Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial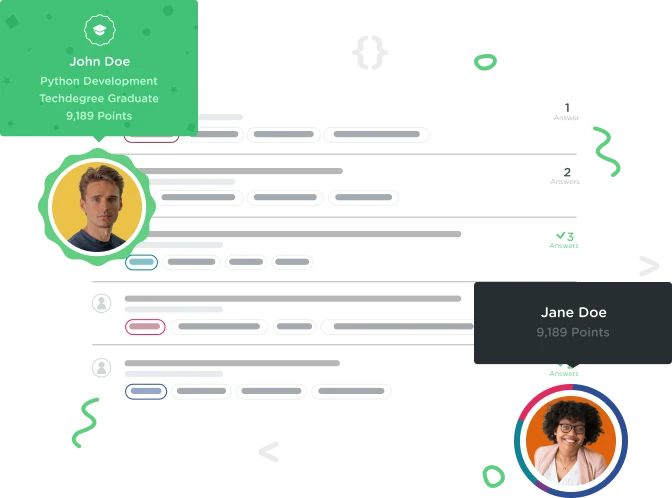

Bob Smith
6,711 PointsCode Challenge: Method 1: Ordering
I can't seem to figure out why this is not working? I clearly don't understand how to order strings by length. I know I am being really dim and its extremely frustrating. Any help would be much appreciated.
Sort the 'saying2' array, on about line 19, so that the words are listed in length order. The shortest first. Use the 'length' property on the strings in the sort function.
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort(function (a, b) { return a.length < b.length;});
7 Answers
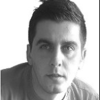
Emilio Queiroz
3,710 Pointsvar saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse(); saying2.sort(function (a, b) { return a.length - b.length;});
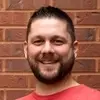
Nick Kohrn
36,936 PointsShea,
I, too, used the greater-than (>) operator at first, but after a little browsing this is what I came up with after checking out a description of the sort() function at Mozilla Developer Network.
If you were to use the greater-than (>) or the less-than (<) operators, then you would be returning a boolean value (true or false). By using the minus (-) operator, you are returning an integer, which is what a.length returns. The sort() function is then using that integer as a way to place the strings in ascending order by length.
For example, assume that you have an array with three strings that you want to sort in ascending order according to the length of each string.
var array = ["The", "quick", "duck"];
array.sort(function (a, b) {
return a.length - b.length;
});
The function is returning an integer from b.length being subtracted from a.length. Looking at the first two strings, you would be comparing these values:
return a.length - b.length
This translates to:
return 3 - 5
3 - 5 returns a negative number (-2). This means that a.length must be a lower value than b.length. Therefore, they are already in ascending order, with (a) having a length of three characters and (b) having a length of five characters. The sequence then moves to the next two strings in the array.
Now, let's compare the second and third strings in the array, which are "quick" and "duck":
return a.length - b.length
The second string is "quick" and the third string is "duck". This now translates to:
return 5 - 4
5 - 4 returns a positive number (1). This means that a.length must be a higher value than b.length. Therefore, they need to be swapped in place, resulting in "duck" coming before "quick".
This is able to be done because it is comparing two integers and using the returned value from the minus (-) operator to know which value comes first.
Simply stated, the sort function does not accept boolean (true or false) values, which the greater-than (>) and less-than (<) operators return.
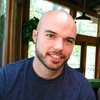
Shea Knox
12,254 PointsThank you for taking the time to write that out! Definitely cleared that up for me. Truly appreciated.

Farhat Jehan
7,268 PointsThanks :)

Bob Smith
6,711 PointsNeeded to use a minus instead of a 'less than' symbol. Uhhhh.
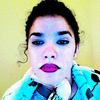
Emelin Burgos
1,067 PointsThis was helpful

ROBERT ROBINSON
7,723 Pointsdid the same thing. totally get it now...
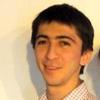
Matias Valenzuela
7,554 PointsThis is the answer my friend:
saying2.sort(function (a, b) {
return a.length - b.length;
});
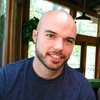
Shea Knox
12,254 PointsCan anyone explain why that is the correct answer? I'm having a hard time understanding the logic behind it. I too used a.length < b.length thinking this would order them from shortest to longest. If someone could explain what exactly is going on in the function to return the value we're looking for that would be very helpful. Thanks in advance!
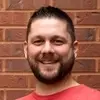
Nick Kohrn
36,936 PointsShea,
I left an explanation that might help you with the concept of the sort() function. I hope it helps!