Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial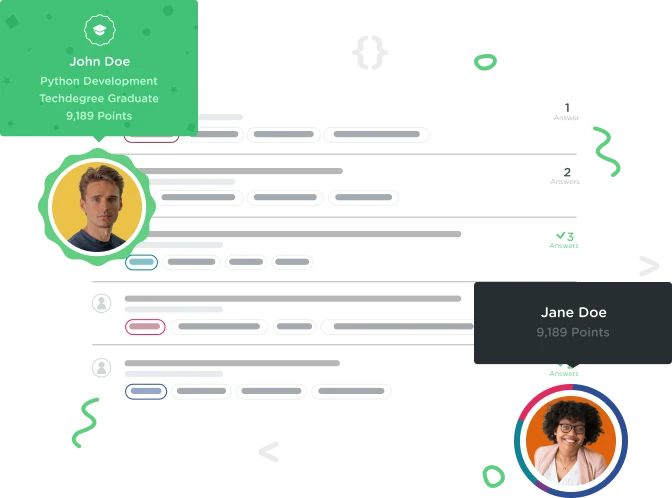
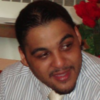
Michael Sims
8,101 PointsCode is not displaying the amount of correct answers. Help!
Hello there, I followed the solution and got it right, then went back to rebuild it, and this time everything works except; it's not giving the correct number of "correct answers"... its telling me "0", even when they are correct... can anyone help?
//Setting up the Array
var questions = [
['How many inches are in a foot?', 12],
['How many feet are in a yard?', 3],
['How many inches are in a yard?', 36]
];
//Setting up empty variables to collect data.
var correctAnswers = 0;
var question;
var answer;
var response;
var html;
function print(message) {
document.write(message);
}
//setting up the loop and defining variables
for (var i=0; i < questions.length; i += 1 ) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
if (response === answer) {
correctAnswers += 1;
}
}
html = 'Congrats, you got ' + correctAnswers + ' answers correct!';
print(html);
2 Answers
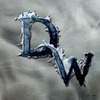
Hugo Paz
15,622 PointsHey Michael,
This happens due to the fact that the prompt method gets a string. So when you type "12" as an answer, you are storing the string '12' in the answer variable instead of the number 12.
This is due to the fact that you use the triple equals comparison (===) in your if statement. If you try to use double equals (==), your code will work as is, but it is not a good practice.
Fortunately there is an easy fix. You just use the parseInt method on your prompt so it returns an integer instead of a string.
Just add this to your code:
//setting up the loop and defining variables
for (var i=0; i < questions.length; i += 1 ) {
question = questions[i][0];
answer = questions[i][1];
response = parseInt(prompt(question));
if (response === answer) {
correctAnswers += 1;
}
}
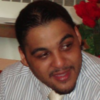
Michael Sims
8,101 PointsHey Marcus, interesting comment! I agree, and totally makes sense.
Thanks a lot!
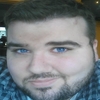
Marcus Parsons
15,719 PointsNo problem, Michael! Happy Coding! =]
Michael Sims
8,101 PointsMichael Sims
8,101 PointsHey Hugo, I literally JUST noticed this as I was re-reviewing the solution video... I changed my questions, which were string answers at first like "Michigan", to integers to match the video, but forgot the parseInt.... Thanks so much, all working now!!
Mike
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 Points+1 but with a slight modification. I wouldn't use parseInt() on the prompt, but I would instead encapsulate the number values in the array as strings. That way in case you want to add more questions, they don't have to have number based associated answers. You will also get faster executing code because of the lack of having to parse those number values. You should really only use parseInt() when you want to manipulate the number data from the input afterwards.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 Points