Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial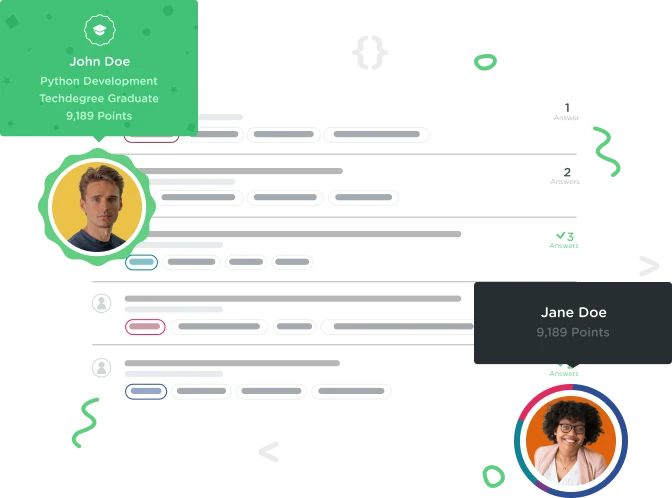
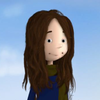
Maximiliane Quel
Courses Plus Student 55,489 PointsCode Challenge - Part 2 using split and join
Hi,
I'm not sure I understand why the following code isn't working. I have seen the question solved using placeholders for X and Y and using the format method, but I thought if I split the sentence, replace X and Y and join it that should give me the same result?
Here is the original question: Now, make a function named summarize that also takes a list. It should return the string "The sum of X is Y.", replacing "X" with the string version of the list and "Y" with the sum total of the list.
And here is my code:
def add_list(list_1):
sum = 0
for item in list_1:
sum = sum + item
return sum
def summarize(list_1):
sentence = "The sum of X is Y"
word_list = sentence.split()
word_list[3] = list_1
word_list[5] = add_list(list_1)
sentence = ' '.join(word_list)
return sentence
4 Answers

nvcxnvkldsjaklfds
Courses Plus Student 5,041 PointsThe reason is fullstop was part of word_list[5]. When you assign str(add_list(list)) to word_list[5] the value word_list[5] holds was replaced by the str(add_list(list)) value.
Please try the below code snippet.
def add_list(list_1):
sum = 0
for item in list_1:
sum = sum + item
return sum
def summarize(list_1):
sentence = "The sum of X is Y"
word_list = sentence.split()
word_list[3] = str(list_1)
word_list[5] = str(add_list(list_1))
sentence = ' '.join(word_list)
return sentence+'.'
I hope it helps.
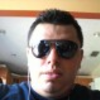
Eddie Flores
9,110 PointsI was able to get it done like this:
def add_list(items_list):
total = 0
for item in items_list:
total = total + item
return total
def summarize(items_list):
list = str(items_list)
total = 0
for item in items_list:
total = total + item
return "The sum of {} is {}.".format(list,total)
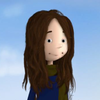
Maximiliane Quel
Courses Plus Student 55,489 PointsThank you for your answer. I realise that this is a valid solution to the problem that other people have taken.
In my question above, the point was to think the approach through to a conclusion, i.e. using the split and join instead of format method, to see how that can be achieved. A proof of concept if you will ( even though it ultimately turns out not to be the most elegant of approaches). :0)

nvcxnvkldsjaklfds
Courses Plus Student 5,041 PointsBecause list_1 is a list. You can't use join() with nested list. join() function expects collection of strings in an iterable. But when it sees there is a list inside a list then it throws error.
word_list[3] = list_1
The above code puts list in word_list[3] index. join() function only merge strings together. When it see list inside another list it throws error.
Try code below. It works fine. I did change the type of list to string using str() function.
def add_list(list_1):
sum = 0
for item in list_1:
sum = sum + item
return sum
def summarize(list_1):
sentence = "The sum of X is Y"
word_list = sentence.split()
word_list[3] = str(list_1)
word_list[5] = add_list(list_1)
sentence = ' '.join(word_list)
return sentence
I hope it helps
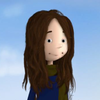
Maximiliane Quel
Courses Plus Student 55,489 Pointsah that makes sense. thanks!

nvcxnvkldsjaklfds
Courses Plus Student 5,041 PointsWelcome Maximiliane Quel I really appreciate your thinking to solve a problem in different way.
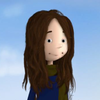
Maximiliane Quel
Courses Plus Student 55,489 PointsSo I tried the solution also converting the add_list(list_1) into a string:
def add_list(list):
total = 0
for item in list:
total = total + item
return total
def summarize(list):
sentence = "The sum of X is Y."
word_list = sentence.split()
word_list[3] = str(list)
word_list[5] = str(add_list(list))
sentence = ' '.join(word_list)
return sentence
and I get the following error message: Bummer! summarize returned the wrong output. Got 'The sum of [1, 2, 3] is 6' instead of 'The sum of [1, 2, 3] is 6.'.
not sure what happens to my fullstop??
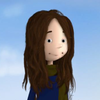
Maximiliane Quel
Courses Plus Student 55,489 PointsI guess I would have to concatenate that one on since it is not part of the answer. Or leave room in the sentence. I guess it is not exactly a great solution ...

Paul Shields
10,066 PointsIt's because the 'Y.' is one item on the list and gets replaced with the 6.
.split is separating on the whitespace, and there is no whitespace between 'Y' and '.'.