Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial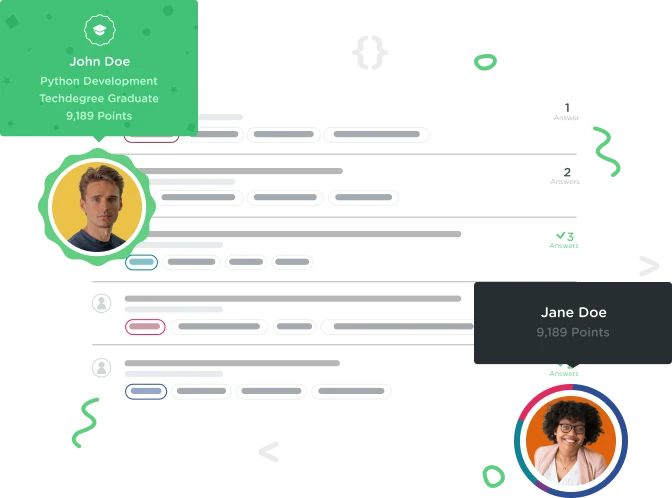

Leonardo Escalante
3,190 PointsCode question.
I don't understand well the purpose of this two lines of code:
class TodoList
attr_reader :name, :todo_items
Please help me with that, thanks.
3 Answers

michael rode
240 PointsYou will come across attr_reader, attr_writer and attr_accessor while working with Ruby. The first line is creating a class called Todoitems. I have recreated an example of that class
class Todolist
attr_reader:name, :todo_item
def initialize(name, todo_item)
@name = name
@todo_item = todo_item
end
end
list = Todolist.new("Mike", "Shoe")
puts list.name
puts list.todo_item
You can see that I am able to call list.name and list.todo_item. This will return "mike" and "shoe" the reason I have access to these variables is because of that method. A really helpful way to break it down is to jump behind the scenes and take a look at what that method is actually doing.
class Todolist
def initialize(name, todo_item)
@name = name
@todo_item = todo_item
end
def name
@name
end
def todo_item
@todo_item
end
end
list = Todolist.new("Mike", "Shoe")
puts list.name
puts list.todo_item
This code is essentially the same as above. But instead of having to define a todo_item method and a name method we can just uset the attr_reader method instead.

Patrick Mooney
34,161 PointsIt adds getter methods for the name and todo_items member variables.
Without that line, you'd need to access them with a preceding "@" from within your class, and you wouldn't be able to access them from outside of your class, unless you added your own getter method.

Leonardo Escalante
3,190 PointsGreat explanation, thanks!