Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial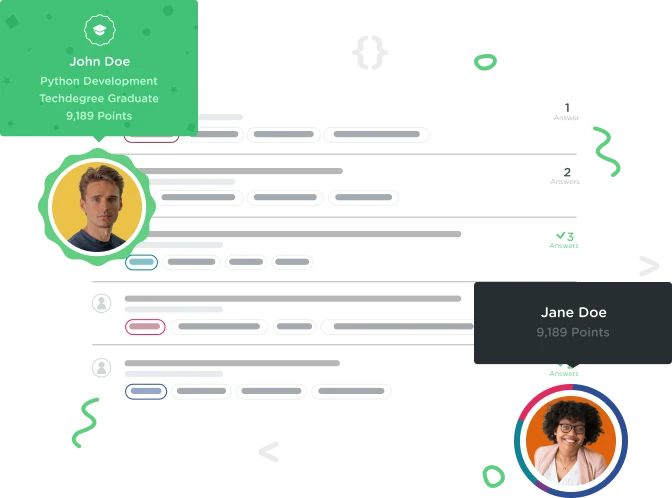

thanmai Deekshith
1,687 PointsCode Challenge Conditionals
Check if each student has a GPA of 4.0. If the student has a GPA of 4.0, use the student's name variable to display "NAME made the Honor Roll". If not, use the variable to display "NAME has a GPA of GPA". Would appreciate if someone could help me with this. my code:
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA >= 4.0){
echo "$studentOneName made the Honor Roll";
}
else{
echo "$studentOneName has a GPA of $studentOneGPA";
}
if ($studentTwoGPA >= 4.0){
echo "$studentTwoName made the Honor Roll";
}
else{
echo "$studentTwoName has a GPA of $studentTwoGPA";
}
?>

Jason Anello
Courses Plus Student 94,610 Pointsformatted code
19 Answers

Darron Brown
10,079 Points<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA == 4.0) {
echo $studentOneName . " made the Honor Roll";
} else {
echo $studentOneName . " has a GPA of " . $studentOneGPA;
}
if ($studentTwoGPA == 4.0) {
echo $studentTwoName . " made the Honor Roll";
} else {
echo $studentTwoName . " has a GPA of " . $studentTwoGPA;
}
?>
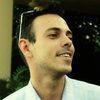
Roman Delcarmen
3,088 PointsGracias esta es la respuesta correcta
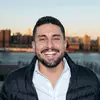
Andres Ramirez
18,094 PointsThis was a fairly easy solution. I would have expected to declare a new variable combining both the students etc etc. I'm sure this code can be simplified!
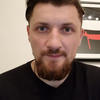
Igor Pavlenko
12,925 Pointsi like you formatting, did it this way. still works however your solution looks cleaner !!
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
if($studentOneGPA === 4.0) {
echo '$studentOneName made the Honor Roll' ."\n";
} else {
echo "$studentOneName has a GPA of $studentOneGPA" ."\n";
}
if($studentTwoGPA === 4.0) {
echo "$studentTwoName made the Honor Roll" ."\n";
} else {
echo "$studentTwoName has a GPA of $studentTwoGPA" ."\n";
}
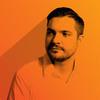
David Soards
18,368 Pointsthis is the worst challenge with the least amount of direction I have come across so far on Treehouse. Really bad. Please update this.
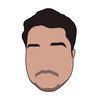
joseph arias
9,269 PointsI agree David Soards ... The challenges are very unclear in what they are looking for
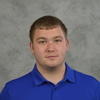
Cameron James
PHP Development Techdegree Student 4,535 Pointsi agree

Adam Sims
688 PointsFormatting on this is a real issue. PHP isnt fussy on whitespace, but this exercise is a different story.

