Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial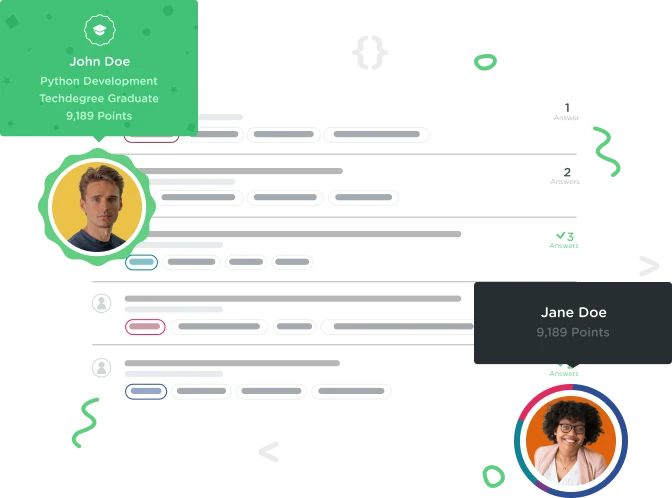

Adam Wilkins
1,677 PointsCode inside of constructor wont run?
Here is the object I'm trying to construct:
import java.util.Random;
public class Jar {
private String mItemName;
private int mMaxItemNumber;
private int mNumberOfItems;
public Jar (String itemName, int maxItemNumber){
mItemName = itemName;
mMaxItemNumber = maxItemNumber;
mNumberOfItems = fill();
System.out.printf("Number of items in jar: %d", mNumberOfItems);
}
private int fill() {
return Random.nextInt(mMaxItemNumber) + 1;
}
}
Here is the code calling the constructor:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class Prompter{
private Jar mJar;
private BufferedReader mReader;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
}
public void buildJar() {
try {
System.out.println("ADMINISTRATOR SETUP");
System.out.println("======================");
System.out.print("What type of item should be in the jar: ");
String itemName = mReader.readLine();
System.out.printf("What is the maximum amount of %s: ",
itemName);
String maxItemNumberAsString = mReader.readLine();
int maxItemNumber = Integer.parseInt(maxItemNumberAsString.trim());
mJar = new Jar (itemName, maxItemNumber);
System.out.println("1");
} catch(IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
}
}
}
1 Answer

andren
28,558 PointsI can see one issue in the fill
method:
private int fill() {
return Random.nextInt(mMaxItemNumber) + 1;
}
nextInt
is not a static method, in other words it cannot be called directly on the class it is in. You have to create an instance of the Random
class and then call the method through that instance. Like this:
private int fill() {
Random random = new Random(); // Create Random object
return random.nextInt(mMaxItemNumber) + 1; // Call nextInt on that object
}
That is the only direct error I can see.
Adam Wilkins
1,677 PointsAdam Wilkins
1,677 PointsThank you for that, I hadn't noticed it because the program never runs the .fill() method.
Does a constructor need to completely finish running before any methods (apart from static ones) inside the class can be run?