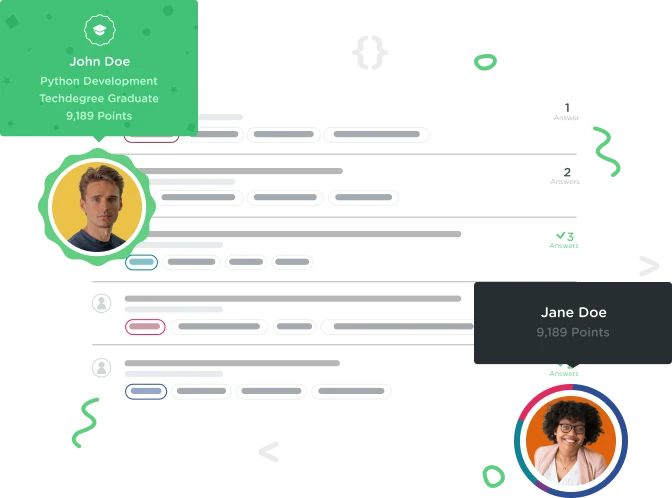

Zack Moravec
1,557 PointsCleaning Up Code
I've been browsing through the discussions and having a blast working with community suggestions and trying out new things. As I've been adding more and more to my code, I've tried to keep it as clean as possible and would love any tips on best practices to keep it clean:
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining > 0:
print("There are {} tickets remaining.".format(tickets_remaining))
try:
name = input("Welcome to MasterTicket! What's your name? ")
if "discount" in name:
raise ValueError("Sorry, there aren't any discounts at this time")
else:
ticket_request = input("Hello, {}! How many tickets would you like?\n"
"Remember, every ticket is ${}. ".format(name, TICKET_PRICE))
if "discount" in ticket_request:
raise ValueError("Sorry, there aren't any discounts at this time")
else:
ticket_request = int(ticket_request)
if ticket_request > tickets_remaining:
raise ValueError("There are only {} tickets remaining".format(tickets_remaining))
elif ticket_request <= 0:
raise ValueError("You must request at least one ticket")
except ValueError as err:
if "base 10" in str(err):
print("Sorry, that's not a valid number. Please try again.")
else:
print("{}. Please try again.".format(err))
else:
price = ticket_request * TICKET_PRICE
print("Your total is ${}.".format(price))
confirm_purchase = input("Please confirm your order.\n"
"(Enter Y/N) ")
is_confirmed = confirm_purchase.upper() == "Y" or confirm_purchase.upper() == "YES"
is_not_confirmed = confirm_purchase.upper() == "N" or confirm_purchase.upper() == "NO"
while not is_confirmed and not is_not_confirmed:
confirm_purchase = input("Please enter a valid input to confirm your purchase. ")
if confirm_purchase.upper() == "Y" or confirm_purchase.upper() == "YES":
is_confirmed = True
elif confirm_purchase.upper() == "N" or confirm_purchase.upper() == "NO":
is_not_confirmed = True
else:
if is_confirmed:
print("Sold!")
tickets_remaining -= ticket_request
else:
print("Thanks for stopping by, {}!".format(name))
else:
print("Sorry, there are no more tickets available!")
[MOD: added ```python formatting -cf]