Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial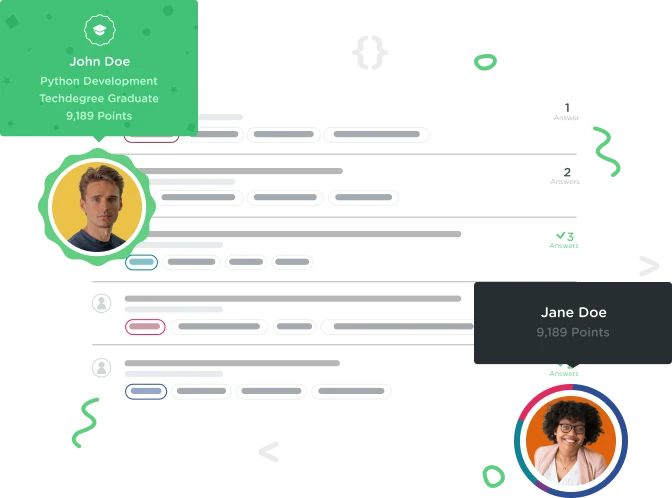

John Crowell
2,856 PointsCode does not work if user answers "Too much" to the first question "What is the total?"
A message in parentheses ("could not convert string to float: 'Too much'") appears in addition to the message "Oh no! That's not a valid value. Try again..." How would I fix this so that only the message "Oh no! That's not a valid value. Try again..." appears?
Here is the code that I used: import math
def split_check(total,number_of_people):
if number_of_people <= 1:
raise ValueError("More than 1 person is required to split the check")
return math.ceil(total/number_of_people)
try:
total_due = float(input("What is the total? "))
number_of_people = int(input("How many people? "))
amount_due = split_check(total_due, number_of_people)
except ValueError as err:
print("Oh no! That's not a valid value. Try again...")
print("({})".format(err))
else:
print("Each person owes ${}".format(amount_due))
If the "user" inputs "Too much" in answer to the question "What is the total?", then the following is returned:
Oh no! That's not a valid value. Try again...
(could not convert string to float: 'Too much')
1 Answer

Steven Tagawa
Python Development Techdegree Graduate 14,438 PointsYou are right—you found a bug in Craig's program! "Fixing" the second possible exception sorta kinda breaks the fix to the first. That can happen quite a bit, especially in complicated scripts.
You asked how to fix it so that just "Oh no! That's not a valid value. Try again..." appears. That's easy—just take out the print("{}".format(err))
line. Then, only the "Oh no!" line will print, no matter where the exception is raised. And since the message makes sense for both an invalid amount and an invalid number of people, it will work just fine. You could take off the as err
bit, too, since you're not using it.
If you need or want a different message to print depending on whether the user put in a bad amount or an invalid number of people, you could test to see what is in the err
variable. Note that err
isn't exactly a string, but it can be converted to one. I don't remember if Craig has gotten to the in
keyword yet, but here it just means "can you find one string inside another string?" For example, if "get" in "together":
is True
, and if "sunny" in "rainbow":
is False
.
except ValueError as err:
if "could not convert string to float" in str(err)
print("Oh no! That's not a valid value. Try again...")
else:
print("{}".format(err))

John Crowell
2,856 PointsThanks Steven! I greatly appreciate it!
boi
14,242 Pointsboi
14,242 PointsCan you post your code here, so I can take a look at it. If you don't know how to post code go here.