Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial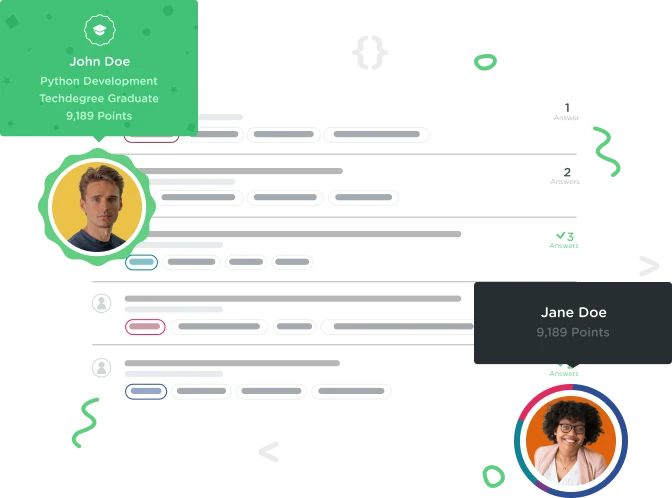

Michael North
2,561 PointsCode is not working, and I don't know why
I feel that my code should work, but for some reason it does not. Can someone please tell me what I'm doing wrong.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public EatFly(int distanceToFly)
{
bool canEatFly = distanceToFly <= tongueLength;
return canEatFly;
}
}
}
1 Answer
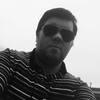
Stephen Kilgore
Java Web Development Techdegree Student 2,610 PointsThere are two problems. First, you did not list the return type of bool for your method.
second, you are accessing the wrong tongueLength variable. The one with the lowercase T does not exist in the current context you are using it. It only existed within the scope of the Frog constructor. You need to access the tongue length that is a member of the class (the one with the capital T). Remember, C# is case sensitive, so tongueLength and TongueLength are two different variable names.
here's a handy trick. I have never done the C# track, so I'm not sure you've learned it yet, but the way I would have written this is:
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int tongueLength;
public Frog(int tongueLength)
{
this.tongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
bool canEatFly = distanceToFly <= tongueLength;
return canEatFly;
}
}
}
The this
keyword points back to the class, so no confusion as to which 'tongue length' is which. The reason I don't have to use this
when I reference tongueLength in the eatFly method is because there is no other tongueLength variable to confuse it with, so it is not necessary to state this
.