Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial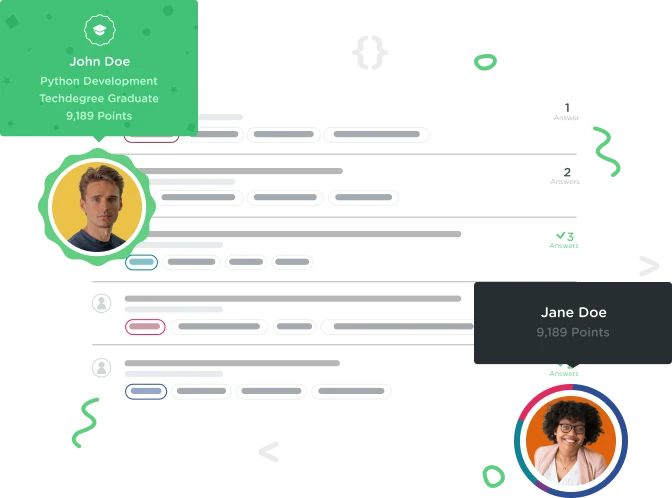

Joshua Thomas
2,807 PointsCode for the computer to guess in less tries
While not exactly a question I figured I'd share the code to people who are interested. Anyways basically what it does, when the computer guess the number, it will be told if the number is higher or lower than what it guessed. From that the computer will change the range of values accordingly, and guess again. This will repeat until it guesses correctly.
var lower = 1;
var upper = 10000;
var randomNumber = getRandomNumber(lower, upper);
var guess;lower
var attempts = 0;
function getRandomNumber(lower, upper) {
return Math.floor( Math.random() * (upper - lower + 1)) + lower;
}
while ( guess !== randomNumber ) {
guess = getRandomNumber(lower, upper );
attempts++;
if (guess > randomNumber) {
upper = guess; }
else if (guess < randomNumber) {
lower = guess;
}
}
document.write("<p> The random number was: " + randomNumber + "<p>");
document.write("<p> It took the computer " + attempts + " attempts to get it right.</p>")
If you have any questions about the code here. Feel free to ask :) This allows it to guess a number from 1 - 10000 in about 20 tries.
3 Answers

Umesh Ravji
42,386 PointsHi Joshua, that's a good way to approach the problem, but see what happens when you just pick the number that is half-way between your lower
and upper
each time.
var lower = 1;
var upper = 10000;
var randomNumber = getRandomNumber(lower, upper);
var guess;
var lower;
var attempts = 0;
function getRandomNumber(lower, upper) {
return Math.floor( Math.random() * (upper - lower + 1)) + lower;
}
function getHalf(lower, upper) {
return Math.floor((lower + upper) / 2);
}
while ( guess !== randomNumber ) {
guess = getHalf(lower, upper);
attempts++;
if (guess > randomNumber) {
upper = guess;
} else if (guess < randomNumber) {
lower = guess;
}
}
document.write("<p> The random number was: " + randomNumber + "<p>");
document.write("<p> It took the computer " + attempts + " attempts to get it right.</p>");
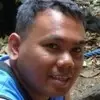
Dennis Amiel Domingo
17,813 PointsI think for Umesh Ravji's version, the computer will have a smaller chance to answer the random number at the very first try (because computer's try#1 will always be equal to 5000) . But that's just what I think, and I'm a noob lol.
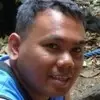
Dennis Amiel Domingo
17,813 PointsI just don't understand what the "++" in attempts is. Or maybe I haven't gotten to that part yet.

Umesh Ravji
42,386 PointsHi Dennis, attempts++
is just shorthand for attempts = attempts + 1
Tt can also be written as attempts += 1
.
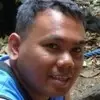
Dennis Amiel Domingo
17,813 PointsThanks Umesh!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Pointsfixed code formatting