Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial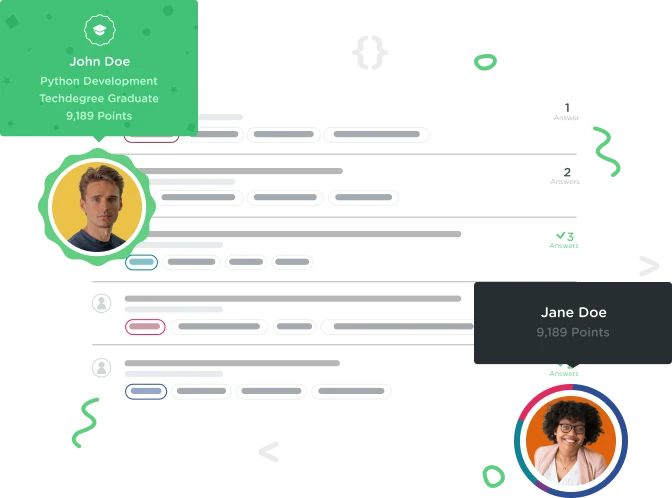

Erik Wells
5,403 PointsCode issues
Attached is the snapshot of my work in this course. I had an error pop up early and it hung around all the way through. I was wondering if anyone could see where I went wrong and show me how to fix it so I can at the least that I got the project right.
Thank You.
1 Answer
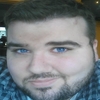
Marcus Parsons
15,719 PointsHey Erik,
A lot of the problems in your code stemmed from simple spelling errors. There were several times where you left off a "t" in "listItem" in a few functions. I included comments where I changed code, so read those over:
//Problem: User Interaction doesn't provide desired results.
//Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTaskHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//New Task List Item
var createNewTaskElement = function(taskString) {
//Create list item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); //checkbox
//label
var label = document.createElement("label");
//input (text)
var editInput = document.createElement("input"); //text
//button.edit
var editButton = document.createElement("button");
//buton.delete
var deleteButton = document.createElement("button");
//Each elements, needs modifying
checkBox.type = "checkBox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
//Each elements needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function() {
console.log("Add task...");
//Create a new list item with the text from #new-task
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to incompleteTaskHolder
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = "";
}
//Edit an existing task
var editTask = function() {
console.log("Edit task...");
var listItem = this.parentNode;
//Changed from input=text to type=text
var editInput = listItem.querySelector("input[type=text]");
//was listIem, instead of listItem
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
//if the class of the parent is .editMode
if(containsClass) {
//Switch from .editMode
//label text become the input's value
label.innerText = editInput.value;
} else {
//Switch to .editMode
//input value becomes the label's text
editInput.value = label.innerText;
}
//Toggle .editMode
//Spelling error
listItem.classList.toggle("editMode");
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
//When the Delete button is pressed
//Remove the parent list item from the ul
var listItem = this.parentNode;
//was listIem, needs to be listItem
var ul = listItem.parentNode;
ul.removeChild(listItem);
}
//Mark a Task as complete
var taskCompleted = function() {
console.log("task complete ...");
//When the Checkbox is checked
//Append the task list item to the completed-tasks
//listItem not defined
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
//Append the task list item to the completed-tasks
//should be listItem, not listIem
var listItem = this.parentNode;
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
var ajaxRequest = function() {
console.log("AJAX request");
}
//Set the click handler to the addTask function
addButton.addEventListener("click", addTask);
addButton.addEventListener("click", ajaxRequest);
//addButton.onclick = ajaxRequest;
//cycle over incompleteTaskHolder ul list items
for(var i = 0; i < incompleteTaskHolder.children.length; i++){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTaskHolder.children[i], taskCompleted);
}
//cycle over completedTaskHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list item's children (taskIncompleted)
//Wrong holder used
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
Erik Wells
5,403 PointsErik Wells
5,403 PointsTHANK YOU!!!! I need to keep an eye out for those spelling errors. They seem to be my worst enemy.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou're very welcome! If you use Workspaces (or most IDEs such as Sublime Text/Brackets/etc.) they will autofill in the variable you created earlier in the script. Be sure to take advantage of that! :) Happy Coding, my friend!