Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial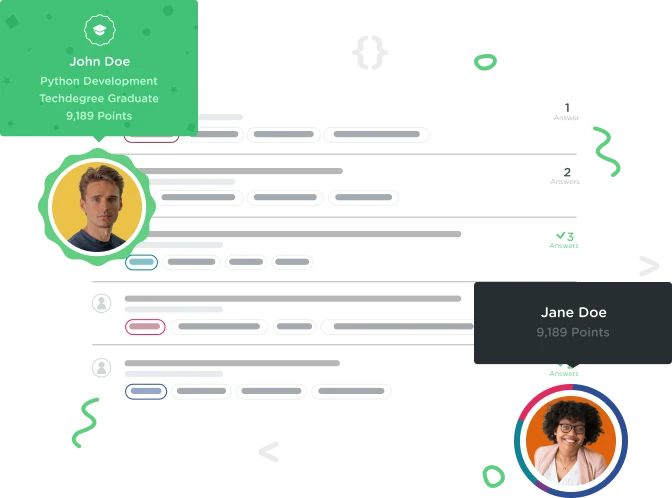
Jack Hill
1,168 PointsCode Challenge Help
This is Android Devolopment and the instructions were to: "Inside the "try" block, add an HttpURLConnection variable named "connection". Initialize it using the openConnection() method of the treehouseUrl variable. The openConnection() method returns a URLConnection object, so don't forget to cast it to HttpURLConnection."
so far this is what I have:
package com.example;
import android.os.Bundle;
import android.view.View;
import java.io.IOException;
import java.net.MalformedURLException;
import android.util.Log;
public class MainListActivity extends ListActivity {
public static final String URL = "teamtreehouse.com";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
try {
URL treehouseUrl = new URL(URL);
}
catch (MalformedURLException e) {
Log.e("CodeChallenge", "MalformedURLException caught!", e);
}
catch (IOException e) {
}
}
}
Can anyone help me with this one?
8 Answers
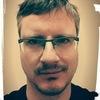
Steve Bedard
14,335 PointsFirst, start with something you already know which is to create a HttpURLConnection object named connection and from there you can initialize it with the openConnection method
structure hint:
double myDouble = 25.4
int number = (int) myDouble.makeMeAnInt();
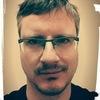
Steve Bedard
14,335 Pointsmy makeMeAnInt method does not really make sense since that's what the cast will do but it could be any method. I hope you get the point.
Jack Hill
1,168 Pointsall i have is
HttpURLConnection connection =
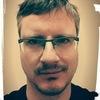
Steve Bedard
14,335 PointsYou're halfway there. To the right of the statement start your cast. (HttpURLConnection) and cast what should be cast. hint: URL objects have an openConnection() method, so you can you this on your cast with URL object already created for you.

Krishna mohan dubey
Courses Plus Student 698 Pointswhat is actual anwer if i typed inside try block HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
then what is going wrong with this. I am conffuged about that .
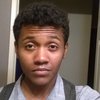
Ricky Sparks
22,249 Pointswont pass?
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();

William Lawson
iOS Development with Swift Techdegree Student 3,597 PointsHey Jack Hill,
I just completed this challenge try this.
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
hope this helps,
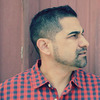
Juan Gallardo
1,567 PointsThe answer is
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
It fits into the rest of the code like this
package com.example;
import android.os.Bundle;
import android.view.View;
import java.io.IOException;
import java.net.MalformedURLException;
import android.util.Log;
public class MainListActivity extends ListActivity {
public static final String URL = "teamtreehouse.com";
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
try {
URL treehouseUrl = new URL(URL);
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
}
catch (MalformedURLException e) {
Log.e("CodeChallenge", "MalformedURLException caught!", e);
}
catch (IOException e) {
}
}
}
Jack Hill
1,168 Pointslol I don't