Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial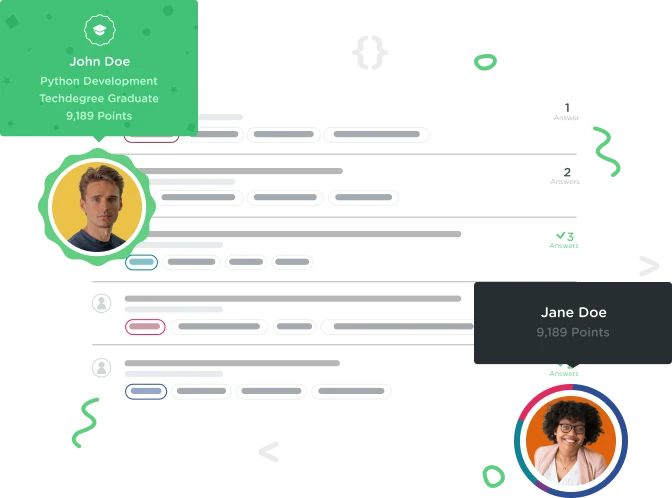

sumit garg
30 PointsClick the button and the text should copy to clipboard
How we can do so, that whenever someone clicks on the button.
That text should be copied to the clipboard.
2 Answers
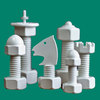
Steven Parker
230,274 PointsApparently, not all browsers support this in the same way. But I found this article that discusses various options for doing what you want.
There's also a "clipboard" package you can install with npm or just download as a .zip file.
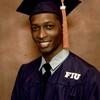
Dane Parchment
Treehouse Moderator 11,075 PointsYou can use the document.execCommand()
method to accomplish the goal that you are looking to do.
I recommend that you read through that documentation link above and then see if you can implement it yourself before checking the example I place below!
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Copying</title>
</head>
<body>
<button id="copy-button">Copy</button>
<textarea id="copy-area">Click the copy button to copy the text here and then you can paste it back or wherever you like!</textarea>
<p id="debug-text"></p>
<script src="./index.js"></script>
</body>
</html>
main.css
p#debug-text {
font-style: italic;
color: navy;
}
index.js
const copyButton = document.querySelector("#copy-button");
const copyArea = document.querySelector("#copy-area");
const debugText = document.querySelector("#debug-text");
let copiedText = "";
copyButton.addEventListener("click", (e) => {
copyArea.select();
try {
copiedText = document.execCommand("copy");
debugText.textContent = "You have successfully copied the text and can now paste it!";
}catch(err) {
debugText.textContent = "The text was not able to be succesfully copied!";
}
});
Here is the code in action on codepen! Now as Steven said, this may not work on all browsers (hence the try catch)!

Jason Anello
Courses Plus Student 94,610 PointsIt worked in firefox and chrome but it did not work with IE11.
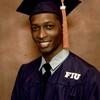
Dane Parchment
Treehouse Moderator 11,075 PointsHence why I said it won't work on all browsers, some like IE handle it differently, but I honestly do not have the time to write a program for someone for every browser out there, so I gave them a baseline to work off of, and a link to documentation, while Steven has provided them with an article with even more information.
I had also assumed that Sumit was rather new to JavaScript (seeing as he only has 30pts on TeamTreehouse) so, I was treating him under the assumption that he wouldn't know NPM or NodeJs or how to use external libraries. Unfortunately to my own knowledge execCommand is the only way I know how to do copying in JavaScript.
After re-reading my comment it seems like I was rather harsh in that response, if you felt that way I sincerely apologize.

Jason Anello
Courses Plus Student 94,610 PointsI could have been more clear in my comment but I understood that you were not making the claim that it would work in every browser.
I was simply letting sumit know which ones worked and didn't work of the ones that I tried.
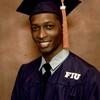
Dane Parchment
Treehouse Moderator 11,075 PointsAh, I see....out of curiosity, do you know of any way to make it work in IE?

Jason Anello
Courses Plus Student 94,610 PointsNo, I've never had a reason to do this. Maybe the sitepoint article Steven linked to has a way to do it.
Joe Beltramo
Courses Plus Student 22,191 PointsJoe Beltramo
Courses Plus Student 22,191 PointsWhat is this for? Part of a course or personal use?