Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial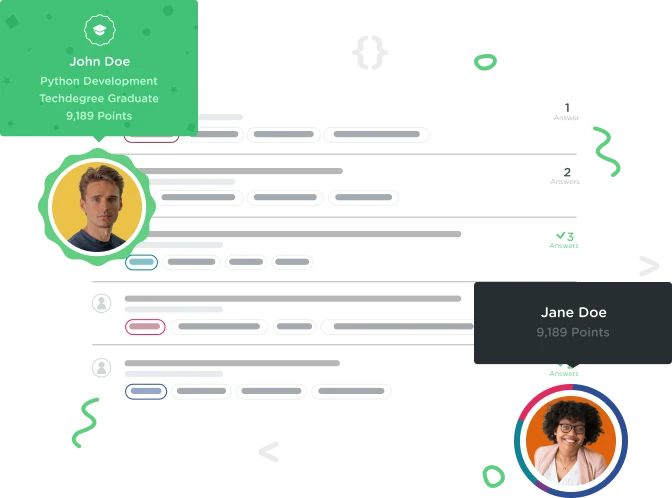

John Locken
16,540 PointsCode Challenge: disemvowel.py ; only removing first vowel, what is my error?
I'm trying to complete the disemvowel.py challenge and I'm unsure what my mistake is. I wanted to do it by turning my string into a list then deleting the list entries. After that I can turn the list back into a string. Below is my code:
def disemvowel(word):
vowels = 'aeiouAEIOU'
wordlist = list(word)
vowellist = list(vowels)
wordlist2 = wordlist
for letter in wordlist2:
for lettercheck in vowellist:
if letter == lettercheck:
wordlist.remove(letter)
continue
word = ''.join(wordlist)
return word
I thought I needed to create a mirror of wordlist and check that against vowellist in order to keep it from missing vowels in the middle, but it still only deletes the first and last vowels from the list. What am I missing? While we're at it, is there any unnecessary code I need to clean up?
1 Answer

andren
28,558 PointsWhat am I missing?
It is hard to judge your code properly since you have posted it without proper formatting. Since spacing actually affects how Python reads your code. I made an educated guess that your code is supposed to look like this:
def disemvowel(word):
vowels = 'aeiouAEIOU'
wordlist = list(word)
vowellist = list(vowels)
wordlist2 = wordlist
for letter in wordlist2:
for lettercheck in vowellist:
if letter == lettercheck:
wordlist.remove(letter)
continue # Not sure where this belongs, but it doesn't really matter either way
word = ''.join(wordlist)
return word
The reason why your code fails is that despite making two separate variables (wordlist
and wordlist2
) you actually only have one list containing the word. In Python when you assign a list to a variable you in reality assign it a reference to a point in memory where the list is stored. When you set another variable equal to that list what actually gets set to the variable is not the contents of the list but the reference to the list. Meaning that both variables now point to the same location in memory.
This means that while there are two variables pointing at the list there is only one actual list existing. So any modifications to that list, be it through wordlist
or wordlist2
will end up changing that list for both of them. The way around this is to use the copy
method built into lists, this method returns a copy of a list that is independent of the original. So if you change wordlist2 = wordlist
to wordlist2 = wordlist.copy()
then your code will actually pass.
While we're at it, is there any unnecessary code I need to clean up?
There are a couple of improvements that can be made:
- You don't really need to create two separate list variables to store the word.
- The vowel variable should start out as a list, there is no need to store a string in it and then have Python convert that to a list. And even if there was you could just reuse the
vowel
variable instead of creating a new variable to hold the list. - Looping through the vowel list is not necessary. It is better to just us the
in
operator to check if theletter
string is found within the vowel list. - The
continue
keyword causes Python to move to the next item in the loop instead of executing the rest of the code in the loop, but since yourfor
loops only contain one action there is no code to skip, making use of the keyword entirely redundant. - You can return the joined wordlist directly without storing it in a variable first.
Here is a revised version of your code:
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u'] # Store list in vowels variable
wordlist = list(word)
for letter in wordlist.copy(): # Loop through copy of wordlist
if letter.lower() in vowels: # Use in operator to check if letter is in the list.
wordlist.remove(letter)
return ''.join(wordlist) # Return joined wordlist
You might notice I also removed the uppercase letters from the vowel list, this is because you can simply use the lower
method on the letter as you compare (but not when you remove) it and get the same result.

John Locken
16,540 PointsMuch obliged, sir. I posted it as I ran out the door and didn't pay any attention to formatting. thanks for the detailed response!
John Locken
16,540 PointsJohn Locken
16,540 PointsPS here is the last error I received when running it: Hmm, got back letters I wasn't expecting! Called disemvowel('YHiAdkNdi') and expected 'YHdkNd'. Got back 'YHAdkNd'