Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial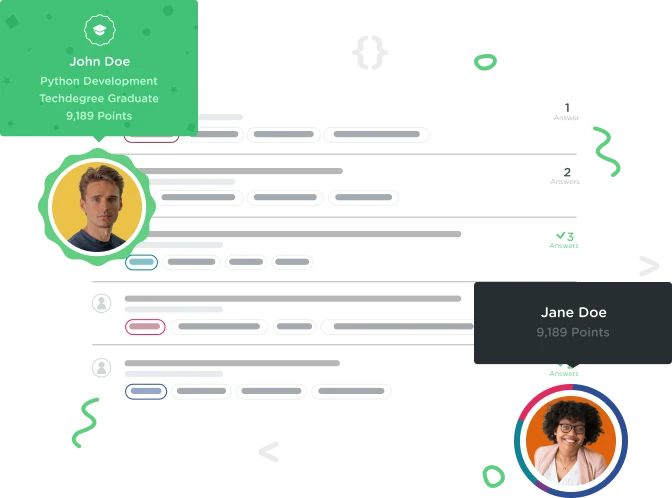

Philipp Ruthensteiner
3,050 PointsCode Challenge Object-Oriented Swift. Stuck with the typ "Location"!
Challenge Task: In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values. For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location. In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(
10 Answers
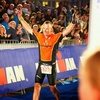
Steve Hunter
57,712 PointsYou're doing fine!!
Create a new Location
object as the parameter:
let someBusiness = Business(name: "The Biz", location: Location(latitude: 3.1, longitude: 58.2))
Make sense?
Steve.

Philipp Ruthensteiner
3,050 PointsHey Steve! Makes a lot of sense! Thank you!
Best Philipp

Pham Minh Dat
1,873 PointsHi Steve,
Can you please take a look at my code and verify if it's correct or not? Thank you!
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init (name: String, latitude: Double, longitude: Double) {
self.name = name
self.location = Location (latitude: latitude, longitude: longitude)
}
}
let someBusiness = Business (name: "Apple", latitude: 3.1, longitude: 58.2)
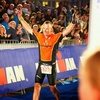
Steve Hunter
57,712 PointsHi there,
Your code works OK, yes, in that it creates an instance of Business
that contains the correct information - it is an interesting solution in concept; the output is correct.
However, it doesn't meet with the requirements as set out in the challenge. The initializer is expected to be able to handle two parameters, a name
string and a Location
object. Your code cannot handle those inputs and so will fail the challenge.
It is important to consider the inputs, not just outputs, when creating code. Knowing what you have to work with is critical to deliver the results required. Just creating the correct end-position is not all what it is about - the route you take is also important. Whilst this is a simplistic example, let's say your co-workers in another development team were tasked with generating a Location
object to pass to your code; their correctly written output would not match your required inputs because your code is unable to handle the Location
object.
Make sense?
Steve.

Pham Minh Dat
1,873 PointsThanks for providing such a detailed reply, Steve :)
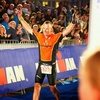
Steve Hunter
57,712 PointsHey, no problem!

Siamak Pourhabib
2,962 PointsHi Steve, my code passed the quiz but I did not pass in the name in the initializer method as it is in above answer. I am confused..
class Business {
let name: String = ""
let location: Location
init(name: String, location: Location){
self.location = location
}
}
let someBusiness = Business(name: "Bookshop", location: Location(latitude: 12.3, longitude: 3.4))
[MOD: edited code block -srh]
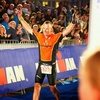
Steve Hunter
57,712 PointsHi there,
I don't think the actual name of the business matters. The code needs to handle a name which is a string and a location that's a Location
.
Make sense?
Steve.

Siamak Pourhabib
2,962 Pointsyea it makes sense, but on the console it just shows the empty string instead of the business name.
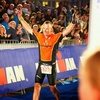
Steve Hunter
57,712 PointsYou haven't assigned the incoming parameter for the name
constant inside your init
method.
You need to add:
self.name = name
within your initialiser.
You are getting an empty string because you have assigned an empty string to every instance of this class. To fix that, remove the assignment at the top of your class. Just have var name: String
rather than assigning an empty string to it.
The final code should look like:
class Business{
let name: String // <- remove = ""
let location: Location
init(name: String, location: Location){
self.name = name // add this
self.location = location
}
}
let someBusiness = Business(name: "Biz", location: Location(latitude: 1, longitude: 2))
Steve.
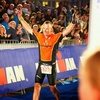
Steve Hunter
57,712 PointsSorry - just read your post more thoroughly! Your code shouldn't pass the challenge; I will report a bug to the development team. The tests must just look for an output result, rather than expecting the input to match the output.

