Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial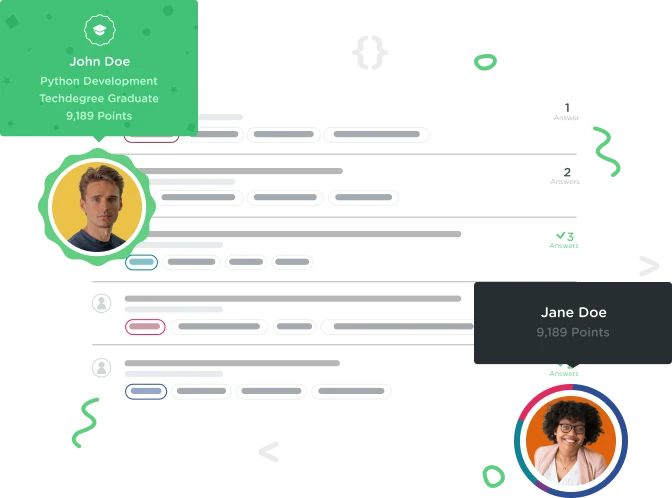
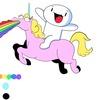
Tin David Klinac
Courses Plus Student 208 PointsCode not working after adding a couple of facts
So basically, I've added a couple of new facts to the code. 21 to be exact. I've done everything in the video but I'm getting errors.
// Declare our view variables
private TextView mFactTextView;
private Button mShowFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Asign the views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] facts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built."
"Banging your head against a wall burns 150 calories an hour."
"In the UK, it is illegal to eat mince pies on Christmas Day."
"When hippos are upset, their sweat turns red."
"A flock of crows is known as a murder."
"“Facebook Addiction Disorder” is a mental disorder identified by Psychologists."
"The average woman uses her height in lipstick every 5 years"
"29th May is officially “Put a Pillow on Your Fridge Day“."
"Cherophobia is the fear of fun."
"Human saliva has a boiling point three times that of regular water."
"If you lift a kangaroo’s tail off the ground it can’t hop."
"Hyphephilia are people who get aroused by touching fabrics."
"Billy goats urinate on their own heads to smell more attractive to females."
"The person who invented the Frisbee was cremated and made into frisbees after he died."
"During your lifetime, you will produce enough saliva to fill two swimming pools."
"Polar bears can eat as many as 86 penguins in a single sitting (if they lived in the same place)."
"King Henry VIII slept with a gigantic axe beside him."
"Bikinis and tampons invented by men."
"An eagle can kill a young deer and fly away with it."
"The world's first handheld mobile phone's cost was $3,995."
"Applesauce was the first food eaten in space by an american astronaut."
"A bat can eat 3,000 insects in one night." };
// The button was clicked so update the fact text view with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(facts.lenght);
fact = facts[randomNumber];
// Update the screen with our dinamic fact
mFactTextView.setText(fact);
}
};
mShowFactButton.setOnClickListener(listener);
}
The errors I'm getting WHEN running the app in the emulator:
Error:(37, 98) error: '}' expected
Error:(39, 25) error: not a statement
Error:(39, 87) error: ';' expected
Error:(66, 21) error: <identifier> expected
Error:(69, 38) error: <identifier> expected
Error:(69, 43) error: <identifier> expected
Error:(71, 14) error: ';' expected
Error:(73, 43) error: <identifier> expected
Error:(73, 52) error: <identifier> expected
Error:(75, 1) error: class, interface, or enum expected
Error:Execution failed for task ':app:compileDebugJavaWithJavac'.
> Compilation failed; see the compiler error output for details.
I'd love some help. Thanks!
5 Answers