nfs
35,526 PointsThis one's cleaner, shorter, concise and doesn't rely on type juggling:
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA === 4.0) {
echo "$studentOneName made the Honor Roll";
} else {
echo "$studentOneName has a GPA of $studentOneGPA";
}
if ($studentTwoGPA === 4.0) {
echo "$studentTwoName made the Honor Roll";
} else {
echo "$studentTwoName has a GPA of $studentTwoGPA";
}
?>
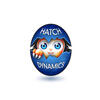
Zachary Scott
1,650 Points** This worked well for me:**
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Check Student One:
if ($studentOneGPA == 4.0) {
echo $studentOneName . "made the Honor Roll";
} else {
echo $studentOneName . " has a GPA of " . $studentOneGPA;
}
//Check Student Two:
if ($studentTwoGPA == 4.0) {
echo $studentTwoName . " made the Honor Roll";
} else {
echo $studentTwoName . " has a GPA of " . $studentTwoGPA;
}
?>
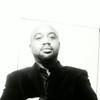
JEAN M CETOUTE
8,356 Pointsif ($studentOneGPA == 4.0) { echo $studentOneName . " made the Honor Roll"; } else { echo $studentOneName . " has a GPA of " . $studentOneGPA; }
if ($studentTwoGPA == 4.0) { echo $studentTwoName . " made the Honor Roll"; } else { echo $studentTwoName . " has a GPA of " . $studentTwoGPA; }

Jason Anello
Courses Plus Student 94,610 PointsHi thanmai,
The challenge wants you to check if the gpa's are equal to 4.0
You're checking if they are greater than or equal to 4.0
Also, this challenge is picky about how you've formatted your code. The else statement needs to be on the same line as the closing brace for the if statement.
Like this
if ($studentOneGPA == 4.0){
echo "$studentOneName made the Honor Roll";
} else {

thanmai Deekshith
1,687 PointsHi Jason, Originally my code had == then I encountered the same issue, hence changed it to >=. I hope there are no other errors apart from >=?
Thanks & Regards, Thanmai

Jason Anello
Courses Plus Student 94,610 PointsI also mentioned the issue with the else statement.
It needs to be on the same line as the curly brace ending the if.
You had it like this
}
else{
it needs to be like this
} else {
This is for the challenge only. It's requiring a very specific format.

thanmai Deekshith
1,687 Pointsthank you :)
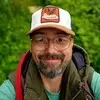
Graham Patrick
8,294 PointsNot working for me but its outputting correctly
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if($studentOneGPA == "4.0") {
echo "$studentOneName made the Honor Roll";
} else {
echo "$studentOneName has a GPA of $studentOneGPA ";
}
if($studentTwoGPA == "4.0") {
echo "$studentTwoName made the Honor Roll";
} else {
echo "$studentTwoName has a GPA of $studentTwoGPA ";
}
?>
outputting the right result! but not passing
Bummer! You need to check that $studentOneGPA is equal to 4.0
output is
Dave has a GPA of 3.8 Treasure made the Honor Roll
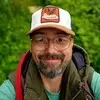
Graham Patrick
8,294 Pointssorted it !
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if($studentOneGPA == 4.0) {
echo "$studentOneName made the Honor Roll";
} else {
echo "$studentOneName has a GPA of $studentOneGPA ";
}
if($studentTwoGPA == 4.0) {
echo "$studentTwoName made the Honor Roll";
} else {
echo "$studentTwoName has a GPA of $studentTwoGPA ";
}
?>

Kenneth Kafunya
735 PointsRemove the "" around the 4.0 in the if statement
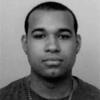
Gregori Benji Ootes Gonzalez
969 PointsI did the same code only with more spaces so that it looks better and it dint work. after I changed only the spaces and why spaces everything was working like it was the spaces.... why??

Jason Anello
Courses Plus Student 94,610 PointsThe challenges are usually looking for an exact match on the output. So you want to make sure that you follow any requirements in the instructions as well as maintaining any formatting that already exists in the starter code.
In other words, if the existing code is outputting everything mashed together on one line then your updated code should do the same.

