Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial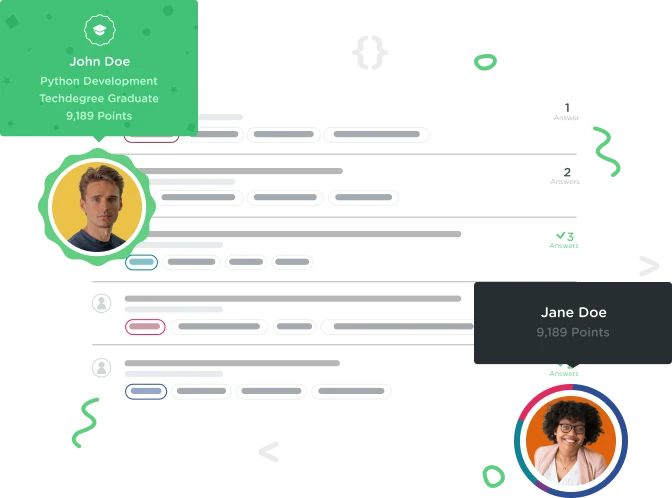
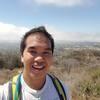
Ben Junya
12,365 PointsCode Challenge: Using intents to Display and Share... Task 3 help
I'm having a problem converting the Uri to a string. Here's my code:
import android.os.Bundle; import android.content.Intent; import android.net.Uri; import android.webkit.WebView;
public class WebViewActivity extends Activity {
protected String mUrl;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_web_view);
WebView webView = (WebView) findViewById(R.id.webView1);
Intent intent = getIntent();
Uri mUrl = intent.getData();
mUrl.toString();
}
}
I know in the video we were told to convert it to a string wiehn we loaded the Url into the webview with
webView.loadUrl(blogUri.toString());
But the challenge says that I need to store mUrl as a string, and I thought the .toString() method would have taken care of that, but I keep encountering this problem. What's going on?
3 Answers

Rohit Sriram
4,235 PointsmUrl = mUrl.toString(); probably doesn't work because of the fact that you said it was a Uri.
Hope this helps

Rohit Sriram
4,235 PointsFirst of all I noticed something weird. If mUrl is a string then how did you say it was a Uri later "Uri mUrl = intent.getData();". This may be the cause of you r problems as they are two different types, and you cannot convert them. To make mUrl a string(ignoring the earlier quirk, you should probably use a different member variable.) just use String<variable name> = <whatever you want to convert>.toString();
*Stuff in angle brackets can be substituted to be whatever you want

Rohit Sriram
4,235 PointsI think when it says that you have to store it as a string, you need to add mUrl.toString() to a string variable. Otherwise, the fact that you converted it doesn't make much difference, as there is no record left that it was converted into a string. In other words, it would have no effect on your app. At least, this is what I understand. Try adding it to a string variable and see if it works. i think that is how I did it.
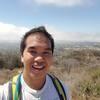
Ben Junya
12,365 PointsHey Rohit, how would I add it to a string variable? It's still not working when I try to declare a new string. There's already a member class variable declared (protected String mUrl) in the top.
I tried:
mUrl = mUrl.toString();
and it gives me a compiler error. This is really frustrating. What's going on?
Ben Junya
12,365 PointsBen Junya
12,365 PointsHey Rohit, thanks for your help. You enlightened me a little bit by showing me what went wrong. Plus, a full night of rest helped clear my head :)
I was setting declaring a mUrl as a String AND a Uri. That's where it went wrong. I needed to declare a new Uri and convert it to a String with toString();
Here's what I fixed:
protected String mUrl;
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_web_view);
WebView webView = (WebView) findViewById(R.id.webView1); Intent intent = getIntent(); Uri data = intent.getData(); mUrl = data.toString();
}
That solved it. Thanks Rohit!