Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial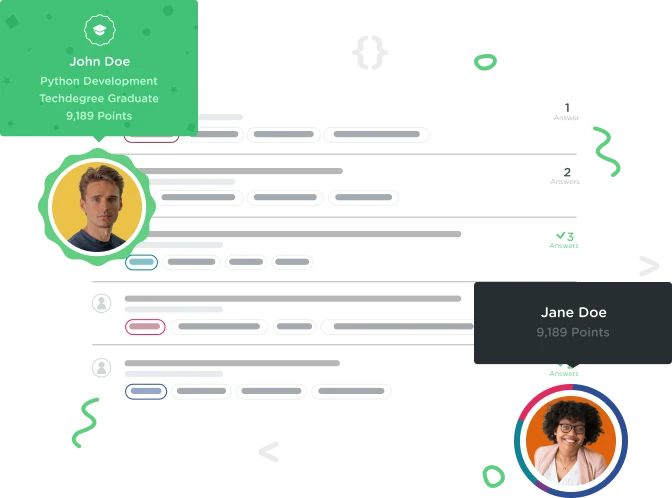
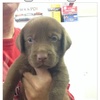
Matthew Clark
7,255 PointsCode Error
I am not sure what the issue is with my code here.
function arrayCounter(arr) { if (typeof arr === Array) { return arr.length; } else (typeof arr === 'string' || arr === undefined || arr === number) { return 0; }
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter(arr) {
if (typeof arr === Array) {
return arr.length;
}
else (typeof arr === 'string' || arr === undefined || arr === number) {
return 0;
}
</script>
</body>
</html>
2 Answers
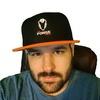
Johnathan Shoff
6,877 PointsI might be mistaken, but where are you declaring the array variable that is being passed into the function? I cannot tell what the error is based on what is provided but I would have to assume that it has something to do with the variable you are passing whether it is an array or not. Please provide me a little more info based off what I said, and I might be able to assist you.
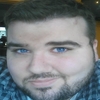
Marcus Parsons
15,719 PointsThere are a few problems with your code, but the problem is with how you are checking for an array, as well. Let me explain:
You need an ending curly brace for your function, but this is only a parse error, not the real root of your problem.
An array is a special type of object. When you use the "typeof" function on an array, it will return "object" not "array". So, you can't use "typeof" to test to see if it is an array. You also can't use the else statement in that way. It would have to be an else-if, but hint: you don't need to use an else-if in this case.
What you can do is check to see if arr is a string, number, or undefined. Remember that the typeof function returns values as strings so when you check them with the if-statement, each individual statement needs to have "typeof" and each case needs to be wrapped in either single or double quotes. Perhaps after you have used that first if-statement to check to see if it is a string, number, or undefined, you can just say anything else is an array (or boolean, technically).
If you are still stuck after trying it some more, I have the solution and can explain it further. Shoot me an email: marcus.parsons@gmail.com. I won't post it here because there are some mods who like to edit my posts that have the solutions despite me giving vivid details about the problem.
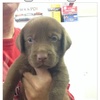
Matthew Clark
7,255 Pointsfunction arrayCounter (array) { if (typeof array === 'undefined'|| array === 'string' || array === 'number') { return 0; } { return array.length; } }
Here is the code I have now. I am still getting an error.
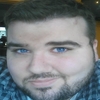
Marcus Parsons
15,719 PointsYou are so close. You are missing an else
statement in between the closing brace for the if-statement and the opening brace for return array.length;
and remember like I said that each statement in the if-statement must have the "typeof" function applied to it in order to get back the desired type.
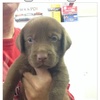
Matthew Clark
7,255 Pointsfunction arrayCounter (array) { if (typeof array === 'undefined'|| array === 'string' || array === 'number') { return 0; } else { return array.length; } }
Ok. I have this code, and I am getthing the following error.
Wrong value returned from arrayCounter(1)' it should be 0
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsI see where you are getting that, and normally you would do exactly as you said. However, this is for a challenge, and declaring an array variable doesn't solve it.