Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial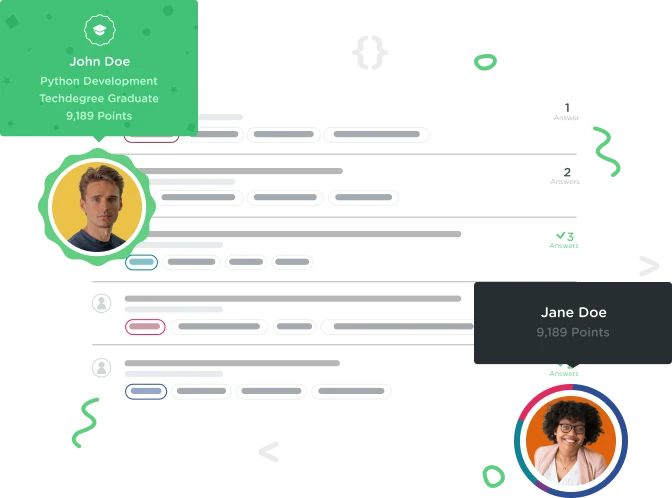

Susan Rusie
10,671 PointsCode looks right, but won't add item.
I am getting these errors and I have been over and over my code and can't find my mistake. Any help anyone can offer would be greatly appreciated.
Uncaught TypeError: li.appendChlld is not a function
at attachListItemButtons (app.js:14)
at HTMLButtonElement.addItemButton.addEventListener (app.js:72)
attachListItemButtons @ app.js:14
addItemButton.addEventListener @ app.js:72
app.js:14 Uncaught TypeError: li.appendChlld is not a function
at attachListItemButtons (app.js:14)
at HTMLButtonElement.addItemButton.addEventListener (app.js:72)
attachListItemButtons @ app.js:14
addItemButton.addEventListener @ app.js:72
Here is my code:
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const listUl = listDiv.querySelector('ul');
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
function attachListItemButtons(li) {
let up = document.createElement('button');
up.className = 'up';
up.textContent = 'Up';
li.appendChlld(up);
let down = document.createElement('button');
down.className = 'down';
down.textContent = 'Down';
li.appendChlld(down);
let remove = document.createElement('button');
remove.className = 'remove';
remove.textContent = 'Remove';
li.appendChlld(remove);
}
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi) {
ul.insertBefore(li, prevLi);
}
}
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi) {
ul.insertBefore(nextLi, li);
}
}
}
});
toggleList.addEventListener('click', () => {
if (listDiv.style.display == 'none') {
toggleList.textContent = 'Hide list';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show list';
listDiv.style.display = 'none';
}
});
descriptionButton.addEventListener('click', () => {
descriptionP.innerHTML = descriptionInput.value + ':';
descriptionInput.value = '';
});
addItemButton.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
attachListItemButtons(li);
ul.appendChild(li);
addItemInput.value = '';
});
Thanks in advance.
2 Answers

andren
28,558 PointsThere is a typo in your code, you misspelled appendChild as appendChlld (you have two l letters instead of il).
If you fix that typo that should fix those errors, though I only took a cursory glance at the code so there could be other issues present as well.

Susan Rusie
10,671 PointsThank you. I discovered it shortly after I posted this. Once I changed the typo, the program ran the way it should.
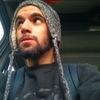
Cosimo Scarpa
14,047 PointsI have a question, but what represent this button.addItemButton in
const addItemButton = document.querySelector('button.addItemButton');
thanks!
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsI forgot to copy the first line
const toggleList = document.getElementById('toggleList');
, but it still isn't working.