Philip G
14,600 PointsFinally, this code should work:
import android.content.DialogInterface;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends AppCompatActivity {
// Declare our view variables
private TextView mFactTextView;
private Button mShowFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Asign the views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] facts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built.",
"Banging your head against a wall burns 150 calories an hour.",
"In the UK, it is illegal to eat mince pies on Christmas Day.",
"When hippos are upset, their sweat turns red.",
"A flock of crows is known as a murder.",
"“Facebook Addiction Disorder” is a mental disorder identified by Psychologists.",
"The average woman uses her height in lipstick every 5 years",
"29th May is officially “Put a Pillow on Your Fridge Day“.",
"Cherophobia is the fear of fun.",
"Human saliva has a boiling point three times that of regular water.",
"If you lift a kangaroo’s tail off the ground it can’t hop.",
"Hyphephilia are people who get aroused by touching fabrics.",
"Billy goats urinate on their own heads to smell more attractive to females.",
"The person who invented the Frisbee was cremated and made into frisbees after he died.",
"During your lifetime, you will produce enough saliva to fill two swimming pools.",
"Polar bears can eat as many as 86 penguins in a single sitting (if they lived in the same place).",
"King Henry VIII slept with a gigantic axe beside him.",
"Bikinis and tampons invented by men.",
"An eagle can kill a young deer and fly away with it.",
"The world's first handheld mobile phone's cost was $3,995.",
"Applesauce was the first food eaten in space by an american astronaut.",
"A bat can eat 3,000 insects in one night."
};
// The button was clicked so update the fact text view with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(facts.length);
fact = facts[randomNumber];
// Update the screen with our dinamic fact
mFactTextView.setText(fact);
}
};
mShowFactButton.setOnClickListener(listener);
}
}

Philip G
14,600 PointsHi Tin,
You have to comma-seperate strings in arrays. This code should work for you:
// Declare our view variables
private TextView mFactTextView;
private Button mShowFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Asign the views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] facts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built.",
"Banging your head against a wall burns 150 calories an hour.",
"In the UK, it is illegal to eat mince pies on Christmas Day.",
"When hippos are upset, their sweat turns red.",
"A flock of crows is known as a murder.",
"“Facebook Addiction Disorder” is a mental disorder identified by Psychologists.",
"The average woman uses her height in lipstick every 5 years",
"29th May is officially “Put a Pillow on Your Fridge Day“.",
"Cherophobia is the fear of fun.",
"Human saliva has a boiling point three times that of regular water.",
"If you lift a kangaroo’s tail off the ground it can’t hop.",
"Hyphephilia are people who get aroused by touching fabrics.",
"Billy goats urinate on their own heads to smell more attractive to females.",
"The person who invented the Frisbee was cremated and made into frisbees after he died.",
"During your lifetime, you will produce enough saliva to fill two swimming pools.",
"Polar bears can eat as many as 86 penguins in a single sitting (if they lived in the same place).",
"King Henry VIII slept with a gigantic axe beside him.",
"Bikinis and tampons invented by men.",
"An eagle can kill a young deer and fly away with it.",
"The world's first handheld mobile phone's cost was $3,995.",
"Applesauce was the first food eaten in space by an american astronaut.",
"A bat can eat 3,000 insects in one night."
};
// The button was clicked so update the fact text view with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(facts.lenght);
fact = facts[randomNumber];
// Update the screen with our dinamic fact
mFactTextView.setText(fact);
}
};
mShowFactButton.setOnClickListener(listener);
}
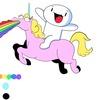
Tin David Klinac
Courses Plus Student 208 PointsHey. The thing has lowered the number of errors. I'm still getting 1 error:
Error:(75, 6) error: reached end of file while parsing
If you could help with this, that would be great.

Philip G
14,600 PointsGlad to help :)
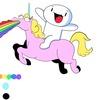
Tin David Klinac
Courses Plus Student 208 PointsAlerting you for the newest edit!

Philip G
14,600 PointsSo, is there a problem again? If so, please post this as a answer to this thread
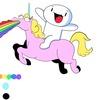
Tin David Klinac
Courses Plus Student 208 PointsYeah, basically, I'm getting this error when trying to run the app:
Error:(75, 6) error: reached end of file while parsing
It's pretty strange as I copied the whole thing in correctly and all of my other code is good.

