Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial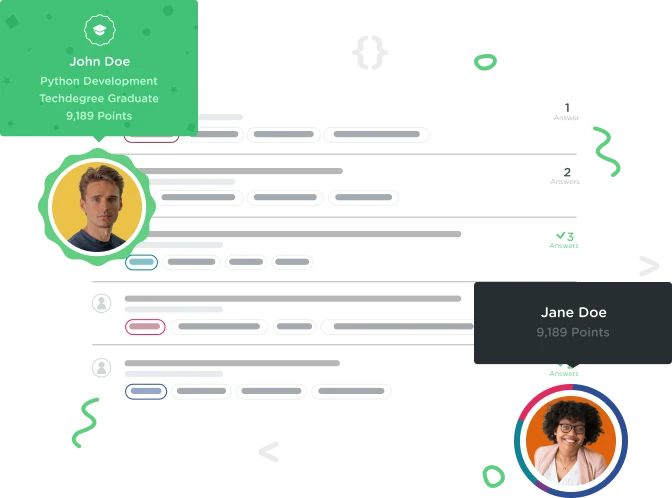

fahad lashari
7,693 PointsCode challenge help please
I am not really sure how to solve this problem. The question asks to produce a string which looks like "Warrior, <weapon>, <attack_limit". However once I achieve this, it expects specific values on these attributes. I am completely lost as to what piece of this puzzle I am missing.
Any help would be greatly appreciated.
Kind regards,
Fahad Lashari
from character import Character
class Warrior(Character):
weapon = "sword"
attack_limit = 20
def rage(self):
self.attack_limit = 20
#def __init__(self, **kwargs):
#for key, value in kwargs.items():
#setattr(self, key, value)
def __str__(self):
return "{}, {}, {}".format(self.__class__.__name__,
Character().__class__.weapon,
Character().__class__.attack_limit)
# This is the error I get: Bummer! Expected "Warrior, axe, 40", got "Warrior, stick, 10".
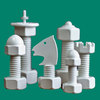
Steven Parker
231,572 PointsIt looks like you asked about this already.
I posted an answer to the other copy of this question.
1 Answer
Dustin James
11,364 PointsYou are calling:
Character().__class__.weapon
Warrior is inheriting Character.
def __str__(self):
return "{}, {}, {}".format(self.__class__.__name__,
self.weapon,
self.attack_limit)
I hope this helps.

fahad lashari
7,693 PointsYep that worked brilliantly thanks. I guess I was just over complicating things.
Much appreciated!
Kind regards,
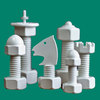
Steven Parker
231,572 PointsEven that's a bit fancier than needed. Just this would do:
return "Warrior, {}, {}".format(self.weapon, self.attack_limit)
Dustin James
11,364 PointsSteven Parker,
In the video, Ken demonstrates how to get the name of the class by using:
self.__class__.__name__
Hence the reasoning for using this format.
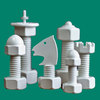
Steven Parker
231,572 PointsHi Dustin James,
I understand that it does work and pass the challenge, but if you read the challenge requirements carefully you will see that "Warrior" is not shown in angle brackets, indicating that it need not be inserted with a placeholder and that you may include it verbatim in the format string.
This does, in fact, pass the challenge also.
fahad lashari
7,693 Pointsfahad lashari
7,693 PointsHi guys, I couldn't really figure out the answer so out of frustration I just cheated and passed the the challenge anyway using this code lol
I just went ahead and set the value the challenge was expecting, explicitly and by doing so the check that makes sure that the value expected are correct got the right values and passed it. I couldn't find a way around it