Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial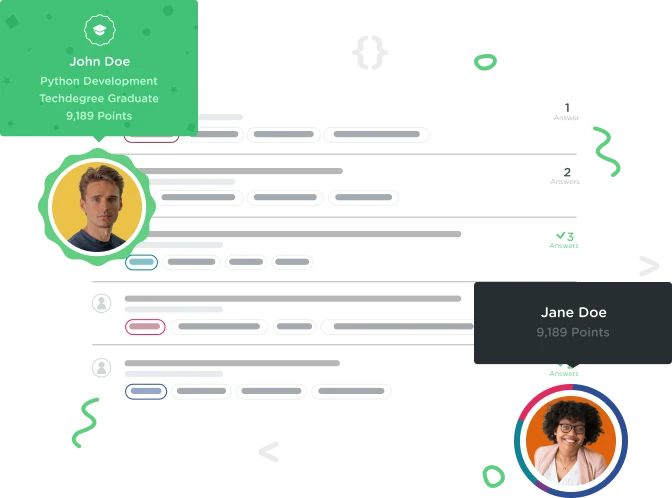

Hussein Amr
2,461 Pointscode challenge
Alright, this one might be a bit challenging but you've been doing great so far, so I'm sure you can manage it. I need you to make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string. Check the comments below for an example.
i'm stuck..
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(**args):
string = ("I am clever am I not").lower()
for words in string:

Hussein Amr
2,461 PointsI kind of figured it out but sadly it's still not passing
def word_count():
count = dict()
sentence = ("I am clever am I not").lower()
words = sentence.split()
for word in words:
count[word] = count.get(word, 0) + 1
print(count)
word_count()
2 Answers
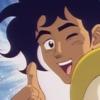
Kazuma Namioka
20,674 PointsYou're getting there. Some small things to change are making the function return the dictionary rather than print it out, as otherwise the challenge won't count as being completed. For your last line in the function:
return count
and you don't need to call the function in the challenge itself, so the last
word_count()
is unnecessary.
The string should be an argument, for this challenge you don't need to specify what the string is.
def word_count(string):
words = string.lower().split()
The main thing is the line:
count[word] = count.get(word, 0) + 1
Here, you need something more like:
count[word] += 1
but this only works for words that have already been added to the count dictionary, so you need something to add the word to the dictionary if it isn't in there yet.
Spoiler for how I solved the challenge below.
def word_count(string):
count = dict()
words = string.lower().split()
for word in words:
try:
count[word] += 1
except KeyError:
count.update({ word : 1 })
return count

Hussein Amr
2,461 Pointsin your solution, what does count.update({word : 1}) exactly do
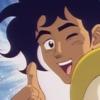
Kazuma Namioka
20,674 PointsThe count dict here starts empty. The for loop has to either add the word to the dictionary if it was the first time this specific word was encountered, or increment the count of that specific word by 1 if the word was already added.
The count.update line is for when the word is not yet in the dictionary. The first time the for loop encounters the word "am", it will try to increase the value of the key "am" by 1, and when that doesn't work (it's the first time encountering the word "am", so the key does not yet exist in the dict) it updates the dict by adding the key "am" and giving it a value of 1 (because it's the first time the word has shown up).
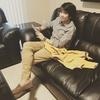
macmondiaz
1,415 PointsQuestion:
Why does my code not passing the challenge? when I run my code on workspace, it does work just like Kazuma's code... HALP!
def word_count(string):
dictionary = {}
sentence = string.lower().split()
count = 1
for word in sentence:
if word in dictionary:
dictionary.update({word:count+1})
else:
dictionary.update({word:count})
return dictionary

Thomas Ross
4,634 PointsHi, I ran a few tests and found this:
print(word_count("AM AM AM WITH WITH TOM TOM TOM TOM HI"))
Should return something like:
{'hi': 1, 'am': 3, 'with': 2, 'tom': 4}
4 tom, 3 am, 2 with, 1 hi
Your function returned:
{'tom': 2, 'with': 2, 'hi': 1, 'am': 2}
I think the problem is that the count is never updated, possibly? Good luck! I'm having trouble with this one, maybe have a look at mine when you solve yours!

Mathew V L
2,910 PointsNot sure why this doesn't work
spits out what is requested but getting I need to lowercase and split in white spaces
def word_count(string):
dictionary = {}
string = string.lower()
string = string.split()
count = 1
for word in string:
if word in dictionary:
dictionary.update({word:count+1})
else:
dictionary.update({word:count})
return(dictionary)
Kazuma Namioka
20,674 PointsKazuma Namioka
20,674 PointsIt seems a shame to just tell you how to pass the challenge (also, I don't want anyone to see my ugly solution) so how about some hints:
list_of_words = "I am clever am I not".lower().split()
splits the string at every space character and gives you a list of the individual words in the string. Handy! I bet you have ideas about how to solve this already. Right now the
for words in string:
bit of code considers individual letters in the string, including the space characters, as words.
I personally didn't use *args to solve this. I'm also pretty sure it's convention to use the double-asterisk ** with **kwargs and single asterisk with *args. Good luck!