Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial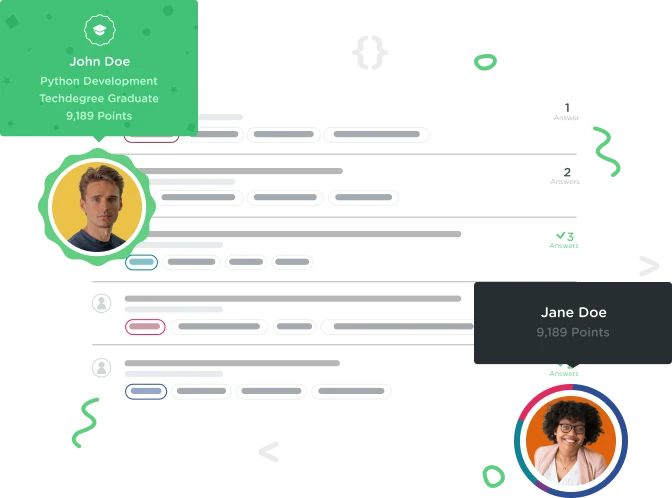

Aaron HARPT
19,845 Pointscode challenge problem
I am having trouble with the following code challenge.
"Add a Boolean value to the condition in this conditional statement. Use a boolean value that will result in the message "This is true" appearing in an alert box."
if ( ) {
alert('This is true');
} else {
alert('This is false');
}
if (true) {
alert("This is true");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
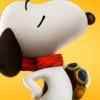
Salman Akram
Courses Plus Student 40,065 PointsHi Aaron,
Don't need to make it very complicated. You have to add just `true' in first line. Start with simple example below:
if (true) {
alert('This is true');
} else {
alert('This is false');
}
Hope that helps. :)
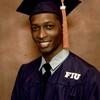
Dane Parchment
Treehouse Moderator 11,075 PointsWell based on the question you have an extra if statement in there that is not really necessary. The javascript code should look like this:
if ( ) {
alert('This is true');
} else {
alert('This is false');
}
Alright now that we have that settled, let's get to work on the problem. We need to add a boolean value that makes this if block return the statement this is true. Well we need to have an understanding of what boolean values are. Well boolean values can only be two things: true or false. So we need to make sure that whatever is in the if block is true or false, because we want to return the alert this is true, we need to make sure that whatever the condition is in the if block is true. Of course we can simply type in the keyword true into the if conditional and that will instantly return this is true.
if (true) {
alert('This is true');
} else {
alert('This is false');
}
Another thing that we can do is add an expression within the if statement that will always return true. Remember the logical operators: &&, ||, !, ==, ===, <, >, <=, >=, <==, >==. Well we can use these to create a statement that will always be true. So for example:
if (5 > 4) {
alert('This is true');
} else {
alert('This is false');
}
Or
if (1 == 1) {
alert('This is true');
} else {
alert('This is false');
}
Or
if (10 <= 11) {
alert('This is true');
} else {
alert('This is false');
}
Or
if (5 > 4 || 2 < 3) {
alert('This is true');
} else {
alert('This is false');
}
You get the point. Hopefully this helps!