Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial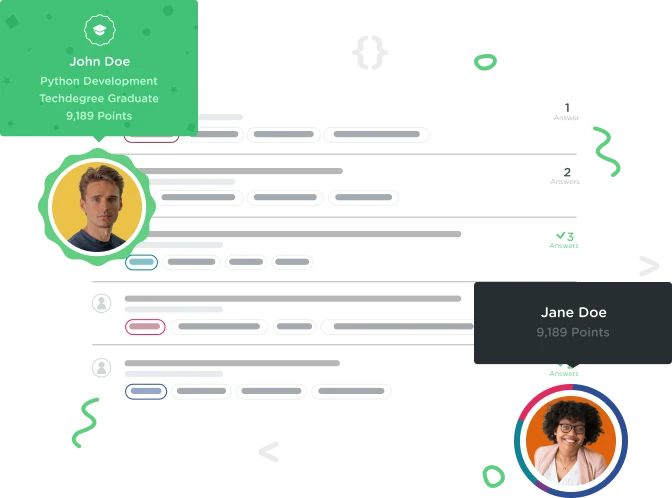

Aditya Ramabadran
3,746 Points"Closest multiple of six?"
Hi,
thanks for this video @pasan! I have a quick, slightly irrelevant question: is that the correct implementation of "closest multiple of six"?
I think your function finds the closest multiple of six to the 'value' greater than or equal to the value. For example, for 32 (your first example test), 30 is closer than 36 to it and is still a multiple of 6.
Thanks!
2 Answers

Daniel Cohen
5,785 PointsI like Ali's method, though I would use an extra set of parentheses around the OR part of the logic to make it clearer.
I did my much less elegant function like this:
func closestMultipleOfSix(value: Int) -> Int {
for x in -2...3 {
if (value + x) % 6 == 0 {
return value + x
}
}
return 0
}
Technically 0 is a multiple of all numbers so you don't need code against getting 0 as an answer.

Julian Regan
3,430 PointsThough my answer's a little longer, I think it's as simple and readable as possible:
func closestMultipleOfSix(value: Int) -> Int {
let remainder = value % 6
if remainder < 3 {
return value - remainder
} else {
return value + (6 - remainder)
}
}
Note that I'm rounding up for values that lie halfway between two multiples
Ali Salama
5,889 PointsAli Salama
5,889 PointsIf anyone is interested, here's a function that does it properly.