Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial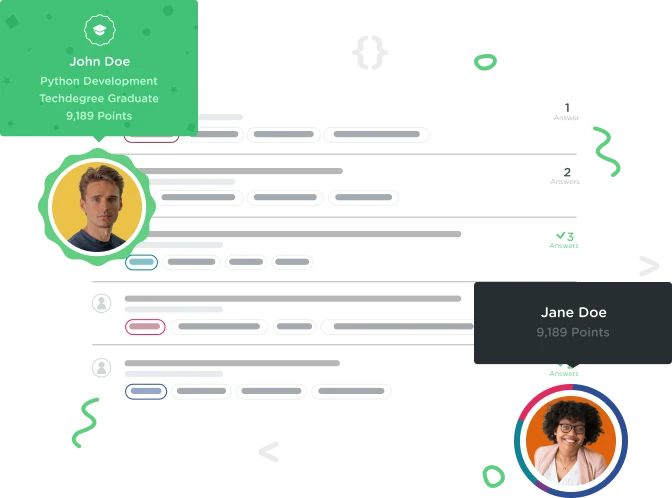

Lawrence Lee
4,240 PointsCode Challenge: Null and Undefined
Hi there,
I am stuck at fixing the code in this code challenge.. Here is the question:
The identical() method is being called but the variables are not identical. See if you can fix the code.
Here is what the code provided in text editor:
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable == myUndefinedVariable) {
identical();
}
Here is what I came up with:
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable === myUndefinedVariable) {
identical(null);
} else {
identical(null);
}
And I received error message says null, so i changed my code to the following:
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable === myUndefinedVariable) {
identical();
}
And I got correct this time....I am not sure how I got it right...by changing the "==" to "===" and not adding the "else" statement... Can someone explains why I getting this question right?
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsYou didn't need the else
statement in the first place. The task was to call a function in case those two variables were equal. The function itself wasn't your concern, it was the comparison.
JavaScript does something called type coercion when you compare two variables with ==
. It'll convert datatypes automatically so it can compare them. Both undefined
and null
are falsy values, they're treated as false
when compared with ==
.
The goal here was to avoid type coercion and check for true equality (when types also match), and that's what the ===
operator does. That's why the third example worked.
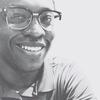
Ricardo Hill-Henry
38,442 PointsI would assume the last works because in theory two items compared with == can technically be non identical. For example a floating point value of 2 and an int (fixed number) value of 2 would be considered identical when comparing with ==. The difference is when using === the two values being compared would have to be the same type (int and int, not double and int) and have the same value. So when comparing values a floating point 2 and a fixed number 2 would not be the same. Though Javascript does't explicitly set types, they do apply when using ===.
In relation to this challenge I think the following link will explain it better than myself. http://thecodeship.com/web-development/the-undefined-vs-null-pitfall-in-javascript/

Dino Paškvan
Courses Plus Student 44,108 PointsJavaScript does not have separate datatypes for floating point numbers and integers. Instead, it has a single numerical datatype called number
. Because of that, your example doesn't really show off type coercion.
A much better example would be "2" == 2
where a string
appears equal to a number
through type coercion.

John Crillo
8,293 PointsI plugged the correct answer in the Javascript console tool on Chrome and it came back undefined instead of true so I'm not sure if that was the right answer or not. If anyone could follow up with any input it would be greatly appreciated.

Dino Paškvan
Courses Plus Student 44,108 PointsThe correct answer is not supposed to be true as null
and undefined
are not identical.
The undefined
you get in the JavaScript console is the return value of the if
statement.