Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial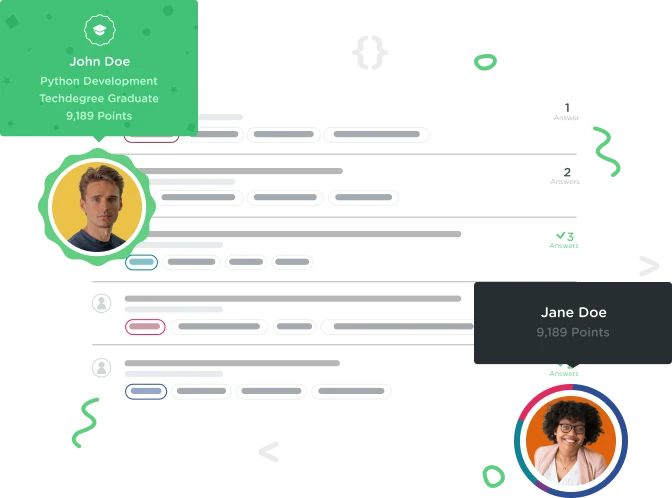

Julia Rix
3,303 PointsCode Challenge for Things That Count
I have been working on this challenge for an hour just plugging variable and changing syntax to try and make it work. I can get through the first two steps but then the third step shoots me back an error message that reads task 1 no longer works. Can anyone help? I know it must be something simple that I am missing.
age = int("37") days = int("52 * 7") * age summary = ("I am " + days + " days old!")
age = int("37")
days = int("52 * 7") * age
summary = ("I am " + days + " days old!")
3 Answers
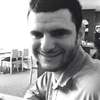
Dan Garrison
22,457 PointsHi Julia,
A few things with your code. First, you don't need to to turn your numbers into strings and then convert them with int(). Instead, just give the value of the variable the number without a quotes. Without the quotes, the number will be an integer by default.
For the summary variable, however, you need to convert the integer value into a string. To do this you will use the str()
There are a couple ways you could do this. I prefer using format, which would like this:
age = 28
days = (age * 52) * 7
summary = "I am {} days old!".format(str(days))
You could also do the same thing without the format.
age = 28
days = (age * 52) * 7
summary = "I am " + str(days) + " days old!"
You just have to remember to convert your integer values to a string. Otherwise it won't work.

Julia Rix
3,303 PointsThank you Dan! My notes were a bit messy and I didn't quite articulate clearly how to use the str.format() function in them. Guess that's why it wouldn't work. :)
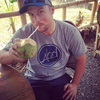
Dave Campbell
13,310 PointsOne other way to write your summary string would be:
age = 28
days = (age * 52) * 7
summary = "I am %d days old!" % (days)
print summary

Jason Anello
Courses Plus Student 94,610 PointsYou would need to put parentheses around summary because the code challenges are running python 3.x and print is now a function.
print (summary)
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsYou don't have to convert to a string when using
.format
but you do with string concatenation.Dan Garrison
22,457 PointsDan Garrison
22,457 PointsAh! Good to know. Thanks!
Matt Mortensen
2,730 PointsMatt Mortensen
2,730 PointsJason Anello, sorry I'm a little confused by your comment. Could you explain more?
Dan Garrison
22,457 PointsDan Garrison
22,457 PointsHi Matt,
Jason is saying that when you use
.format
, you don't need to usestr()
to convert the variable to a string. Using.format
will do the work for you. If you use string concatenation, like I did in my second example, you have to use `str()
to convert your integer values into a string. Does that make more sense?Matt Mortensen
2,730 PointsMatt Mortensen
2,730 PointsThank you so much Dan! That makes perfect sense now.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsThanks for clearing that up Dan!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Pointsselected best answer