Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial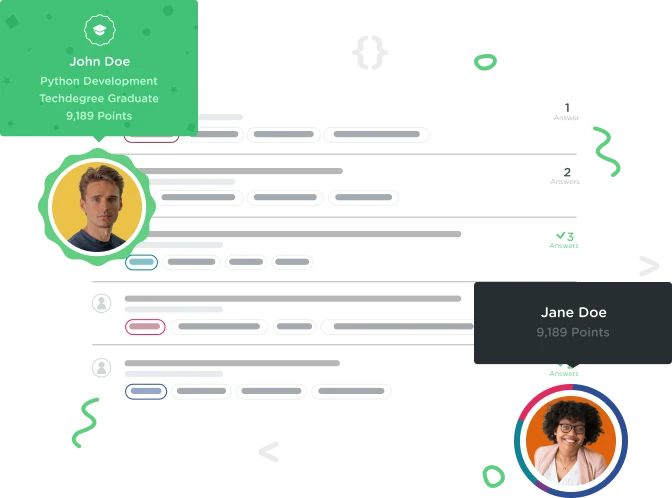

VIVIAN CENTENO
2,335 PointsCode Challenge: Create a function named max()
On the Code Challenge: Create a function named max(). ---I have been stuck on this challenge. I've tried different ways, but I really don't know what else could be the solution. Anybody?? Thanks in advance!
6 Answers
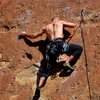
Shawn Flanigan
Courses Plus Student 15,815 PointsHi Vivian,
Let's take it step by step. They're asking us to set up a function named max()
, so let's start there.
function max() {
}
We're supposed to be able to pass this function 2 numbers as parameters. We can call them anything, but let's just call them a
and b
.
function max($a, $b) {
}
Next, we'll need to compare the two numbers with some if
statements. If a
is greater than b
, we'll want to return a
, so:
function max($a, $b) {
if($a>$b) {
return $a;
}
}
Likewise, if b
is greater than a
, we'll want to return b
.
function max($a, $b) {
if($a>$b) {
return $a;
} else if($b>$a) {
return $b;
}
}
In the real world, I'm sure we'd want to make sure these are both numbers...and we'd want to add a provision for a case where the numbers are equal, but for this exercise, the above code should pass.
Hope that all makes sense!

Micole Noddle
5,797 PointsHi Shawn, Thanks for your explanation, it really helps! If you have a sec, would you mind throwing the alert in there and explaining that a bit as well? Thanks again!

Alistair Olson
Courses Plus Student 4,283 PointsThank you Shawn. I struggled with this challenge as well. I believe I had it set up correctly, but when I used the numbers 13 & 66, the code failed. When I plugged in $a & $b, it worked like a charm. Clearly something I did was wrong, I'm just not sure what it was.

Duncan Sailors
5,644 PointsThanks Shawn! I was stuck on this for a while too. Your breakdown really helped!
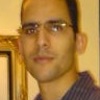
Samuel Mayol
4,786 PointsThis is the easiest way:
function max(number1, number2) {
if (number1 > number2)
return number1;
else
return number2;
}
alert( max(10, 20) );

Micole Noddle
5,797 PointsHow come we can just throw the numbers 10 and 20 into the alert and the code passes? I am so confused!
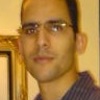
Samuel Mayol
4,786 PointsMicole, you can throw any pair of number that you want and will always return the maximum of those number, because the function validate the greatest of those.
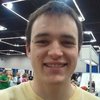
Michael Mayer
6,161 PointsDoes this work?
function max() { alert('im a function') }

VIVIAN CENTENO
2,335 PointsThank You so much Shawn Flanigan, your explanation is as clear as can be! Surely appreciate your time and effort, as well as others will too, I'm sure!
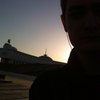
Maxim Levikov
6,546 PointsTry this solution:
var max = function () {
return a > b ? a : b;
};
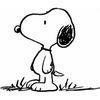
Ken Osborn
732 PointsThank you, Shawn!
I kept getting syntax errors since I was forgetting to include my condition within parenthesis.
Wrong:
if number1 > number2 {
Right:
if (number1 > number2) {
Herb Bresnan
10,658 PointsHerb Bresnan
10,658 PointsVivian, Please post your question along with the code from the challenge.