Philip G
14,600 PointsThat is strange... Please post the whole code of your activity
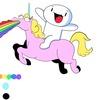
Tin David Klinac
Courses Plus Student 208 PointsDamn it. I wish someone had a project download of this.

Philip G
14,600 PointsWell, I can upload the project for you somewhere
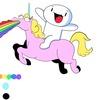
Tin David Klinac
Courses Plus Student 208 PointsThat'd be awesome.
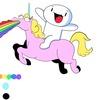
Tin David Klinac
Courses Plus Student 208 Pointsimport android.content.DialogInterface;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class FunFactsActivity extends AppCompatActivity {
// Declare our view variables
private TextView mFactTextView;
private Button mShowFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Asign the views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] facts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built.",
"Banging your head against a wall burns 150 calories an hour.",
"In the UK, it is illegal to eat mince pies on Christmas Day.",
"When hippos are upset, their sweat turns red.",
"A flock of crows is known as a murder.",
"“Facebook Addiction Disorder” is a mental disorder identified by Psychologists.",
"The average woman uses her height in lipstick every 5 years",
"29th May is officially “Put a Pillow on Your Fridge Day“.",
"Cherophobia is the fear of fun.",
"Human saliva has a boiling point three times that of regular water.",
"If you lift a kangaroo’s tail off the ground it can’t hop.",
"Hyphephilia are people who get aroused by touching fabrics.",
"Billy goats urinate on their own heads to smell more attractive to females.",
"The person who invented the Frisbee was cremated and made into frisbees after he died.",
"During your lifetime, you will produce enough saliva to fill two swimming pools.",
"Polar bears can eat as many as 86 penguins in a single sitting (if they lived in the same place).",
"King Henry VIII slept with a gigantic axe beside him.",
"Bikinis and tampons invented by men.",
"An eagle can kill a young deer and fly away with it.",
"The world's first handheld mobile phone's cost was $3,995.",
"Applesauce was the first food eaten in space by an american astronaut.",
"A bat can eat 3,000 insects in one night."
};
// The button was clicked so update the fact text view with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(facts.lenght);
fact = facts[randomNumber];
// Update the screen with our dinamic fact
mFactTextView.setText(fact);
}
};
mShowFactButton.setOnClickListener(listener);
}

Philip G
14,600 PointsYou are missing a closing bracket at the end! This is the edited code:
import android.content.DialogInterface;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class FunFactsActivity extends AppCompatActivity {
// Declare our view variables
private TextView mFactTextView;
private Button mShowFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Asign the views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] facts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built.",
"Banging your head against a wall burns 150 calories an hour.",
"In the UK, it is illegal to eat mince pies on Christmas Day.",
"When hippos are upset, their sweat turns red.",
"A flock of crows is known as a murder.",
"“Facebook Addiction Disorder” is a mental disorder identified by Psychologists.",
"The average woman uses her height in lipstick every 5 years",
"29th May is officially “Put a Pillow on Your Fridge Day“.",
"Cherophobia is the fear of fun.",
"Human saliva has a boiling point three times that of regular water.",
"If you lift a kangaroo’s tail off the ground it can’t hop.",
"Hyphephilia are people who get aroused by touching fabrics.",
"Billy goats urinate on their own heads to smell more attractive to females.",
"The person who invented the Frisbee was cremated and made into frisbees after he died.",
"During your lifetime, you will produce enough saliva to fill two swimming pools.",
"Polar bears can eat as many as 86 penguins in a single sitting (if they lived in the same place).",
"King Henry VIII slept with a gigantic axe beside him.",
"Bikinis and tampons invented by men.",
"An eagle can kill a young deer and fly away with it.",
"The world's first handheld mobile phone's cost was $3,995.",
"Applesauce was the first food eaten in space by an american astronaut.",
"A bat can eat 3,000 insects in one night."
};
// The button was clicked so update the fact text view with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(facts.lenght);
fact = facts[randomNumber];
// Update the screen with our dinamic fact
mFactTextView.setText(fact);
}
};
mShowFactButton.setOnClickListener(listener);
}
}
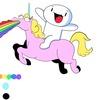
Tin David Klinac
Courses Plus Student 208 PointsOh gosh. After doing that, I'm getting even MORE errors.
Error:(65, 17) error: cannot find symbol class Random
Error:(65, 46) error: cannot find symbol class Random
Error:(66, 65) error: cannot find symbol variable lenght

