Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial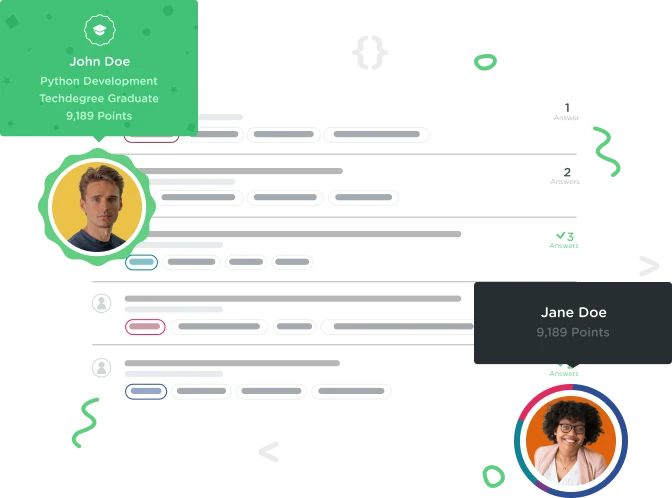

Aaron HARPT
19,845 PointsCode challenge question
I am having trouble with the following code challenge:
Complete the implementation of the merge method in utilities.js file. You should be able to pass in a string with placeholders with percent signs (%) surrounding them. The second parameter should be an object with values to be inserted in to the placeholders. Look at index.js to see how it should work.
var utilities = require("./utilities");
var mailValues = {};
mailValues.first_name = "Janet";
var emailTemplate = "Hi %first_name%! Thanks for completing this code challenge :)";
var mergedContent = utilities.merge(emailTemplate, mailValues);
//mergedContent === "Hi Janet! Thanks for completing this code challenge :)";
function merge(content, values) {
return content;
}
module.exports.merge = merge;
5 Answers

Yawar Jamal
606 PointsHey Aaron, nice that youworked upon it . So you are very close except that in your replace function instead of using "{" "}" you are suppose to use "%" + key + "%"
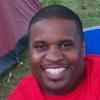
Michael Walker
45,428 PointsHi Aaron,
Your answer is very similar to mine in that we both followed Andrew's example in the lesson by cycling over the keys using a loop. The only difference here is the test gives you further instructions of using percent signs instead of brackets. You do seem to have the answer already, just in the wrong format so I'll paste my answer here:
function merge(content, values) {
for (var key in values) {
content = content.replace("%" + key + "%", values[key]);
return content;
}
module.exports.merge = merge;
Hope it helps!
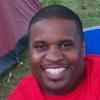
Michael Walker
45,428 Pointsfunction merge(content, values) {
for (var key in values) {
content = content.replace("%" + key + "%", values[key]);
return content;
}
module.exports.merge = merge;
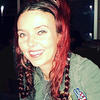
Juliette Tworsey
Front End Web Development Techdegree Graduate 32,425 PointsIt took some trial and error, but this is what inevitably worked for me:
function merge(content, values) { for (var key in values) { content = content.replace("%" + key + "%", values[key]); } return content;
}
module.exports.merge = merge;
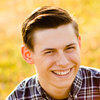
Caleb Kleveter
Treehouse Moderator 37,862 PointsHi Aaron :^)
Not sure what your ideas are to solve this, but could you use an if loop? Not much of a hint, just trying to help.

Yawar Jamal
606 PointsHi Aaron,
So the problem is complete the merge function so that it replaces the placeholders with the correct value (although merge "name for function" doesn't sound right with the behaviour and purpose of the function), anyway let me give you a hint: try using String replace function which takes two parameter "".replace(string to be replaced, string to replace for) Also a traversing over the values content will be useful as well.
Hope that helps. Now try it out :)

Aaron HARPT
19,845 PointsMr. Jamal- Attached is my current version of the challenge, although I still cannot figure it out. Any more help that you could give me would be greatly appreciated.
index.js
var utilities = require("./utilities");
var mailValues = {};
mailValues.first_name = "Janet";
var emailTemplate = "Hi %first_name%! Thanks for completing this code challenge :)";
var mergedContent = utilities.merge(emailTemplate, mailValues);
//mergedContent === "Hi Janet! Thanks for completing this code challenge :)";
utilitites.js
function merge(content, values) {
for(var key in values) {
content = content.replace("{{"+ key +"}}", values[key]);
}
return content;
}
module.exports.merge = merge;