Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial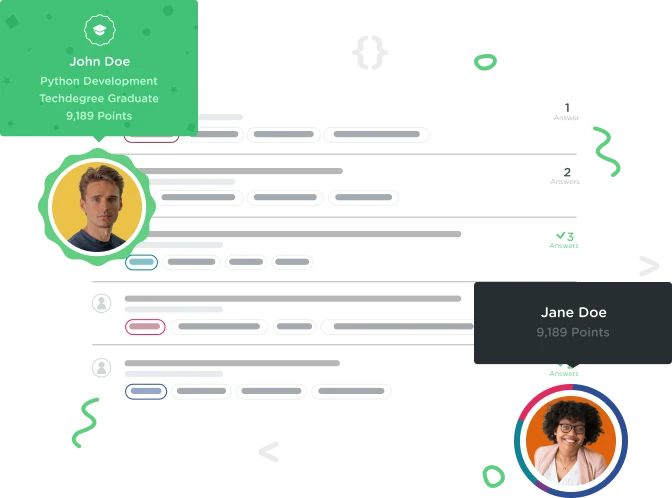
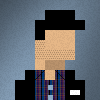
Adeeb Syed
3,275 PointsCode Challenge Variables - Null and Undefined
I'm having a little trouble understanding this code challenge.
Question: The identical() method is being called but the variables are not identical. See if you can fix the code.
Code given:
<script>
var myUndefinedVariable;
var myNullVariable = null;
if(myNullVariable == myUndefinedVariable) {
identical();
}
</script>
My problem:
During the Null and Undefined video, we were taught that the operator ==
considers null and undefined to be the same thing. So in the code given, that "if" statement is true so the method identical()
should be called, right?
However, the correct answer is using the operator ===
. ===
is the strict operator as we were taught in the lesson prior to the challenge and does not consider null and undefined to be the same thing, right?
Summary: Why is "==" wrong and "===" right?
5 Answers

James Barnett
39,199 PointsYou are confusing equal and identity. null
& undefined
are equal due to type coercion but not identical.
===
basically does the same thing as ==
but without any type conversion.
Read more about difference between equal & identity equal in this article

Eduardo Garcia
4,571 PointsI have a feeling that the operators were switched in the question, because null and undefined have the same value but are not the same type, so double equal should be the correct answer and the incorrect code should of had triple equal to make it "wrong".

Kaitlyn Dryer
18,581 PointsExactly! I'm pretty sure it's backwards. I was trying everything to ensure they were identical when actually they already were and the question wanted them not to be.

Kaitlyn Dryer
18,581 PointsExactly! I'm pretty sure it's backwards. I was trying everything to ensure they were identical when actually they already were and the question wanted them not to be.
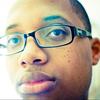
David Cole
13,136 PointsEDIT: The below is wrong, but I'll leave it here in case anyone else was similarly confused.
Yeah, I'm pretty sure the code challenge is broken. I ran this test, where I wrote things out a bit more to make sure I understood what was happening:
var myUndefinedVariable;
var myNullVariable = null;
//Check the null or undefined status of each variable
console.log("myUndefinedVariable is considered as: " + myUndefinedVariable);
console.log("myNullVariable is considered as: " + myNullVariable);
//Check whether or not the variables are considered as equals
if(myNullVariable == myUndefinedVariable) {
console.log("These are considered as equals!");
} else {
console.log("These are NOT considered as equals!")
}
It looks like using == results in the variables being equal, while using === results in them not being equal.
David Cole
13,136 PointsDavid Cole
13,136 PointsThanks for the helpful article, James. I misunderstood the goal of the question, it seems. It makes sense after reading your comment, the article, and the question over again.
Originally, I thought the point of the exercise was to fix the code so that identical(); would run. However, it was already running based on the given code using the == operator. Hence my confusion.
It seems that the point was to make identical(); run only if the values of the variables were identical (===). Since null and undefined are not identical, the identical(); function doesn't run.