Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial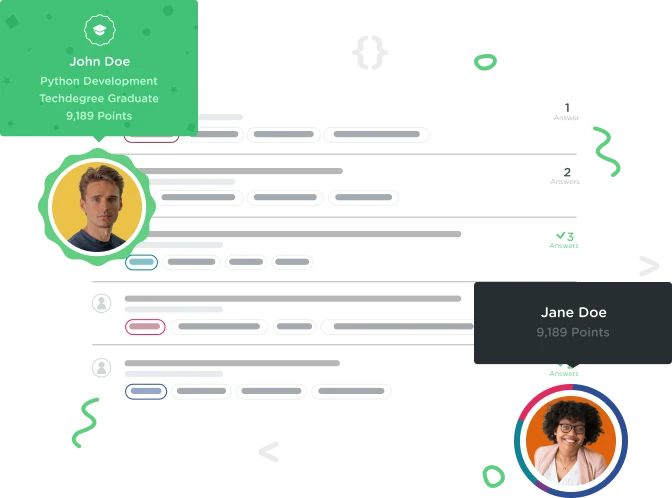

Michael Nanni
7,358 PointsCode is treating string inputs as number when it shouldn't?
Here's what should happen. The user is notified that there's an amount of tickets left. When they enter a number they are prompted to proceed with purchasing the tickets for a certain price. However it should handle an error when a string of letters is entered instead of an integer.
At one point the number_of_tickets variable is multiplied by TICKET_PRICE.
When text such as "blue" is input, it seems it's multiplying that string as if it were an integer. What's causing this?
Snapshot of my workspace: https://w.trhou.se/afpvnx9v0c
2 Answers
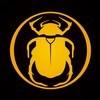
rydavim
18,814 PointsOkay, so the problem is that you're raising the error, but then the block just continues on without getting a number. So when you get to the next line you're expecting to have an integer, but you've still got the string. When the error is raised, you need to make sure you go back and ask the user for a number again.
try:
number_of_tickets = input("How many tickets would you like, {}? ".format(user_name))
# Expect a ValueError to happen and handle it appropriately...remember to test it out.
number_of_tickets = int(number_of_tickets)
except ValueError:
print("I'm sorry but that isn't a number. Try again") # Whoops, no number! But this continues on to...
total_due = int(number_of_tickets) * TICKET_PRICE # Here, where you've still got "blue" for example.
Hint: How might you go about looping back to ask for the input again when you get a ValueError
?
Let me know if you're still feeling stuck, and we can walk through a solution. Happy coding!
Update:
This is probably not the most elegant solution, but a quick and simple way to fix the issue of not asking for an alternate input on type failure is to make sure it loops until you get a number.
while True: # Yup, just keep doing this...
try:
number_of_tickets = input("How many tickets would you like, {}? ".format(user_name))
number_of_tickets = int(number_of_tickets)
break # ...until you get a number from the user, then break.
except ValueError: # Not a number? Recurse!
print("I'm sorry but that isn't a number. Try again")
# Once you get a number, the break exits back out of the block to continue with your code.
total_due = int(number_of_tickets) * TICKET_PRICE

Nina Maxberry
10,630 PointsMichael, I just ran your code using integer and a string and the code performed fine. When I used an integer i received:
There are 100 tickets remaining. You might want to purchase before they run out! What is your name, peasant? jie How many tickets would you like, jie? 56 56 tickets will be $560, please pay before they are gone Would you like to proceed? (Enter Y/N) y SOLD! There are 44 tickets remaining. You might want to purchase before they run out!
When I used a string, I received: What is your name, peasant? tdj How many tickets would you like, tdj? trew I'm sorry but that isn't a number. Try again ValueError: invalid literal for int() with base 10: 'trew'
Maybe, I don't understand your question.

Michael Nanni
7,358 PointsHi Nina Maxberry ! Thanks so much for replying. Check out the reply to rydavim's answer. That's what I was experiencing. I did see what you mentioned in an earlier test, but I wanted to handle that ValueError with a printed message rather than seeing "ValueError" being returned in the console.
Michael Nanni
7,358 PointsMichael Nanni
7,358 PointsThanks for replying rydavim ! There were a few weird things happening when I was trying to get this to work. One was the string being input getting multiplied like this:
How many tickets would you like? blue blue tickets will be $blueblueblueblueblueblueblueblueblueblue, please pay before they are gone.
And then it would continue on as you said. One thing I noticed is that it does continue until here:
proceed = input("Would you like to proceed?\n(Enter Y/N) ") if proceed.lower() == "y": print("SOLD!")
If the input is N then it will start the loop over. If the input is Y then I get a TypeError: unsupported operand type for -=: 'int' and 'str'. To me this means the code doesn't understand what the input was. I guess I need it to stop or redirect it somewhere right? Before I tried to handle the error, the loop would continue so long as an integer was entered. It would only stop once there were 0 tickets. I found out later than an else block right after the except statement resolves both issues. The thing is I really don't understand why that fixes it. Can you help explain?
rydavim
18,814 Pointsrydavim
18,814 PointsHmm, have you made any changes to your code since you posted the snapshot? Snapshots are static, so they don't update with any progress you've made.
I'm not able to reproduce the behavior you're seeing now, but if you post another snapshot I'll take a look.
I've updated the answer with one possible solution to the original problem, but I'm more than happy to explore other solutions you may be working on. 😊