Ramon Emilio Matos
849 PointsIn my opinion that statement does not have logic or sense. "Check if each student has a GPA of 4.0. If the student has a GPA of 4.0, use the student's name variable to display "NAME made the Honor Roll". If not, use the variable to display "NAME has a GPA of GPA". Would appreciate if someone could help me with this. my code:"

Ramon Emilio Matos
849 Pointsdoes not have logic or sense if de result is this " if ($studentOneGPA == 4.0) { echo $studentOneName . " made the Honor Roll"; } else { echo $studentOneName . " has a GPA of " . $studentOneGPA; }
if ($studentTwoGPA == 4.0) { echo $studentTwoName . " made the Honor Roll"; } else { echo $studentTwoName . " has a GPA of " . $studentTwoGPA; }"

Jason Anello
Courses Plus Student 94,610 PointsHi Ramon,
Can you clarify what you mean?

Ramon Emilio Matos
849 PointsI think the statement is not clear enough to solve the exercise

Ramon Emilio Matos
849 Pointsif the result is this "if ($studentOneGPA == 4.0) { echo $studentOneName . " made the Honor Roll"; } else { echo $studentOneName . " has a GPA of " . $studentOneGPA; }
if ($studentTwoGPA == 4.0) { echo $studentTwoName . " made the Honor Roll"; } else { echo $studentTwoName . " has a GPA of " . $studentTwoGPA; } "
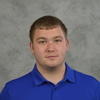
Cameron James
PHP Development Techdegree Student 4,535 Points<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA == 4.0) {
echo "$studentOneName made the Honor Roll";
} else {
echo "$studentOneName has a GPA of $studentOneGPA";
}
if ($studentTwoGPA == 4.0) {
echo "$studentTwoName made the Honor roll" ;
} else {
echo "$studentTwoName has a GPA of $studentTwoGPA";
}
?>
still says im wrong any ideas?

nathanielcusano
9,808 Pointsit gave me trouble as well.

nathanielcusano
9,808 Pointsyour "Roll" is spelt with a lowercase "r". It looks fine otherwise

nathanielcusano
9,808 Points<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA== 4.0) {
echo $studentOneName. " " . "made the Honor Roll";
} else {
echo $studentOneName. " has a GPA of $studentOneGPA";
}
if ($studentTwoGPA== 4.0) {
echo $studentTwoName. " " . "made the Honor Roll";
} else {
echo $studentTwoName. " has a GPA of $studentTwoGPA";
}
?>
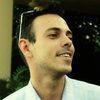
Roman Delcarmen
3,088 PointsI have to keep studying concatenation
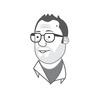
Steve Davies
10,389 PointsI have no idea what's going on here! :-/

Recep Onalan
15,541 PointsI made this and it worked
$studentOneName = ‘Dave’;
$studentOneGPA = 3.8;
$studentTwoName = ‘Treasure’;
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA == 4.0) {
echo “$studentOneName “ . “made the Honor Roll”;
} else {
echo “$studentOneName “ . “has a GPA of $studentOneGPA” . “\n”;
}
if ($studentTwoGPA == 4.0) {
echo “$studentTwoName “ . “made the Honor Roll”;
} else {
echo “$studentTwoName “ . “has a GPA of $studentTwoGPA”;
}
?>

James Osborne
5,693 Pointsthis is my answer yet it returns with "You will need to create separate if statements for each student"
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ( $studentOneGPA === 4.0 ) {
echo $studentOneName . ' made the Honor Roll';
} else if ( $studentOneGPA != 4.0 ) {
echo $studentOneName . ' has a GPA of ' . $studentOneGPA . "\n";
}
if ( $studentTwoGPA === 4.0 ){
echo $studentTwoName . ' made the Honor Roll';
} else if ( $studentTwoGPA != 4.0 ) {
echo $studentTwoName . ' has a GPA of ' . $studentTwoGPA . "\n";
}
?>
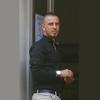
Dan Maliano
12,142 Pointsnot else if. just else...
Christian Andersson
8,712 PointsChristian Andersson
8,712 PointsSo what's the question?