Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial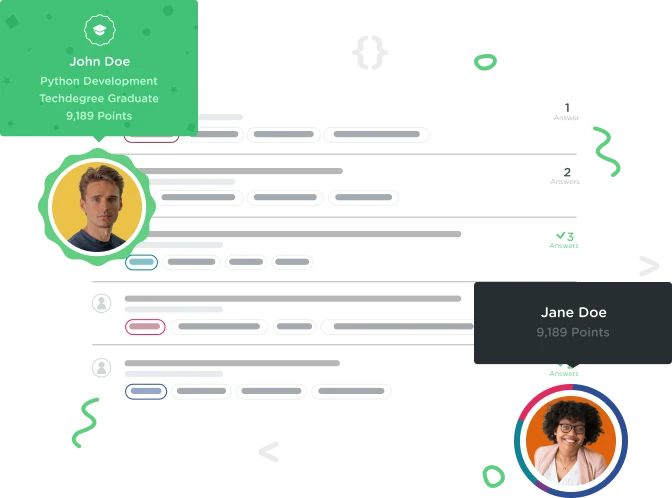

Carlos Guzman
1,300 PointsCode Improvement - Stats Challenge
Looking to improve my code. It's definitely messy and this is the first problem that gave me a good challenge.
my_dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections', 'Python Stuff', 'Python other stuff']}
def most_classes(dicts):
max_count = 0
teacher_name = None
for key in dicts:
if len(dicts[key]) > max_count:
max_count = len(dicts[key])
teacher_name = key
return teacher_name
print(most_classes(my_dict))
def num_teachers(dicts):
teacher_number = len(dicts.keys())
return teacher_number
print(num_teachers(my_dict))
def stats(dicts):
the_list = []
for key in dicts:
teacher_name = key
num_classes = len(dicts[key])
new_list = [teacher_name, num_classes]
the_list.append(new_list)
return the_list
print(stats(my_dict))
def courses(dicts):
courses = []
for key in dicts:
courses += dicts[key]
return courses
print(courses(my_dict))
1 Answer
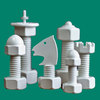
Steven Parker
230,274 PointsThese functions can be compacted into one-liners, using list comprehension, lambda, and reduce (which are probably concepts that are introduced later or in more advanced courses):
from functools import reduce
def stats(dicts):
return [ [key, len(dicts[key])] for key in dicts ]
def most_classes(dicts):
return reduce(lambda x,y: x if x[1] > y[1] else y, stats(dicts))[0]
def num_teachers(dicts):
return len(dicts.keys())
def courses(dicts):
return [ dicts[key] for key in dicts ]
Carlos Guzman
1,300 PointsCarlos Guzman
1,300 PointsThanks! I was kind of hoping to compact the code without having to import any libraries. But this at least gives me a library to look into.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsReduce used to be a built in, in older Python versions. They moved it to functools in the newer versions.
All these concepts are introduced at some point on the Python track.