Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial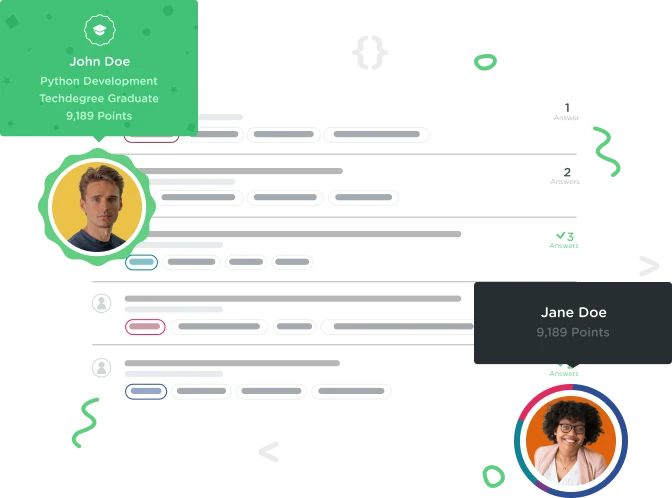
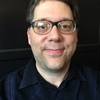
Chris Komaroff
Courses Plus Student 14,198 PointsCode Challenge - custom user manager - error "User's password wasn't set correctly"
Is there something I am missing, a hint I did not understand? This code is similar to Django docs "full example" https://docs.djangoproject.com/en/1.9/topics/auth/customizing/#a-full-example, as well as the example in the video.
Current error message: "User's password wasn't set correctly"
Thank you!
from django.contrib.auth.models import BaseUserManager
class UserManager(BaseUserManager):
def create_user(self, email, dob, accepted_tos=None, password=None):
if not accepted_tos or accepted_tos is None:
raise ValueError("TOS not accepted or missing!")
user = self.model(
email=self.normalize_email(email),
date_of_birth=dob,
)
user.set_password(password)
user.save()
return user
1 Answer
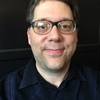
Chris Komaroff
Courses Plus Student 14,198 PointsWell I accidentally cheated when I started work on the next code challenge for custom user model (on the next video):
user = self.model(
email=self.normalize_email(email),
dob=dob,
accepted_tos=True
)
So you have to set accepted_tos=True "explicitly", maybe if accepted_tos argument is either not boolean or maybe is not well defined. Also the dob field really 'dob' and not 'date_of_birth' like BaseUserManager has in docs (I think).
The error message "User's password wasn't set correctly" was bogus. Kinda thought so since it was the easiest part of the code challenge.
Bernardo Augusto García Loaiza
Courses Plus Student 792 PointsBernardo Augusto García Loaiza
Courses Plus Student 792 PointsChris Komaroff thanks for the tip.
My code section stayed of this way: