Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial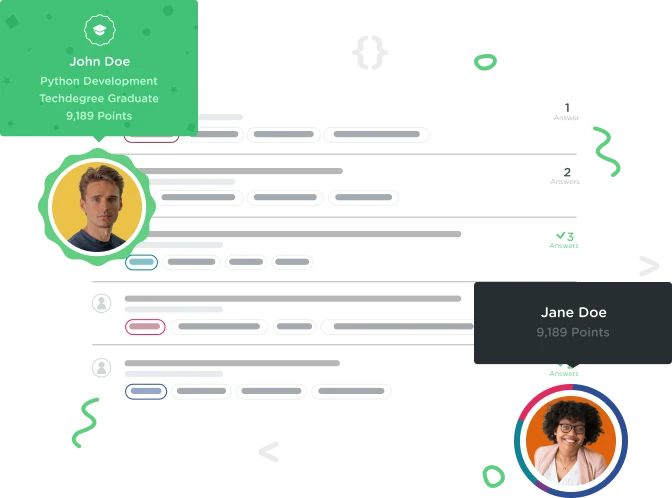

qlpxjevhuv
10,503 PointsCode Challenge: Returning Minutes
I'm not sure why this isn't working.
import datetime
def minutes(dt1, dt2):
mints1 = dt1.minute
mints2 = dt2.minute
mints = round(dt2 - dt1)
return mints
2 Answers
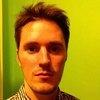
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsA couple things:
Taking just the minute property out of a datetime object is going to cause problems here. Let's say my datetime object it was (2009, 1, 6, 15, 8, 24, 78915) - it's just going to return '8', it's going to ignore all the other stuff.
When you calculated
mints = round(dt2 - dt1)
you're using the original datetime arguments that were passed in, and you're not using the mints1 and mints2 variables that you just created.
Here's my solution. When you subtract one datetime object from another datetime object, you get a timedelta object - delta being "change", so this is an object representing a change in time. Then you can call the total_seconds() method on a timedelta object, and you can divide that number by 60 to get minutes, and then round that number.
def minutes(dt1, dt2):
change = dt2 - dt1
seconds = change.total_seconds()
minutes = seconds / 60
return round(minutes)

vikram choudermet
5,701 Pointsimport datetime def minutes(old, new): old = datetime.datetime.now() new = datetime.datetime.now() - old return (round(new.seconds/60))

vikram choudermet
5,701 Pointswhy this isn't working