Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial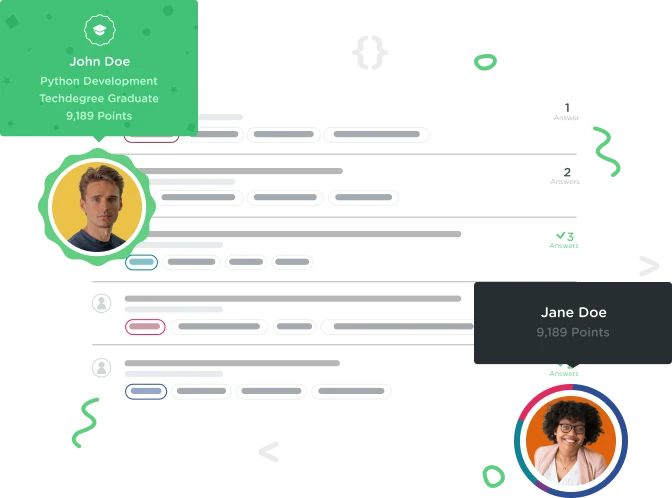

Warren Harding
1,942 PointsCode Challenge Problem - Object Oriented Swift 3 - Classes with Custom Type
Hi there, the task is as follows:
In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness.
All seems well until the last line of code where I create the instance and assign it to the constant called someBusiness.
I've looked at past examples and other answers to the same question, but I just can't figure out what I'm doing wrong. I've even copied in answers previously given on the forum.
Help is greatly appreciated.
Thanks Warren
struct Location {
let latitude: Double
let longitude: Double
class Business {
let name: String
let location: Location
init(name: String, latitude: Double, longitude: Double) {
self.name = name
self.location = Location(latitude: latitude, longitude: longitude)
}
}
}
let someBusiness = Business(name: "My Business", location: Location(latitude: 1.2, longitude: 2.1))
5 Answers

Caleb Abraham
15,158 PointsSince you're creating an instance of Location when you create someBusiness, it's not needed in your Business initializers.
init(name: String, location: Location) {
self.name = name
self.location = location
}
}

Warren Harding
1,942 PointsHi Caleb,
Thanks for the response. I'm afraid that's still giving me an error though. I'm getting the same error as last time, but this time I'm getting an additional error which says:
swift_lint.swift:18:19: error: assigning a property to itself self.location = location ~~~~~~~~~~~~~ ^ ~~~~~~~~ swift_lint.swift:21:2: error: extraneous '}' at top level } ^
In my original code, the compiler worked fine up to the 'let someBusiness' statement. If I left that out, it compiled just fine. It was adding that statement that caused the problem.
Thanks a lot. Warren
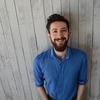
Matt Skelton
4,548 PointsHey Warren,
You've also declared your class within the same scope as your struct! If you separate it out and make the changes that Caleb suggested, you should be good to go.
Hope that helps, let me know if it's still causing problems!

alexander88
10,824 PointsI had a semi-random question in regards to scope: are we not able to declare the struct within the scope of the class, since we're only using it within that class anyways? When I originally tried this challenge, it was causing problems:
'''class Business { struct Location { let latitude: Double let longitude: Double } let name: String let location: Location
init (name: String, location: Location){
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "AppleShack", location: Location(latitude: 2.3, longitude: 2.3))'''
Should the format be different because it is within a class, or is it just not doable? I take it Classes and Structs must be created outside of the scope of of each other before being used within one another. Random question but just curious. Already fixed up my code thanks to Treehouses great community =)

Warren Harding
1,942 PointsThanks Matt... That has worked now. I had actually thought the class needed to be inside the struct, but I see now the error of my ways. I think a bit more study is required.
Thanks again for your help. Warren
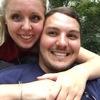
Nicholas Richardson
4,867 PointsFull answer
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "Apple", location: Location(latitude: 92.0, longitude: 93.0))