Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial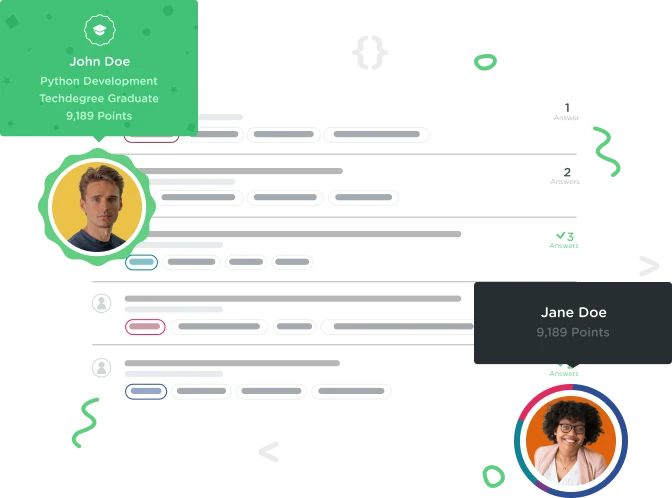

Stephen Dixon
933 PointsCode not working at all!
Okay, I've gone over this code with a toothcomb and I still can't see the error. None of the functions seem to be working proper, If some body can please look this over so I can get this code up and running, I'd be very grateful. I realize this is asking a lot! Anyway, here goes:
var taskInput = document.getElementById("new-task");
var incompleteTasksHolder = document.getElementById("incomplete-tasks");
var completeTasksHolder = document.getElementById("completed-tasks");
var addButton = document.getElementsByTagName("button")[0];
var createNewTaskElement = function(taskString) {
var listItem = document.createElement("li");
var checkBox = document.createElement("input");
var label = document.createElement("label");
var editInput = document.createElement("input");
var editButton = document.createElement("button");
var deleteButton = document.createElement("button");
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
deleteButton.innerText = "Delete";
editButton.className = "edit";
deleteButton.className = "delete";
label.innerText = taskString;
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
var addTask = function() {
console.log("Add task");
var listItem = createNewTaskElement(taskInput.value);
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = "";
}
var editTask = function() {
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
if(containsClass) {
label.innerText = editInput.value;
}
else
editInput.value = label.innerText;
}
listItem.classList.toggle("editMode");
var deleteTask = function() {
var listItem = this.parentNode;
var ul = listItem.parentNode;
ul.removeChild(listItem);
}
var taskCompleted = function() {
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncompleted);
}
var taskIncompleted = function() {
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("bind list items");
var checkBox = taskListItem.querySelector("input[type="checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
editButton.onclick = editTask;
deleteButton.onclick = deleteTask;
checkBox.onchange = checkBoxEventHandler;
}
addButton.onclick = addTask;
for(var index = 0; index < incompleteTasksHolder.children.length; index++) {
bindTaskEvents(incompleteTasksHolder.children[index], taskCompleted);
}
for(var index = 0; index < completeTasksHolder.children.length; index++) {
bindTaskEvents(completeTasksHolder.children[index], taskIncompleted);
}
3 Answers
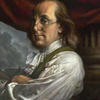
Jeff Busch
19,287 PointsHi Stephen,
I found a few typos. Your code has the two greater than signs ">>".
Jeff
>>var completeTasksHolder = document.getElementById("completed-tasks");
var completedTasksHolder= document.getElementById("completed-tasks");
>>var checkBox = taskListItem.querySelector("input[type="checkbox]");
var checkBox = taskListItem.querySelector("input[type=checkbox]");
>>for(var index = 0; index < completeTasksHolder.children.length; index++) {
bindTaskEvents(completeTasksHolder.children[index], taskIncompleted);
}
for(var i = 0; i < completedTasksHolder.children.length; i++) {
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}

Christopher Hall
9,052 PointsI see an error on this line:
var checkBox = taskListItem.querySelector("input[type="checkbox]");
You can't use double quotes within double quotes. Also you're missing an end quote after ="checkbox". Either use single quotes inside, or escape your double quotes with a backslash:
var checkBox = taskListItem.querySelector("input[type='checkbox']");
var checkBox = taskListItem.querySelector("input[type=\"checkbox\"]");
One more thing, you're missing a closing bracket after "text" on this line:
var editInput = listItem.querySelector("input[type=text");
... and this code is probably causing problems as well:
var editTask = function() {
if(containsClass) {
label.innerText = editInput.value;
}
else
editInput.value = label.innerText;
}
If you're going to use curly brackets for the if statement, also use them for the else. While the above is technically legal javascript, it could cause some problems for the parser. It works fine ONLY if you are strict and add a semicolon after the final curly bracket, since it then recognizes this as the end of a function variable definition and not an error in the "else" statement.

Stephen Dixon
933 PointsJeff, I wish I would have read your answer more thoroughly. I had some serious issues even before I submitted this with variable names, specifically taskCompleted & taskIncomplete which have to match exactly, obviously!