Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial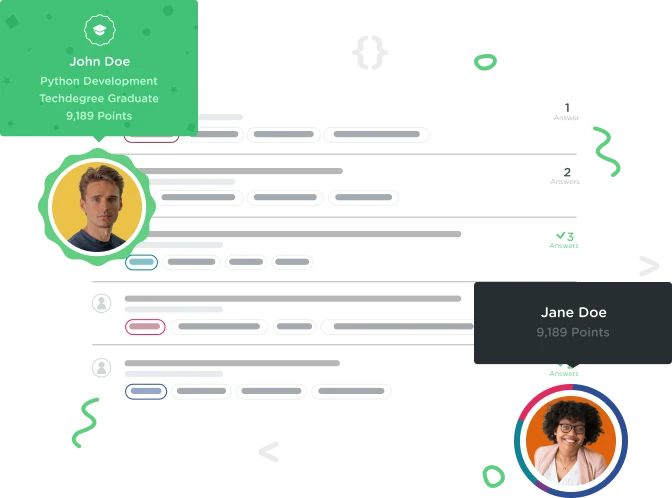

Mitchell Blankenship
12,109 PointsCode Feedback please?
this is what I have:
// Set some variables we will need var numQuestions = 6; var qAnswered = 0; var qLeft; var correct = 0;
var q1 = "YES"; var q2 = 5; var q3 = "BLUE"; var q4 = "DOGS"; var q5 = "INDIANA"; var q6 = 42;
var guess;
//Start asking questions
// question 1 guess = prompt("Yes or no, Have you ever gone fishing on a hot summers day?");
// Check the answer if ( guess.toUpperCase() === q1 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 1 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q1.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 2 guess = prompt("What is 4 + 1?");
// Check the answer if ( parseInt(guess) === q2 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 2 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q2 + " better luck next time. Only " + qLeft + " more questions"); }
// question 3 guess = prompt("What is my favorite color?");
// Check the answer if ( guess.toUpperCase() === q3 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 3 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q3.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 4 guess = prompt("Cats or Dogs?");
// Check the answer if ( guess.toUpperCase() === q4 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 4 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q4.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 5 guess = prompt("What state do I live in?");
// Check the answer if ( guess.toUpperCase() === q5 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 5 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q5.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 6 guess = prompt("How old am I?");
// Check the answer if ( parseInt(guess) === q6 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 6 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q6 + " better luck next time. Only " + qLeft + " more questions"); }
if ( correct === 6 ){ document.write("<p>Great job you got them all right! You win the Golden Cupie Doll.</p>"); } else if ( correct >= 3 && correct < 6 ) { document.write("<p>More than half right. You win a brand new toothpick.</p>"); } else if (correct > 0 && correct < 3 ) { document.write("<p>Well at least you got some of them right... You win the gift of happy thoughts.</p>"); } else { document.write("<p>You didn't get any of them right. You are a burden to society and all of your friends are dumber for having known you. You get no prizes and may God have mercy on your soul.</p>"); }
As you can see I was trying to add functionality to let users know the correct answer and keep track of how many questions they had left. I did this mainly to challenge myself a little. Also I made the prizes a little more me :P
3 Answers

Antonija Kasum
1,977 PointsSo, if your code works then there's nothing much to add to the logic since this is very simple. I would only suggest giving meaningful names to your variables because that will be very important in the future but now also because it makes your code more readable to yourself and others and it's harder to make a mistake (q1 - question1). Also look into:
- const vs. let vs. var. Using var keyword will give you problems with scope in the future so get in the habit of using let instead (or const whenever the value of the variable is going to stay the same forever).
- string interpolation
- using javascript formaters and beautifiers (eg. Beautify, Prettier) which will break your long lines of code into new lines and fix the spacings and so on - again making your code more readable to yourself and others
Other than that don't be troubled with how your code looks if it works, there is always a way to write it different or better and you will learn it with time. Just keep doing it! Good luck and happy coding!

Mitchell Blankenship
12,109 Pointssure sorry bout that
// Set some variables we will need var numQuestions = 6; var qAnswered = 0; var qLeft; var correct = 0;
var q1 = "YES";
var q2 = 5;
var q3 = "BLUE";
var q4 = "DOGS";
var q5 = "INDIANA";
var q6 = 42;
var guess;
//Start asking questions
// question 1
guess = prompt("Yes or no, Have you ever gone fishing on a hot summers day?");
// Check the answer
if ( guess.toUpperCase() === q1 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 1 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q1.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 2
guess = prompt("What is 4 + 1?");
// Check the answer
if ( parseInt(guess) === q2 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 2 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q2 + " better luck next time. Only " + qLeft + " more questions"); }
// question 3
guess = prompt("What is my favorite color?");
// Check the answer
if ( guess.toUpperCase() === q3 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 3 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q3.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 4
guess = prompt("Cats or Dogs?");
// Check the answer
if ( guess.toUpperCase() === q4 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 4 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q4.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 5
guess = prompt("What state do I live in?");
// Check the answer
if ( guess.toUpperCase() === q5 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 5 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q5.toLowerCase() + " better luck next time. Only " + qLeft + " more questions"); }
// question 6
guess = prompt("How old am I?");
// Check the answer
if ( parseInt(guess) === q6 ) { correct += 1; qAnswered += 1; qLeft = numQuestions - qAnswered; alert("Yay you got question 6 correct! only " + qLeft + " left to go."); } else { qAnswered += 1; qLeft = numQuestions - qAnswered; alert("I'm sorry the correct answer was " + q6 + " better luck next time. Only " + qLeft + " more questions"); }
if ( correct === 6 ){
document.write("<p>Great job you got them all right! You win the Golden Cupie Doll.</p>");
} else if ( correct >= 3 && correct < 6 ) {
document.write("<p>More than half right. You win a brand new toothpick.</p>");
} else if (correct > 0 && correct < 3 ) {
document.write("<p>Well at least you got some of them right... You win the gift of happy thoughts.</p>");
} else {
document.write("<p>You didn't get any of them right. You are a burden to society and all of your friends are dumber for having known you. You get no prizes and may God have mercy on your soul.</p>");
}

Mitchell Blankenship
12,109 PointsThanks I noticed const and let in another course. During this one I didn't even know they existed :P I will try to make sure to use them more in the future as I begin to understand things better.
Antonija Kasum
1,977 PointsAntonija Kasum
1,977 PointsCould you post it as code? Just put ```(backticks) before and after. If it was more readable I'm sure we could help you :)