Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial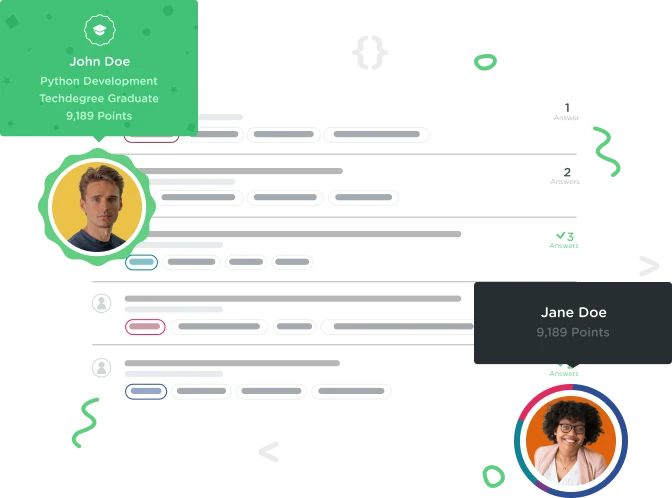
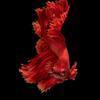
Michael Williams
Courses Plus Student 8,059 Pointscode from lesson in object oriented programming not working–swift
I typed this code out word for word while doing the lesson, but I keep getting two errors on the isInRange
method:
use of unresolved identifier 'position'
use of unresolved identifier 'range'
struct Point {
let x: Int
let y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}/// Returns the surrounding points in range of
/// the current point.
func points(inRange range: Int = 1) -> [Point] {
var results = [Point]()
let lowerBoundOfXRange = x - range
let upperBoundOfXRange = x + range
let lowerBoundOfYRange = y - range
let upperBoundOfYRange = y + range
for xCoordinate in lowerBoundOfXRange...upperBoundOfXRange {
for yCoordinate in lowerBoundOfYRange...upperBoundOfYRange {
let coordinatePoint = Point(x: xCoordinate, y: yCoordinate)
results.append(coordinatePoint)
}
}
print(results)
return results
}
}
let coordinatePoint = Point(x: 0, y: 0) //This creates an instance of the struct.
class Enemy {
var life: Int = 2
let position: Point
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func decreaseLife(by factor: Int) {
life -= factor
}
}
class Tower {
let position: Point
var range: Int = 1
var strength: Int = 1
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func fire(at enemy: Enemy) {
if isInRange(of: enemy) {
enemy.decreaseLife(by: strength)
print("Gotcha")
} else {
print("Darn! Out of range")
}
}
}
func isInRange(of enemy: Enemy) -> Bool {
let availablePositions = position.points(inRange: range)
for point in availablePositions {
if point.x == enemy.position.x && point.y == enemy.position.y {
return true
}
}
return false
}
let tower = Tower(x: 0, y: 0)
let enemy = Enemy(x: 1, y: 1)
tower.fire(at: enemy)
2 Answers
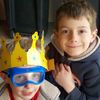
Chris Stromberg
Courses Plus Student 13,389 PointsYou have to include the isInRange function within your tower class!
import Foundation
struct Point { let x: Int
let y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}/// Returns the surrounding points in range of
/// the current point.
func points(inRange range: Int = 1) -> [Point] {
var results = [Point]()
let lowerBoundOfXRange = x - range
let upperBoundOfXRange = x + range
let lowerBoundOfYRange = y - range
let upperBoundOfYRange = y + range
for xCoordinate in lowerBoundOfXRange...upperBoundOfXRange {
for yCoordinate in lowerBoundOfYRange...upperBoundOfYRange {
let coordinatePoint = Point(x: xCoordinate, y: yCoordinate)
results.append(coordinatePoint)
}
}
print(results)
return results
}
}
let coordinatePoint = Point(x: 0, y: 0) //This creates an instance of the struct.
class Enemy {
var life: Int = 2
let position: Point
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func decreaseLife(by factor: Int) {
life -= factor
}
}
class Tower {
let position: Point
var range: Int = 1
var strength: Int = 1
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func fire(at enemy: Enemy) {
if isInRange(of: enemy) {
enemy.decreaseLife(by: strength)
print("Gotcha")
} else {
print("Darn! Out of range")
}
}
func isInRange(of enemy: Enemy) -> Bool {
let availablePositions = position.points(inRange: range)
for point in availablePositions {
if point.x == enemy.position.x && point.y == enemy.position.y {
return true
}
}
return false
}
}
let tower = Tower(x: 0, y: 0)
let enemy = Enemy(x: 1, y: 1)
tower.fire(at: enemy)
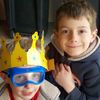
Chris Stromberg
Courses Plus Student 13,389 PointsAlso to add code formatted for swift do this....
Wrap your code with 3 backticks (```) on the line before and after. If you specify the language after the first set of backticks, that'll help us with syntax highlighting.
```swift Your code here.... ```
Michael Williams
Courses Plus Student 8,059 PointsMichael Williams
Courses Plus Student 8,059 PointsThanks!