Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial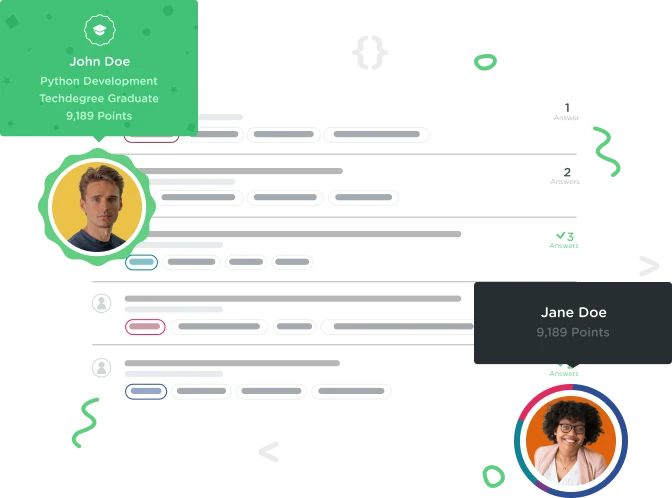

Mike Ernest
22,680 PointsCode cannot be compiled... Not sure why??
I cannot for the life of me figure this code challenge out. However, when I paste this code into an Xcode playground it seems to work fine. What am I missing?
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: String) {
self.title = title
self.author = author
self.tag = Tag(name: tag)
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOS Development", author: "Mike Ernest", tag: "swift")
let postDescription = firstPost.description()
2 Answers
Dan Lindsay
39,611 PointsHey Mike,
The challenge doesn’t ask for an init method, and yours has tag being type String, when it should be of type Tag . Also, when you created your firstPost constant, you didn’t create the proper Tag
So to pass the first part of the challenge, this will work:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
}
let firstPost = Post(title: "iOS Development", author: "Mike Ernest", tag: Tag(name: "swift"))
For the second part of the challenge, your description method is perfect, so nothing need to change there. Same for your postDescription constant. So this code will pass:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOS Development", author: "Mike Ernest", tag: Tag(name: "swift"))
let postDescription = firstPost.description()
While you don’t need the init method to pass the challenge, this one added to Post will still pass the challenge:
struct Post {
var title: String
var author: String
var tag: Tag
init(title: String, author: String, tag: Tag) {
self.title = title
self.author = author
self.tag = tag
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
Hope this helps!
Dan
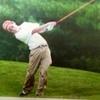
kjvswift93
13,515 PointsThe issue here is that the challenge doesn't ask you to create a custom initializer method.
struct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let myTag = Tag(name: "Classics")
let firstPost = Post(title: "1984", author: "George Orwell", tag: myTag)
let postDescription = firstPost.description()

Mike Ernest
22,680 PointsThanks, Kyle! Very helpful.
Mike Ernest
22,680 PointsMike Ernest
22,680 PointsThanks so much for taking the time to explain this. Really helpful and I understand where I went wrong.