Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial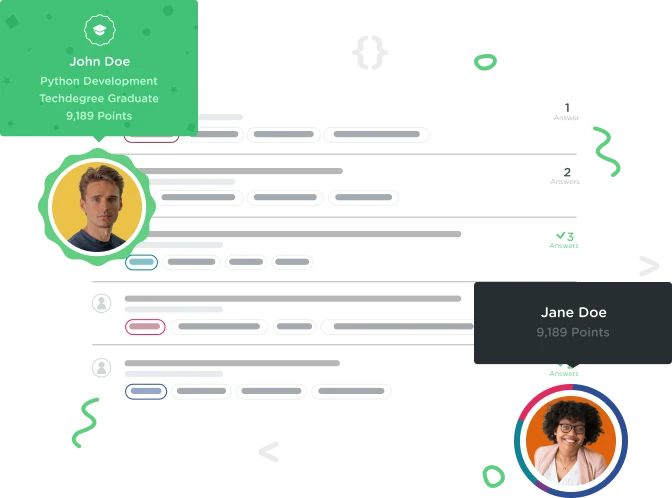
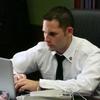
Kyle Brooks
6,753 PointsCode Challenge: Arrays - Methods Part 2 - Question 2 of 2
Task: Sort the 'saying2' array, on about line 19, so that the words are listed in length order. The shortest first. Use the 'length' property on the strings in the sort function.
Link: http://teamtreehouse.com/library/websites/javascript-foundations/arrays/methods-part-2-4
The array that I typed was...
<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort (function () {
return length });
</script>
16 Answers

Mike Bronner
16,395 PointsOK, it looks like you probably should watch the video again and see exactly how they do it there. I would recommend studying the following concepts a bit more before continuing:
- functions
- conditional statements (if else, etc.)
Otherwise you will find that you will be banging your head against the wall at every step. One thing you could also do is open the video immediately previous to this challenge in a separate window, and run it side-by-side with the challenge, pausing the video as you complete the challenge. That way you are doing as he is explaining, and things may become clearer to you.
Here is what they are looking for in the challenge:
saying2.sort(function(a, b)
{
if (a.length > b.length) {return 1;} //return 1 if a is longer than b
else if (a.length == b.length) {return 0;} //otherwise return 0 if they are the same length
return -1; //if this is reached, return -1, as by default a must be shorter than b
});
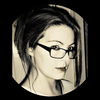
Lauren Clark
33,155 PointsThis seems a bit complex! You just need to do the same as Jim does with the function, but refer to the length of a and b.
saying2.sort(function (a, b){return a.length - b.length});
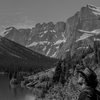
Travis Stewart
15,188 PointsThanks, I was close. Your comment got me squared away.

Erick Bongo
8,539 PointsI got a slightly stuck on this challenge as well. I realized you don't need to add if or else statements, since the challenge at this stage is all about learning the use of methods which are available in JS Take a close look at the code Jim uses in video before the challenge.
myArray.sort(function (a, b){
return a - b;
});
Hint: This code to this will work by adding the .length property in a particular set of places.

Mike Bronner
16,395 PointsExcellent use of code refactoring! Didn't think of that, but great solution.
Here's why it works (just to explain it if others are wondering):
- the function returns a positive number if a is larger than b.
- the function returns 0 if a is equal to b.
- the function returns a negative number if a is smaller than b.
So this means that the sort function is concerned if its exactly -1, 0 or 1, but any negative, 0, or positive will do. Learned something new here. :) Thanks Davie!

Erick Bongo
8,539 PointsYour welcome Mike.

Mike Bronner
16,395 PointsThe code you typed will most likely return null, as length is a variable that hasn't been instantiated in the inline function.
To create your own sort method:
- Create a new function that accepts 2 arguments.
- Compare the length of the two arguments, if the first one is shorter than the second one, return -1. If the first one is longer than the second one, return 1. If they are of equal length, return 0.
- Set the sort method of the array to your custom function.
This article (http://stackoverflow.com/questions/1129216/sorting-objects-in-an-array-by-a-field-value-in-javascript) should give you what you need. :)
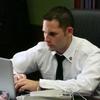
Kyle Brooks
6,753 PointsStill running into problems...
This is the latest.
<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort(function(a, b) {return (a.length < b.length) });
</script>
Error message keeps saying that I had the wrong order. Thoughts?