Philip G
14,600 PointsOf course you have to import the Random class, your IDE should have done that automatically.
Add import java.util.Random;
`at the top of your code.
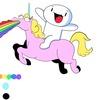
Tin David Klinac
Courses Plus Student 208 PointsOh gosh. I'm getting only 1 error after this.
Error:(67, 65) error: cannot find symbol variable lenght
Thanks a shit ton for actually taking care of this problem for me for the last 30 minutes.

Philip G
14,600 PointsNo problem! length in Java is not an attribute, its a method. In your case you have to the random number initialization line to facts.lenght()
Ignore that answer, this is wrong
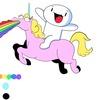
Tin David Klinac
Courses Plus Student 208 PointsCould you just paste the code? I'm too clumbsy to actually put it in myself as I'm just getting the same error. Thanks one more time.
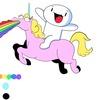
Tin David Klinac
Courses Plus Student 208 PointsGetting an error again. I must be a pain in the ass with this haha.
Error:(67, 65) error: cannot find symbol method lenght()

Philip G
14,600 PointsSorry - It was my mistake (Mixing it up with C# a little bit at the moment) Length is of course a property, you just misspelled it. Updated my answer
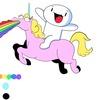
Tin David Klinac
Courses Plus Student 208 PointsAlright. It's now working and shows no errors. The only problem is when ran on the emulator, the app doesn't run through the facts randomly but instead shows only the first 2 facts.

Philip G
14,600 PointsThere can be several problems causing this issue:
- The random is time based and you just have bad luck
- The facts haven't properly been replaced in the interface
- Some other code is affecting the facts
- There is an issue with the emulator
- ...
You can try running the app on a real android device.
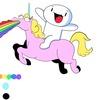
Tin David Klinac
Courses Plus Student 208 PointsNumber 1 and 4 don't apply here as I'm 100% sure that isn't the problem. Now, how would I fix it if potentially the facts haven't properly been replaced in the interface? And I'm pretty sure that nothing is affecting the code.

Philip G
14,600 PointsJust set a breakpoint to see what number the random is and go into debugging mode
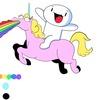
Tin David Klinac
Courses Plus Student 208 Pointsaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaand how would I do that? Haha, sorry. Pretty new to this.

Philip G
14,600 PointsNo problem, you can find a guide over here

Philip G
14,600 PointsYou can find the project here Though I would recommend you to still follow up with the videos and look only on it when you get stuck. Otherwise you won't learn much :)
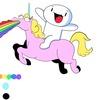
Tin David Klinac
Courses Plus Student 208 PointsDo you possibly have the APK file? When trying to save mine it's not possible as there's this error with syncing.
Error:Unable to save 'C:\Program Files\Android\Android Studio\FunFacts\local.properties'C:\Program Files\Android\Android Studio\FunFacts\local.properties (Access is denied)

Philip G
14,600 PointsI've uploaded it. Maybe you have to adjust the sdk path of the project. If this doesnt't work for you, you can copy the relevant files over to your project manually
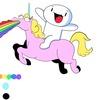
Tin David Klinac
Courses Plus Student 208 PointsYou've accidentally uploaded the debuggable APK. :D

Philip G
14,600 PointsNo, I don't think so. It's just the filename Android Studio gave my file :) Try if this one works
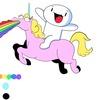
Tin David Klinac
Courses Plus Student 208 PointsYeah. Still the debuggable version.

Philip G
14,600 PointsUploaded the release version
Philip G
14,600 PointsPhilip G
14,600 PointsAdded code formatting