Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial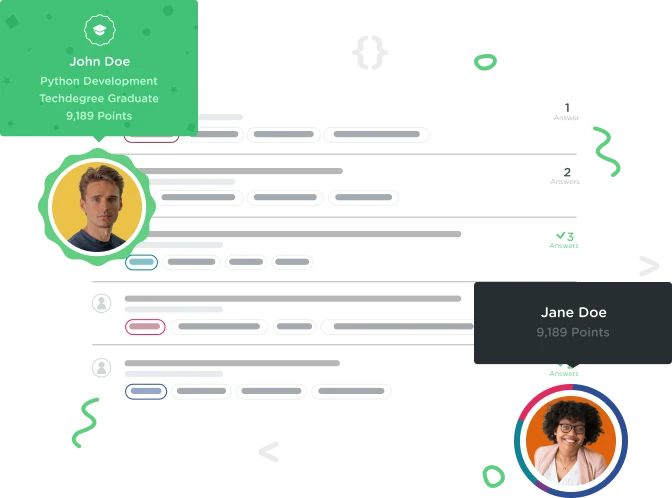
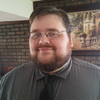
Michael Davidson
5,519 PointsCode Feedback for Weather App
Hey Guys, I built a simple little weather app thing using jQuery and AJAX. Not sure this is the right place, but was wondering if anyone would mind critiquing this little project.
Live Link : www.michaelpdavidson.net/weather.html
GitHub Code Link : https://github.com/Mpdavidson/simple-weather-openweathermap-.git
Jquery Code:
$(document).ready(function(){
//declare lat and lon
var longitude;
var latitude;
//prevent default
$('form').submit(function (evt) {
evt.preventDefault();
// define variables
var weatherAPI = "http://api.openweathermap.org/data/2.5/weather?zip=" + search + ",us&APPID=ab6945f5d48c51b058971dbb9140fa01";
var search = $('#search').val();
// display data
if(search.length < 5){
$('#lessThanFive').fadeIn(1800, function(){});
$('#greaterThanFive').hide();
$('table').hide();
$('#fiveDay').hide();
}else if(search.length > 5){
$('#greaterThanFive').fadeIn(1800, function(){});
$('#lessThanFive').hide();
$('table').hide();
$('#fiveDay').hide();
}else{
$('#lessThanFive').hide();
$('#greaterThanFive').hide();
$('#fiveDay').hide();
$('#mainWeather').fadeIn(2400, function(){});
$('#supWeather').fadeIn(3200, function(){});
$('#forecast').fadeIn(3200, function(){});
// the AJAX part
var weatherOptions = {
zip : search,
units : 'imperial'
};
function displayWeather(data) {
//declare icon variable for later use
var icon = data.weather[0].icon;
//capitalize description for output
var description = data.weather[0].description;
var capitalizedDescription = description.charAt(0).toUpperCase() + description.substring(1);
//add data to tables
$('#temperature').text(Math.round(data.main.temp) + "째 Fahrenheit");
$('#img').html('<img src="assets/img/' + icon + '.png">');
$('#description').text(capitalizedDescription);
$('#windSpeed').text( data.wind.speed.toFixed(1) + " mph");
$('#humidity').text(data.main.humidity + "%");
//give lat and lon their value
longitude = Math.round(data.coord.lon);
latitude = Math.round(data.coord.lat);
}
$.getJSON(weatherAPI, weatherOptions, displayWeather);
}
}); // end click
$('#forecast').click(function(){
//declare variable
var count = 6;
var weatherAPITwo = "http://api.openweathermap.org/data/2.5/forecast/daily?lat=" + latitude + "&lon=" + longitude + "&cnt=" + count;
//display
$('#fiveDay').fadeIn(2400, function(){});
//Ajax Part
var weatherOptionsTwo = {
lat : latitude,
lon : longitude,
cnt : count,
units : 'imperial'
};
function displayWeatherTwo(data){
//icon array
var icon = [];
for(i=1;i<data.list.length;i++){
icon.push(data.list[i].weather[0].icon);
}
console.log(icon);
//capitalize description for output
var description = [];
for(i=1;i<data.list.length;i++){
description.push(data.list[i].weather[0].description);
}
var capitalizedDescription = [];
for(i=0;i<description.length;i++){
capitalizedDescription.push(description[i].charAt(0).toUpperCase() + description[i].substring(1))
}
//Icon for day
for(i=0;i<icon.length;i++){
$('#day' + i).html('<img src="assets/img/64px/' + icon[i] + '.png">');
}
//Min / Max temps for forecast
for(i=1;i<6;i++){
$('#min' + i).text(Math.round(data.list[i].temp.min) + "째");
}
for(i=1;i<6;i++){
$('#max' + i).text(Math.round(data.list[i].temp.max) + "째");
}
//Description of weather
for(i=0;i<5;i++){
$('#des' + i).text(capitalizedDescription[i]);
}
}
$.getJSON(weatherAPITwo, weatherOptionsTwo, displayWeatherTwo);
});//end click 2
}); //end ready
For sake of room I'm not going to include the css, but its viewable in github, and view source will show the HTML.