Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial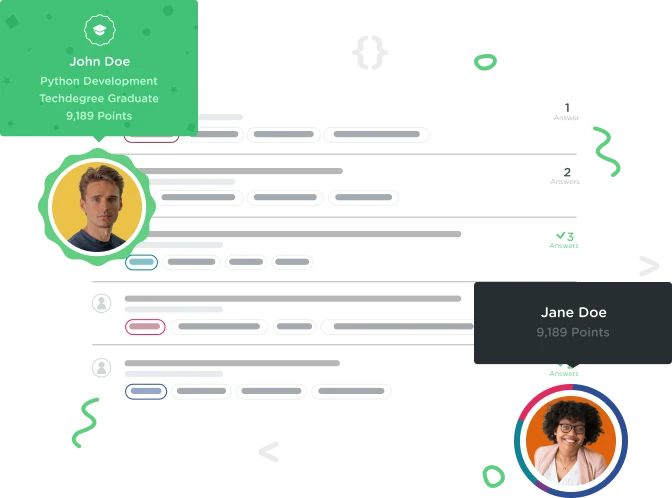

Amit Ghosh
9,314 PointsCode is working perfectly in native C# complier, not sure what is missing here..
I tried to run this on VSTF, it working as expected, however I am getting this error here.
Did you throw a exception when value is less than 0 or greater than 20...
try
{
int value = int.Parse(Console.ReadLine());
if (value<0 || value>20)
{
throw new System.Exception();
}
else
{
Console.WriteLine(string.Format("You entered {0}",value));
}
}
catch (System.Exception)
{
Console.WriteLine("Value is outside of the range");
}
2 Answers

andren
28,558 PointsThere is nothing missing, rather the opposite is the problem. When doing these challenges you should generally do exactly what the instructions tell you and nothing beyond that. Doing additional things, even if they feel like a natural part of the exercise, can often cause issues with the code checker if they are things you were not explicitly told to do.
In this case the problem is that the challenge is to throw an exception, and that's all. You are not told to catch said exception. The fact that you are catching the exception is what causes your code to fail the challenge. If you remove the try...catch block and just leave the rest of the code behind like this:
int value = int.Parse(Console.ReadLine());
if (value<0 || value>20)
{
throw new System.Exception();
}
else
{
Console.WriteLine(string.Format("You entered {0}",value));
}
Then you will be able to complete the challenge.

Amit Ghosh
9,314 PointsThanks for unblocking me, its kinda annoying when you are pointed to the same code challenge again and again when you know the answer. I understand its difficult to run throw all iterations for a code checker.
Cheers!! Amit