Siamak Pourhabib
2,962 PointsI got it, Now I know why I was getting an error when I tried to add "self.name = name" offering me to change "let" to "var" to make it a mutable value . I just needed to remove that empty string. Thank you for your help I appreciate that.
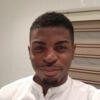
Nnanna Okoli
Front End Web Development Techdegree Graduate 19,181 PointsThe wording for this question was really poor, especially in the latter phrases :(
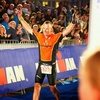
Steve Hunter
57,712 PointsThis post is about Swift 2.0 - that's well out of date. Is the course still live?! I thought they'd been retired?
Which challenge are you having a problem with? I'll try to help.
Steve.
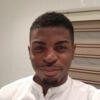
Nnanna Okoli
Front End Web Development Techdegree Graduate 19,181 PointsYes, this course is still live, I'm currently on the beginning iOS track and this topic Object-Orientated swift is within this track. Honestly, I've been struggling hard to keep up with the challenges as I feel the questions have been worded poorly or the video tutorial examples do not do enough to help you answer the challenges in my opinion.
I have passed the challenge code for now but it seems like for me I keep coming on this forum to seek answers and it's not doing me any good for my confidence.
I do not fully understand the use of struct or methods and especially instances in this content but I'm a beginner and I'm still learning.
You seem experienced Steve, do you reckon I should continue this course 'beginner for iOS' if not what do you recommend?
Many thanks in advance for taking the time to read this...
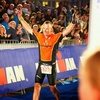
Steve Hunter
57,712 PointsHiya,
Yes; keep going. Your challenges are the same as we all faced. Ask questions in the forums here - you'll learn loads. Don't put yourself down - be positive, you are doing great already! (No, I'm not paid by Treehouse, I'm just a volunteer)
You can @ mention me, like on Twitter and I'll try to answer your questions. Swift isn't my thing, but I know how to write an iOS app. And this course has been retired on January 15, 2017. I think the forum might link to the same subject but from a different course.
Not to worry - keep asking questions - that's the best way to learn. Check out my profile; I am reachable in many ways, but keeping it in here is the best, for me.
Did you get this challenge passed?
Steve.
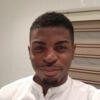
Nnanna Okoli
Front End Web Development Techdegree Graduate 19,181 PointsOkay understood, i'll keep going. I'm excited to finish this course anyway. And yes I managed to pass the challenge thanks for the help
I followed you on twitter I'll give you a shout if I need anything cheers man!
Nnanna @1k__n7
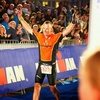
Steve Hunter
57,712 PointsAh - yes, I saw the follow and wondered how the hell we were connected!! If I can help; I will. Just shout.
Steve.
Rich Braymiller
7,119 PointsRich Braymiller
7,119 PointsSteve thanks so much for always helping out...def helps !
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsDjon Sm
iOS Development Techdegree Student 179 PointsDjon Sm
iOS Development Techdegree Student 179 PointsHello Steve, can you check whether my code is correct or not? It passed the task:
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsWill do... I'll be back in the office soon so I will look then.
Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Djon Sim!
For some reason I'm getting a 404 on the challenge page right now so can't check the wording of the question.
However, from memory, (and bear in mind this post is a year old!) the idea of the task was to pass a
Location
object into theBusiness
class when instantiating aBusiness
object. That's not what your code does. You have created an elegant solution to work around the problem but your code doesn't actually fit the task in hand, even if the tests of the challenge aren't clever enough to work that out!So, well done on creating an interesting solution to the challenge, but do think about what the requirements of the task set out before you. Plus, if you omit the
init
method and set up theLocation
object within the call for a newBusiness
object, you save on lines of code. While that's not always a good thing, it is often strived for.Let me know if I can explain that more thoroughly; happy to help.
Steve.
Djon Sm
iOS Development Techdegree Student 179 PointsDjon Sm
iOS Development Techdegree Student 179 PointsCould not find how to reply to your recent comment ;) Your explanations are quite clear, thanks. The only thing I could not understand is: " if you omit the init method and set up the Location object within the call for a new Business object, you save on lines of code. While that's not always a good thing, it is often strived for."
I am not so good in classes yet, however as I understand it is a must to specify init method, so how can I omit it? ;)
And how will the shorten line of code look like? If I am not asking too much ;)
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Djon Sim!
Two things, first of all. 1. apologies; I got some of that answer wrong because I had a different code challenge in mind - I'll correct that now, and, 2, you can never ask too much! Ask away! I love answering questions as it helps me keep fresh too,
init
methods aside. So, let's sort out my bad answer first ...Yes, you are correct, every class needs an
init
method. So ignore that part of my answer (I had a challenge in mind where there are twostructs
, not a class - that's why I wanted the challenge link to work!). My bad. I apologise unreservedly.But the rest of my post remains the same. To instantiate a
) which requires two
Business
class object, it should take two parameters; aname
which is aString
and aLocation
instance. ALocation
is astruct
(which doesn't need aninit
methodDouble
numbers, as you know. So, when you instantiate yourBusiness
object, pass aLocation
into theinit
` method.This looks like:
let someBusiness = Business(name: "The Biz", location: Location(latitude: 3.1, longitude: 58.2))
So, here, we are creating a
Location
inside the call to theinit
method of theBusiness
class.Does that make sense?
Steve.
Djon Sm
iOS Development Techdegree Student 179 PointsDjon Sm
iOS Development Techdegree Student 179 PointsYep, it is much clearer now. Thanks a bunch!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsGlad to help. Keep up the good work.
Steve.