Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial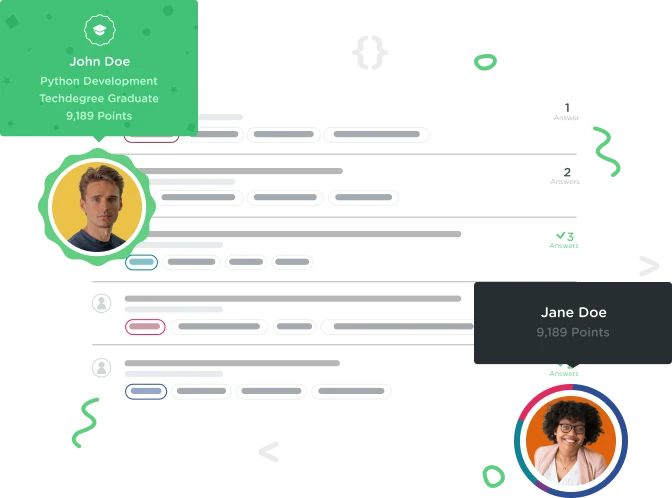
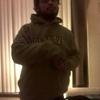
Isaac Calvo
8,882 PointsCode executes as expected except when a user gets the score 3/5 the score in decimal= 60.00000000000001%
messes everything up and the rank for scoring 0/5 gets printed.
code: /*
- ask 5 q's
- keep track of # of correct q's by user
- provide final message to player giving them a score of how many questions they got correct
- rank the player based on correct answers
- 5/5 gold crown
- 3-4/5 silver crown
- 1-2/5 bronze crown
- 0 no crown */
var userScore = 0;
var userQuestion1 = parseInt(prompt('What is 5 + 1?')); if (userQuestion1 === 6) { alert('Correct! 4 more to go!'); userScore += 1/5 } else { alert('Incorrect! Click Ok for Next question'); }
var userQuestion2 = parseInt(prompt('What is 4 + 2?')); if (userQuestion2 === 6) { alert('Correct! 3 more to go!'); userScore += 1/5 } else { alert('Incorrect! Click Ok for Next question'); }
var userQuestion3 = parseInt(prompt('What is 3 + 3?')); if (userQuestion3 === 6) { alert('Correct! 2 more to go!'); userScore += 1/5 } else { alert('Incorrect! Click Ok for Next question'); }
var userQuestion4 = parseInt(prompt('What is 2 + 4?')); if (userQuestion4 === 6) { alert('Correct! 1 more to go!'); userScore += 1/5 } else { alert('Incorrect! Click Ok for Next question'); }
var userQuestion5 = parseInt(prompt('What is 1 + 5?')); if (userQuestion5 === 6) { alert('Correct! Test Completed'); userScore += 1/5 } else { alert('Incorrect! Test Completed. Click Ok for final score'); }
if (userScore === 5/5) { alert('Congratulations! You Win the Gold Crown!'); document.write('<h3>Rank: Gold Crown</h3>'); } else if (userScore === 2/5 || userScore === 3/5) { alert('Good Job. Bronze Crown For you!'); document.write('<h3>Rank: Bronze Crown</h3>'); } else if (userScore === 4/5) { alert('Great Job. Silver Crown For you!'); document.write('<h3>Rank: Silver Crown</h3>'); } else { alert('Sorry, Keep studying. No crown for you ') document.write('<h3>Rank: Noob</h3>'); }
document.write('<h3>Final Score: ' + userScore * 100 + '% </h3>');
3 Answers
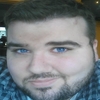
Marcus Parsons
15,719 PointsHey Isaac,
JavaScript has an issue with floating point numbers. The easiest way for you to get around this is to use an integer for the userScore, check for the integer values, then multiply by 20 at the end (because 100/5 = 20). This will eliminate any need for fractions. I also changed your parseInt's to use the base 10 system explicitly as this is recommended by the MDN when parsing integers to keep the parsing consistent across browsers.
var userScore = 0;
var userQuestion1 = parseInt(prompt('What is 5 + 1?'), 10);
if (userQuestion1 === 6) {
alert('Correct! 4 more to go!');
userScore += 1; } else { alert('Incorrect! Click Ok for Next question'); }
var userQuestion2 = parseInt(prompt('What is 4 + 2?'), 10);
if (userQuestion2 === 6) {
alert('Correct! 3 more to go!');
userScore += 1;
}
else {
alert('Incorrect! Click Ok for Next question');
}
var userQuestion3 = parseInt(prompt('What is 3 + 3?'), 10);
if (userQuestion3 === 6) {
alert('Correct! 2 more to go!');
userScore += 1;
}
else {
alert('Incorrect! Click Ok for Next question');
}
var userQuestion4 = parseInt(prompt('What is 2 + 4?'), 10);
if (userQuestion4 === 6) {
alert('Correct! 1 more to go!');
userScore += 1;
}
else {
alert('Incorrect! Click Ok for Next question');
}
var userQuestion5 = parseInt(prompt('What is 1 + 5?'), 10);
if (userQuestion5 === 6) {
alert('Correct! Test Completed');
userScore += 1;
}
else {
alert('Incorrect! Test Completed. Click Ok for final score');
}
if (userScore === 5) {
alert('Congratulations! You Win the Gold Crown!');
document.write('<p>Rank: Gold Crown</p>');
}
else if (userScore === 2 || userScore === 3) {
alert('Good Job. Bronze Crown For you!');
document.write('<p>Rank: Bronze Crown</p>');
}
else if (userScore === 4) {
alert('Great Job. Silver Crown For you!');
document.write('<p>Rank: Silver Crown</p>');
}
else {
alert('Sorry, Keep studying. No crown for you');
document.write('<p>Rank: Noob</p>');
}
document.write('<p>Final Score: ' + (userScore * 20) + '% </p>');
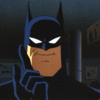
georgekiknadze
Courses Plus Student 6,307 Pointscan you copy your code block, because I think nobody can read JavaScript like this.

Mark Buckingham
5,574 PointsHi,
it is because Javascript doesn't accurately represent floating point arithmetic.
Your logic (1/5 + 1/5 + 1/5) * 100 which you would normally expect to result in 60 has accumulated some extra noise during the conversions.
You could fix the challenge code by just counting 1 to 5 or rounding the result.
Doing a Google search shows floating point numbers seem to be hot topic in Javascript, perhaps others more experienced with the language will chip in to tell how they have got round this issue.
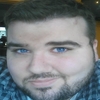
Marcus Parsons
15,719 PointsHey Mark,
I made a very powerful calculator app that gets around this issue using a round function found here at http://phpjs.org/functions/round/. This round function is the best I've seen so far because of how it deals with those floating point issues.

Mark Buckingham
5,574 PointsThanks Markus,
I thought there would be a solution, I will keep that code handy for the future.
Cool calc app by the way.
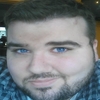
Marcus Parsons
15,719 PointsGood deal, and thanks Mark!