Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial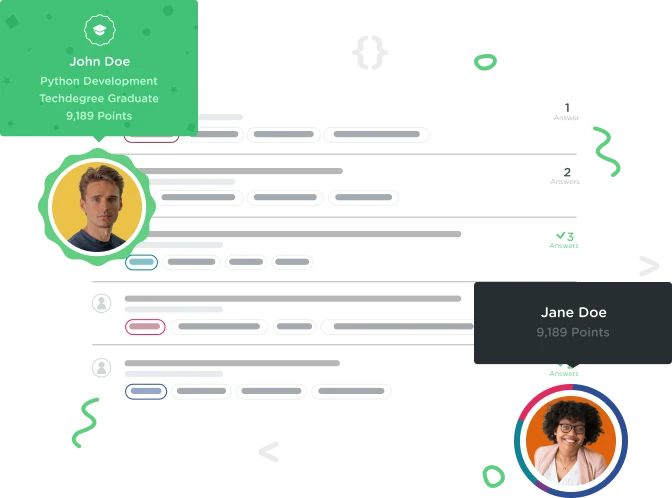

Dragan Ceriman
1,215 PointsCode not compiled even though it is correct
Even though code is compiled and running OK in xcode, does not even compile in code challenge. Therefore, can not proceed. :)
I removed ' from string "i'm a machine" since it commented out all of my code.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below
class Robot: Machine {
init(x: Int, y: Int) {
super.init()
self.location.x = x
self.location.y = y
}
override func move(direction: String) {
switch direction {
case "Up":
self.location.y += 1
case "Down":
self.location.y -= 1
case "Left":
self.location.x -= 1
case "Right":
self.location.x += 1
default:
break
}
}
}
4 Answers
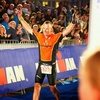
Steve Hunter
57,712 PointsHi Dragan,
The only thing here is that you don't need to rewrite the init
method. Remove that part of your code and the rest is fine.
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": location.y++
case "Down": location.y--
case "Left": location.x--
case "Right": location.x++
default: break
}
}
}
Deleting the apostrophe makes no difference, but you can escape it with a backslash else it does mess with the formatting in the code window, which is annoying!
func move(direction: String) {
print("Do nothing! I\'m a machine!")
}
I hope that helps,
Steve.
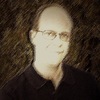
Jason Anders
Treehouse Moderator 145,860 PointsHey Dragan,
Yes, this is a glitch in the code challenge and the Support Team has been made aware.
In the meantime, you can't remove the '
, but you can escape it - add a backslash before the single quotation mark.
print("Do nothing! I\'m a machine!")
However, there is also an error in your code. You added an unnecessary init statement for the subclass of Robot. Robot is inheriting everything from its Super Class of Machine, so you don't have to re-initialize them.
Additionally, the self.location
can be just location
but either work... the latter is just less typing.
The completed code from the affected line will be:
print("Do nothing! I\'m a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up":
self.location.y += 1
case "Down":
self.location.y -= 1
case "Left":
self.location.x -= 1
case "Right":
self.location.x += 1
default:
break
}
}
}
I apologize it took this long for someone to address the problem for you.
Keep Coding! :)

Dragan Ceriman
1,215 PointsThank you for your answers. I thought that my version would be better since you can put any location and not only (0,0) :) But, nevertheless, many thanks!
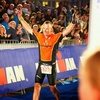
Steve Hunter
57,712 PointsHi Dragan,
I agree that the functionality that you added does make sense. However, the compiler doesn't know that, unfortunately, so it wouldn't know how to instantiate a Robot
. The behind-the-scenes tests wouldn't be generated to pass in two integers as parameters so no Robot
object would be generated. This would mean the compiler fails your code.
Make sense?
Steve.

Dragan Ceriman
1,215 PointsMake sense. Cheers!
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsGuess Steve types faster than I do... lol
Steve Hunter
57,712 PointsSteve Hunter
57,712 Points