Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial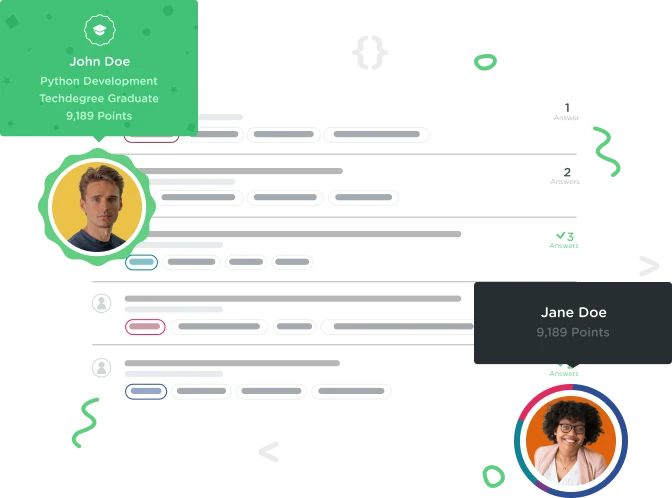
Tyrone Taylor
Courses Plus Student 11,792 Pointscode challenge
can someone tell me what is wrong
spareWords.unshift("firstWord"); spareWords.shift();
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Arrays</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Arrays: Methods Part 1</h2>
<script>
var spareWords = ["The","chimney","sweep's","dog"];
var saying = ["quick", "brown", "fox", "jumps", "over", "the", "lazy"];
var firstWord = spareWords;
var lastWord = spareWords;
saying;
saying;
</script>
</body>
</html>
2 Answers
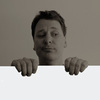
Sean T. Unwin
28,690 PointsI assume you got the first part of the challenge correct with (on line 18):
var firstWord = spareWords.shift();
The second part of the challenge wants you to assign the variable lastWord
(on line 19) with the last item in the spareWords
array while also removing that last item from the spareWords
array. To do this you need to use pop()
.
If you are having trouble try watching the video again while doing the excerices that Jim is doing in your browser's DevTools.
Please continue to ask questions that you may have. :)

LaVaughn Haynes
12,397 PointsI'm not sure what you are trying to do but if you are having trouble with using shift and unshift, this is how they work:
// your array
var spareWords = ["The","chimney","sweep's","dog"];
// shift() REMOVES the first item in the array AND RETURNS IT
// in this case the item is returned to the variable theFirstSpareWord
var theFirstSpareWord = spareWords.shift(); // var theFirstSpareWord = "The";
// outputs: The
console.log( theFirstSpareWord );
// outputs: ["chimney", "sweep's", "dog"]
console.log( spareWords );
// unshift ADDS a value to the FRONT of an array
// in this case we prepend "Hello" to the array
spareWords.unshift( "Hello" );
// outputs: ["Hello", "chimney", "sweep's", "dog"]
console.log( spareWords );