Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial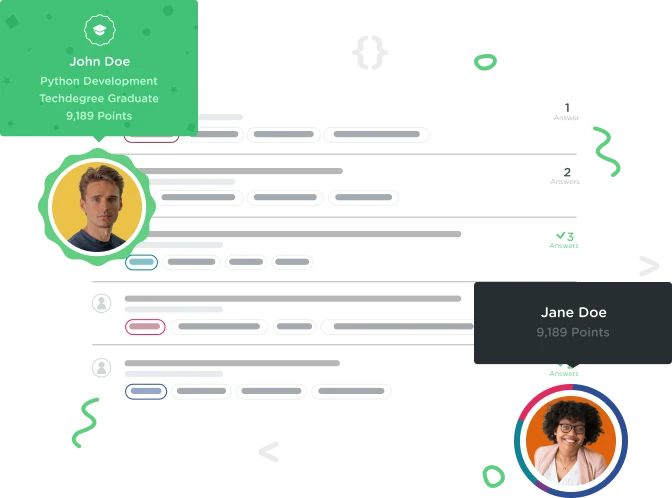

ISAIAH S
1,409 PointsCode Challenge "Defaulting Parameters" -------------------------------------------------- Challenge Task 1 of 1
Not really clear what I am supposed to do.
Challenge Task 1 of 1
So at your new Java job, you've written a brand new Shopping Cart system for the website. After some developers have used the objects you created for a while, they ask you to make it easier to add things to the cart.
Check out more instructions in Example.java. Once you implement their request, check your work, and you will pass the challenge.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
// cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers
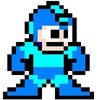
Robert Richey
Courses Plus Student 16,352 Points// here is the method given to us
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
// create a new method signature that accepts only an item
public void addItem(Product item) {
// call addItem passing in two arguments: item, 1
// this will call the above addItem function and keep your code DRY
}
// once that is done, go back to Example.java and uncomment the line below
// cart.addItem(dispenser);

Chetram Chinapana
1,793 PointsRobert Richey thank you for explaining but this defaulting parameter thing is hard to grasp. After a whole week of trying to fiddle around with it without the System.out.printf statement I gave up. After adding public void addItem(Product item){ int quantity = 1; } my compiler is saying that the Pez count is not 1, to uncomment the line, but I did uncomment it in Example.java. I am trying to do it Dennis' way but the method is not taking it.
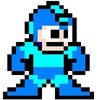
Robert Richey
Courses Plus Student 16,352 PointsYep, this can be a hard topic to understand initially. At this point, the best I can offer is some documentation that may help explain things in a different way. Eventually, you'll need to get comfortable with reading 'official' docs. I try not to be a "Read The Friendly Manual" kind of guy, but in this case, I'm not sure how else to try and explain it.
Here is Oracle's attempt at explaining methods, and I think they do a good job at it. You may need to scroll down a bit to find the section labeled Overloading Methods.
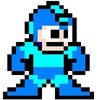
Robert Richey
Courses Plus Student 16,352 PointsEdit: The answer has been updated to reflect a DRY approach and what Craig was trying to get us to do all along. To reduce confusion, I'm deleting the rest of this comment, as it points out two other - unintended, and unintuitive ways of completing the challenge.
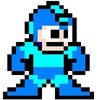
Robert Richey
Courses Plus Student 16,352 PointsHi there,
The Method Signatures video explains this pretty well.
The idea is that we need a method that can be called with only an item and, by default, add 1 item to the cart. Other languages can allow default parameters to be defined, but in Java this is done by writing a new method signature - or, overloading a method.
// here is the method signature given to us
public void addItem(Product item, int quantity) {
}
// we need another method signature that when called with just an item,
// will by default add 1 of that item to the cart
// once that is done, go back to Example.java and uncomment the line below
// cart.addItem(dispenser);
Please let me know if this helps or not.
Cheers

ISAIAH S
1,409 PointsI still don't understand how to finish the challenge.

Chetram Chinapana
1,793 PointsI posted to the wrong thread. Could you see my comment below.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherCall the
addItem
method and pass it 1. Do not do the System.out command. I thought I caught that in the test.Natenda Manyau
3,005 PointsNatenda Manyau
3,005 PointsI still don't get exactly what am supposed to do in which .java file
confused
ISAIAH S
1,409 PointsISAIAH S
1,409 PointsThanks!! I had been stuck on that one for days. :-)
Giancarlo M.
1,502 PointsGiancarlo M.
1,502 PointsThank you, this helped so much!
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHi Giancarlo,
While my previous answer worked, it was not the intended 'good' answer. This challenge wants us to practice being DRY with our code, so I've updated the comment in the new method signature to reflect this new understanding.
Craig Dennis, sorry bud - I wasn't thinking DRY when going through this the fist (several) times. Better late than never!
ISAIAH S
1,409 PointsISAIAH S
1,409 PointsThanks!!