Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial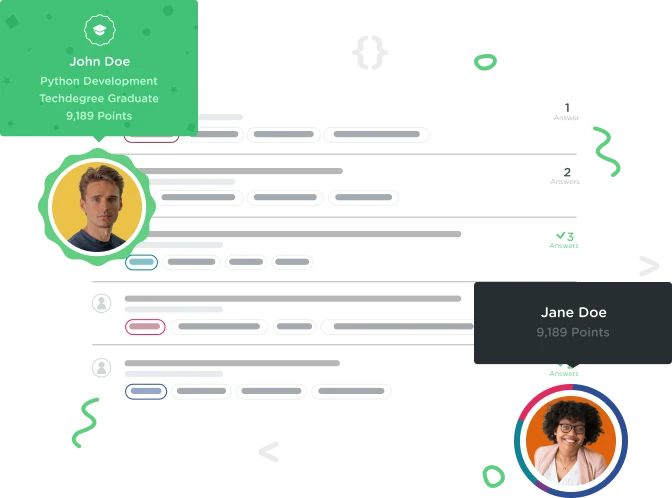

Brian Chewning
7,270 Pointscode challenge: Adding Secondary Data with a SimpleAdapter 3 of 4
I cannot figure out what I am doing wrong on this, the challenge is "Now create a SimpleAdapter variable. It's constructor has 5 parameters. 1. The context (use 'this'). 2. The array to be adapted. 3. The layout for each item (use 'android.R.layout.simple_list_item_2'). 4. An array of keys needed to map values (use the 'keys' array). 5. An array of int IDs where the values are placed (use the 'ids' array)." Here is my code...
import android.os.Bundle;
import android.widget.SimpleAdapter;
public class WebsiteListActivity extends ListActivity {
public static final String KEY_NAME = "name";
public static final String KEY_URL = "url";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_website_list);
String[] keys = { KEY_NAME, KEY_URL };
int[] ids = { android.R.id.text1, android.R.id.text2 };
SimpleAdapter = new SimpleAdapter(this, websites, android.R.layout.simple_list_item_2, keys, ids);
// Add code here!
HashMap<String, String> name = new HashMap<String, String>();
name.put(KEY_NAME, "one");
name.put(KEY_URL, "two");
HashMap<String, String> url = new HashMap<String, String>();
url.put(KEY_NAME, "three");
url.put(KEY_URL, "four");
ArrayList<HashMap<String, String>> websites = new ArrayList<HashMap<String, String>> ();
websites.add(name); websites.add(url);
}
}
3 Answers

Ben Jakuben
Treehouse TeacherTwo things:
- Your line for the SimpleAdapter doesn't set a name for the variable.
- You are defining the SimpleAdapter (using
websites
) before you actually declare thewebsites
variable.
Move your SimpleAdapter line and fix it and you'll be all set!
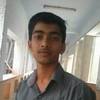
Ajay Maheshwari
Courses Plus Student 6,423 PointsUse this
import android.os.Bundle; import android.widget.SimpleAdapter;
public class WebsiteListActivity extends ListActivity {
public static final String KEY_NAME = "name"; public static final String KEY_URL = "url";
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_website_list);
String[] keys = { KEY_NAME, KEY_URL };
int[] ids = { android.R.id.text1, android.R.id.text2 };
ArrayList<HashMap<String, String>> websites = new ArrayList<HashMap<String, String>> (); SimpleAdapter adapter = new SimpleAdapter(this, websites,android.R.layout.simple_list_item_2, keys, ids); // Add code here! HashMap<String, String> name = new HashMap<String, String>(); name.put(KEY_NAME, "one"); name.put(KEY_URL, "two"); HashMap<String, String> url = new HashMap<String, String>(); url.put(KEY_NAME, "three"); url.put(KEY_URL, "four");
websites.add(name); websites.add(url);
}
}

james white
78,399 PointsThis is what I got to pass the Adding Secondary Data with a SimpleAdapter 3 of 4 code challenge
(Just so there is some properly forum marked up code in this post):
import android.os.Bundle;
import android.widget.SimpleAdapter;
public class WebsiteListActivity extends ListActivity {
public static final String KEY_NAME = "name";
public static final String KEY_URL = "url";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_website_list);
String[] keys = { KEY_NAME, KEY_URL };
int[] ids = { android.R.id.text1, android.R.id.text2 };
// Add code here!
HashMap<String, String> name = new HashMap<String, String>();
name.put(KEY_NAME, "one");
name.put(KEY_URL, "two");
HashMap<String, String> url = new HashMap<String, String>();
url.put(KEY_NAME, "three");
url.put(KEY_URL, "four");
ArrayList<HashMap<String, String>> websites = new ArrayList<HashMap<String, String>> ();
websites.add(name); websites.add(url);
SimpleAdapter adapter = new SimpleAdapter(this, websites, android.R.layout.simple_list_item_2, keys, ids);
}
}
..and I think to pass the final stage of the challenge you just have to add one line after the SimpleAdapter line:
setListAdapter(adapter);
Now I can finally move on to "Implementing Designs for Android": http://teamtreehouse.com/library/implementing-designs-for-android (for which the Blogger App was a pre-requisite..)
Brian Chewning
7,270 PointsBrian Chewning
7,270 PointsI got it. Thanks for the help.