Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial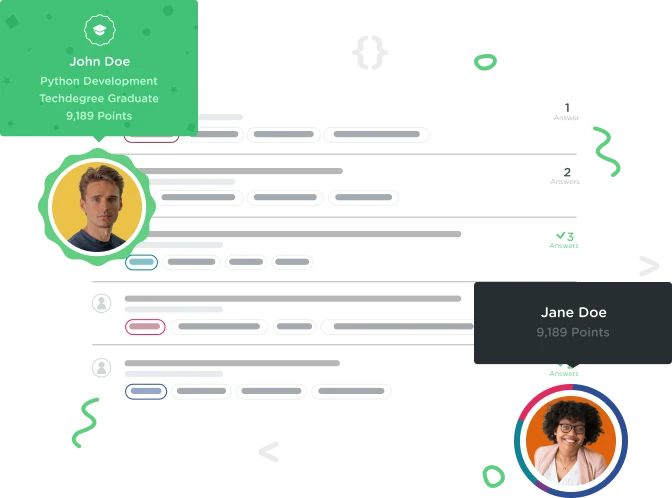

Kylie Soderblom
3,890 PointsCode not working. Node returns no errors, but doesn't print out any console logs written within the function get(query)
I've added additional console logs to check if ex: stringify is working correctly Only my console.logs outside of the "function get (query)" are working. Something is wrong in the function and after numerous comparisons - I can't see it.
const http = require("http"); // statuscodes
const https = require('https');
const querystring = require("querystring");
const api = require('./api.json');
// Print out temp details
function printWeather(weather){
const message = `Current temperature in ${weather.name} is ${weather.main.temp}F and is ${weather.description}`;
console.log(message);
}
// Print out error message
function printError(error){
console.error(error.message);
}
console.log("check point 1");
function get(query) {
try{
const parameters = {
APPID: api.key,
units: 'imperial'
};
const zipCode = parseInt(query);
if (!isNaN(zipCode)) {
parameters.zip = zipCode + ',us';
} else {
parameters.q = query + ',us';
}
// check if stringify works
const string = querystring.stringify(parameters);
console.log(string);
const url = `https://api.openweathermap.org/data/2.5/weather?${string}`;
console.log(url);
console.log("checkpoint 2, you should've seen parameters in a string AND the url printed out");
const request = https.get(url, response => {
if(response.statusCode === 200){
let body = "";
// Read the data
response.on('data', chunk => {
body += chunk;
});
response.on('end', () => {
try{
//Parse data
const weather = JSON.parse(body);
//Print the data
printWeather(weather);
}catch (error) {
//parser error
printError(error);
}
});
} else {
//status error code
const statusErrorCode = new Error(`There was an error getting the message for #${query}. (${http.STATUS_CODES[response.statusCode]})`);
printError(statusErrorCode);
}
});
}catch (error) {
printError(error);
}
}
console.log("goodbye");
module.exports.get = get;
treehouse:~/workspace$ node weather.js 91361
check point 1
goodbye
2 Answers

Blake Larson
13,014 PointsDid you ever figure this out?
It looks like you are exporting the get
function to be called in a different script with a query being sent.
node weather.js 91361
runs the weather.js script which I'm guessing parses the arguments to grab the zip code.
Is this weather.js? I ask because I don't see the get
function getting called anywhere so if you run that code with the function brackets commented out then the same code would run automatically.

Kylie Soderblom
3,890 PointsBlake Larson Thank you for checking in with me. I honestly just steamed forward. I need to revisit this and will do so in the morning. Then I'll be able to report. Hopefully, I'll get better results in the morning.
Kylie Soderblom
3,890 PointsKylie Soderblom
3,890 PointsTaking apart the code further, it appears that the issue pops up when I try to add function get(query) and module.exports.get = get. Prior to that, the code prints out: Current temperature in Barstow is 107.13F and clear sky.