Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial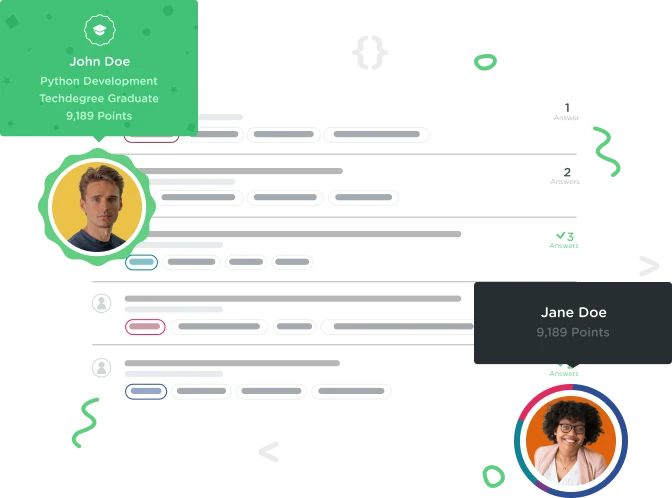

Enemuo Felix
1,895 PointsCode challenge
The code below logs all of the even numbers from 2 to 24 to the JavaScript console. However, there's a lot of redundant code here. Re-write this using a loop.
PLS HOW DO I GO ABOUT IT?
console.log(2);
console.log(4);
console.log(6);
console.log(8);
console.log(10);
console.log(12);
console.log(14);
console.log(16);
console.log(18);
console.log(20);
console.log(22);
console.log(24);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
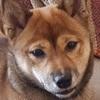
Katie Wood
19,141 PointsHi Enemuo,
You'd start with the basic 'for' loop structure. We know that the numbers we print need to be between 2 and 24, and that we only want the evens. Since a 'for' loop declaration includes a loop variable, how long to loop, and how the variable changes each time, we can say:
- the variable should start at 2
- the variable should end at 24
- the variable should go up by 2 each time
- the variable should be logged to the console each time
Going from this, we can create the basic loop structure:
for (var i = 2; i <= 24; i += 2) { //i starts at 2, stops after 24, goes up by 2 each time
}
We've done most of the work at this point. Now, you just need to add:
console.log(i); //print the number out
inside the loop. Good luck!
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsThank you very much Katie, tbh this idea really came to my head but It switched to confusion in a split second. Look at me getting all confused at this stage. smh
Katie Wood
19,141 PointsKatie Wood
19,141 PointsDon't worry about it - it can take some time and practice for 'for' loops to really click. If it popped into your head, that means you can recognize when you might want a 'for' loop - that's great. From here, it's just about practice.
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsThanks, Hopefully. Can you recommend any Tool i can use to practice Js? and secondly do you know how i can enter another line when writing with the console,since the enter key automatically runs the code?
Katie Wood
19,141 PointsKatie Wood
19,141 PointsFor me, the best practice for syntax and general logic structures is to try to recreate card or dice games. If you generally use Workspaces to follow along, you can use one of your class projects as a starting point by finding it in your Workspaces page (the link is in the upper right by your profile), and clicking the "Fork Workspace" button next to it (looks like some branching lines). This will give you a new copy of that workspace that you can mess with without messing up the other project.
For writing multiple lines in a console, you need a REPL (read-eval-print loop). I'm not sure off the top of my head if Workspaces offers a JavaScript repl, but luckily the browser's console does. You can even pull it up on this page - in Chrome it's ctrl-shift-J and in Firefox it's ctrl-shift-K (a quick Google would give you the keys for any other browser you might be using). In that console, you can write out things like functions, and the enter key won't exit the function until you've closed it with a closing curly brace ( } ). In the browser, you can create variables and functions, and call those functions, so it can be a good way to test small code snippets to see how they would behave in the browser. Things like console.log() and prompt() will work from there.
Good luck!
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsBy practice i mean writing a code and maybe a few days then i can come back , edit or continue where i left off . I can't quite get your answer to my second question. Do you mean to avoid putting the curly bracket in my code when writing in the console until i have written other part of the code? I mean entering another paragraph in the console to continue writing(since the enter key runs the code even when you are not through)
Katie Wood
19,141 PointsKatie Wood
19,141 PointsFor writing some code, leaving, then coming back, you could use Workspaces, as I mentioned. You could also set something up on your own computer - maybe download the files for one of your courses to have a starting point - you'd just need a text editor, like Atom or Sublime (there are many others too).
Sorry if the second part was confusing - I meant that the browser console would allow you to write multiple lines to create something like a function that uses multiple lines. For example, if you type "function myFunction () {" and then hit enter, it will let you enter your other lines and hit enter without submitting anything until you put in the "}" to close the function.
By definition, the console isn't going to let you type out more than one line at once - if you need that, you'll have to do it in a script file. In the console, you can do more than one thing at once by typing something like "let varOne = 1; let varTwo = 2;" on the same line, but you can't hit Enter in between if you want them in the same command. It's worth noting, though, that you don't need them to be together - as long as you have the console open, it will save those variables and you can use them in later commands.
Does that answer your question?
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsThank you katie for saving me
Katie Wood
19,141 PointsKatie Wood
19,141 PointsHappy to help!