Mike Bronner
16,395 PointsI believe you are returning the wrong value in the sort function. True evaluates to 1, false evaluates to 0. This means that you're not considering -1.
Given your code, if a is shorter than b, it would return 1, but that is contrary to how the sort function works. It expects a 1 if a is longer than b. So, I would write out the if .. else if to cover all three scenarios.
- a > b => 1
- a == b => 0
- a < b => -1
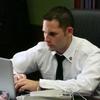
Kyle Brooks
6,753 PointsI am now beyond confused lol. Since it's intro to js, I'm hoping that I'm just overthinking this...

Mike Bronner
16,395 PointsSorry about that. Hang on for a moment, I'll go take the quiz and see exactly what's expected. :)

Mike Bronner
16,395 PointsOK, no -- we're on the right track here.
So, the last code snippet you have above needs to be adjusted a bit. You need to add the if-if else statement in, and return a value for each condition. This means you will have 3 return statements inside the function, each will be reachable only if they fulfill one of the 3 conditions (longer, equal, or shorter).
So to back up a bit: the sort method will essentially loop through the array and compare each element with the next (a and b). If it gets a 1 returned from the comparison, it knows that a is larger than b, if 0 they are the same, and if -1 a is shorter than b. That's why we add the if statements inside our custom sort function and have 3 return values, one for each possible condition.
I hope that made a bit more sense?
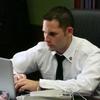
Kyle Brooks
6,753 Points<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort(function (a, b, c) {return (a.length < b.length) ; return (a.length > b.length) ; return (a.length === b.length)};
</script>
Now it says task 1 is no longer passing. I'm failing miserably here...do you know the answer so I can work my way backwards?

Mike Bronner
16,395 PointsNo worries, you're making progress. If you look at your code, you don't have any if-statements. Basically it will always return 1 or 0, so that won't work. Here's some pseudo-code to illustrate what needs to be in the braces of the custom sort function:
- Check if a is shorter than b, and if so return -1.
- Check if a is the same length as b, of so return 0.
- Return 1, as this will only be reached if the other two conditions are false.

Mike Bronner
16,395 PointsThe reason Task 1 failed is because you have 3 return statements without any conditions around them.

Mike Bronner
16,395 PointsAlso, the function only accepts 2 arguments, yet you are accepting 3: a, b, c. Remove c.
You may want to review how functions work, if this area is unclear to you: http://channel9.msdn.com/Series/Javascript-Fundamentals-Development-for-Absolute-Beginners/JavaScript-Functions-07
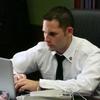
Kyle Brooks
6,753 Points<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort(function (a, b, c) {
(if a.length > b.length) return 1, (else if a.length < b.length) return 0, (else a.length === b.length)
return -1 });
</script>;
Hands in the air...i quit.
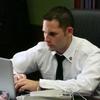
Kyle Brooks
6,753 Pointstried taking away the "c" too...
<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort(function (a,b) (if a.length > b.length return 1), (else a.length < b.length return 0),
(else if a.length === b.length return -1) });
</script>
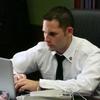
Kyle Brooks
6,753 PointsThanks for the help, Mike! The problem I was having was that I didn't see it in the last video. What you shared made total sense!
Happy New year!

Mike Bronner
16,395 PointsExcellent! Glad to hear it worked out and that you learned something along the way.
These "choke-points" we experience while learning new technologies are breakthrough-moments, once you overcome them you raise your understanding by another level. :)
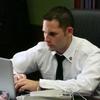
Kyle Brooks
6,753 Pointsfor sure. I will still say that my biggest weakness is JavaScript and jQuery.

Mike Bronner
16,395 PointsStick with it! :) Maybe take the same course a few times, before moving on in the track. Also, see if you can complete all JavaScript projects. By the end you should be well on your way to creating your own JavaScript scripts. :) Best of luck!
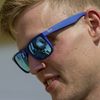
Patrick Koch
40,496 PointsWell I also had some probs with this excercises, thanks alot to Kyle and Mike, I had the right if statements but had prob with the numbers and letters!!
Made my day thanks
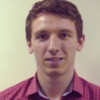
Matthew Aitken
6,558 PointsI have just recently started the Javascript module (The one I was least looking forward to working on) and I, too, was having trouble with this particular task. When reading through this thread it was not helping clear up my 'lack-of' understanding regarding this. Until the very end, that is. Lauren, the code you posted not only was as clear to read (I think that may be due to it being just one line and not a block) but instantaneously made me understand. So for that, thank you.
- MjA -