Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial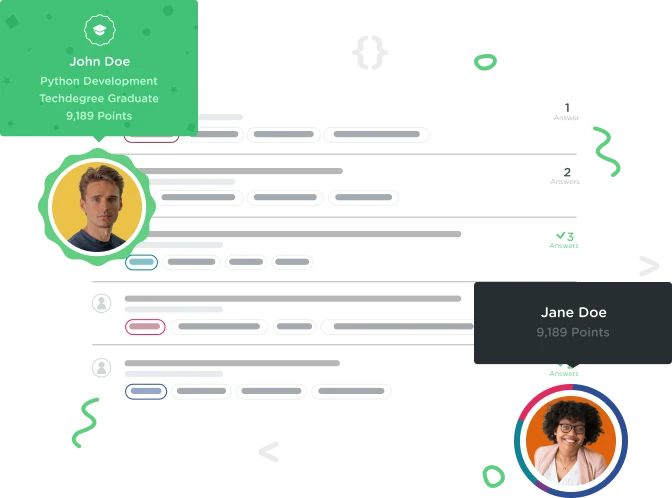

Jeffrey Hallett
Courses Plus Student 1,619 PointsCode Challenge: Overriding Methods || Need Help
I don't understand why this isn't working, the error is about converting the type "String.Type" to string or something like that.
Thanks, Jeffrey
class Person {
var firstName: String
var lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
class Doctor: Person {
override init(firstName: String, lastName: String){
super.init(firstName: String, lastName: String)
self.firstName = "Dr."
self.lastName = lastName
}
}
let someDoctor = Doctor(firstName: "Jeffrey", lastName: "Hallett")
1 Answer
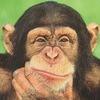
mrtroy
8,069 PointsIn your Doctor class you are overriding the firstName and lastName hence the override init line.
Then you need to pass the values in from the super class. As an example you can look at the LaserTower class in the video starting about the 2 minute mark. You will see this:
override init(x: Int, y: Int) {
super.init(x: x, y: y)
self.range = 100
self.strength = 100
}
Notice the syntax in the super.init line. It looks a little strange at first but you should be able to figure it out from there.
Happy Coding!
Jeffrey Hallett
Courses Plus Student 1,619 PointsJeffrey Hallett
Courses Plus Student 1,619 PointsThanks!