Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial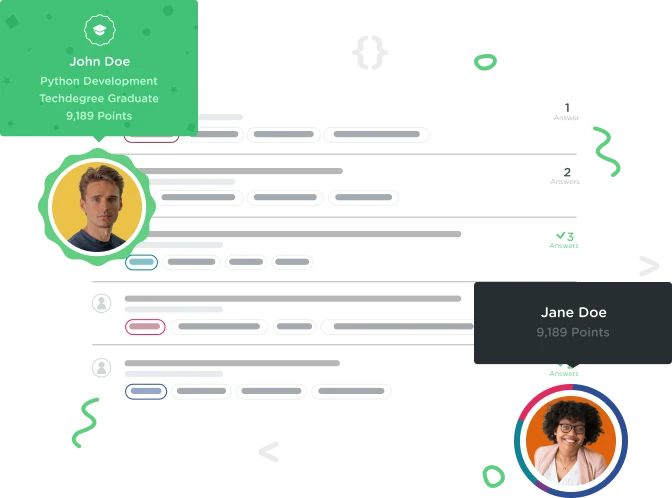
Christian McMullin
13,086 Pointscode correction on reseting stepper value not just quantity variable.
When running the app on my phone I noticed whenever I performed an action that would call the reset() function it would reset the quantity label to 1, but if I clicked up or down on the stepper the label would be right back from where it left off, so the stepper's value wasn't actually being reset. The code as of this video is only updating the quantity variable and displaying that to the label not the actual quantity stepper value. Hopefully I'm not just jumping the gun and this is fixed later in the course. I'm pretty sure I didn't miss anything in the past, but even if I did this way works properly.
So to actually update the value of the stepper this is what I did. first I went to the Main.Storyboard and control drag clicked the stepper to the top of the viewController.swift like so
class ViewController: UIViewController, UICollectionViewDataSource, UICollectionViewDelegate {
@IBOutlet weak var collectionView: UICollectionView!
@IBOutlet weak var totalLabel: UILabel!
@IBOutlet weak var balanceLabel: UILabel!
@IBOutlet weak var quantityLabel: UILabel!
//adding this outlet as so below.
@IBOutlet weak var quantity: UIStepper
//delete the var quantity
then anywhere that was calling the variable quantity I put quantity.value instead like below.
@IBAction func purchase() {
if let currentSelection = currentSelection {
do {
try vendingMachine.vend(currentSelection, quantity: quantity.value)
updateBalanceLabel()
} catch VendingMachineError.OutOfStock {
showAlert()
} catch {
}
} else {
// FIXME: Alert user to no selection
}
}
@IBAction func updateQuantity(sender: UIStepper) {
quantity.value = sender.value
updateTotalPriceLabel()
updateQuantityLabel()
}
func updateTotalPriceLabel () {
if let currentSelection = currentSelection, let item = vendingMachine.itemForCurrentSelection(currentSelection) {
totalLabel.text = "$\(item.price * quantity.value)"
}
}
func updateQuantityLabel() {
quantityLabel.text = "\(quantity.value)"
}
func updateBalanceLabel() {
balanceLabel.text = "$\(vendingMachine.amountDeposited)"
}
func reset() {
quantity.value = 1
updateQuantityLabel()
updateTotalPriceLabel()
}
This way when the reset function is called the actual value of the stepper is changed and not the variable quantity and displaying that to the quantity label. Instead the stepper value is being displayed.