Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial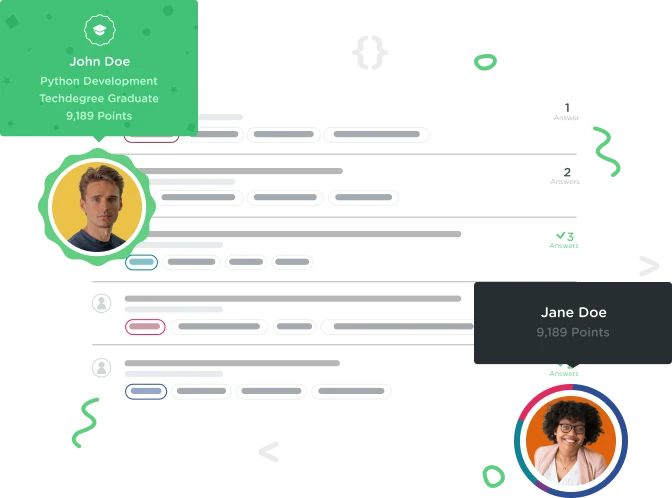

Aaron HARPT
19,845 PointsCode challenge problem
I am having trouble with this code challenge. I believe I am running a endless loop but am unsure how to fix it.
The code in app.js just opens a prompt, asks for a password, and writes to the document. Something is missing. Add a while loop, so that a prompt keeps appearing until the user types "sesame" into the prompt.
var secret = prompt("What is the secret password?");
while (prompt !== "sesame") {
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
2 Answers
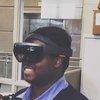
Guled Ahmed
12,806 PointsWhat you want to do is make a way for the user to exit the loop. What you have essentially done here is told the while loop to keeping looping unless "prompt" is equal to "sesame". I'll point out a few things that are missing. You stored your prompt in a variable so I suggest using that variable throughout the program. You named this variable "secret" so in our while loop we will change it from evaluating "prompt" to evaluating "secret". To ask the user continuously to verify the password you also need to place the prompt inside of the loop itself.
var secret = prompt("What is the secret password?");
while (secret !== "sesame") {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
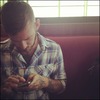
Erik McClintock
45,783 PointsAaron,
In your while condition, try changing "prompt" to "secret", like this:
while(secret !== "sesame") {
}
Remember, you are storing the value of the user's response in the variable "secret", so that's what you want to check the value of in your while condition.
Of course, you also need to write the rest of the logic for the challenge inside the while loop, but we want the condition correct first!
Erik
Erik McClintock
45,783 PointsErik McClintock
45,783 PointsGuled,
In this case, using the while loop as we have, the if statement within is unnecessary. This loop will exit itself once it's found an appropriate match for its condition, which will be once the user finally enters "sesame" in the prompt.
Open up your favorite developer tools and paste in your code, but remove your interior if statement, and test it out to see this is effect!
Erik
Guled Ahmed
12,806 PointsGuled Ahmed
12,806 PointsI caught my mistake. Thank you for the insight Erik.